Maximizing Code Reusability with Kleisli Composition
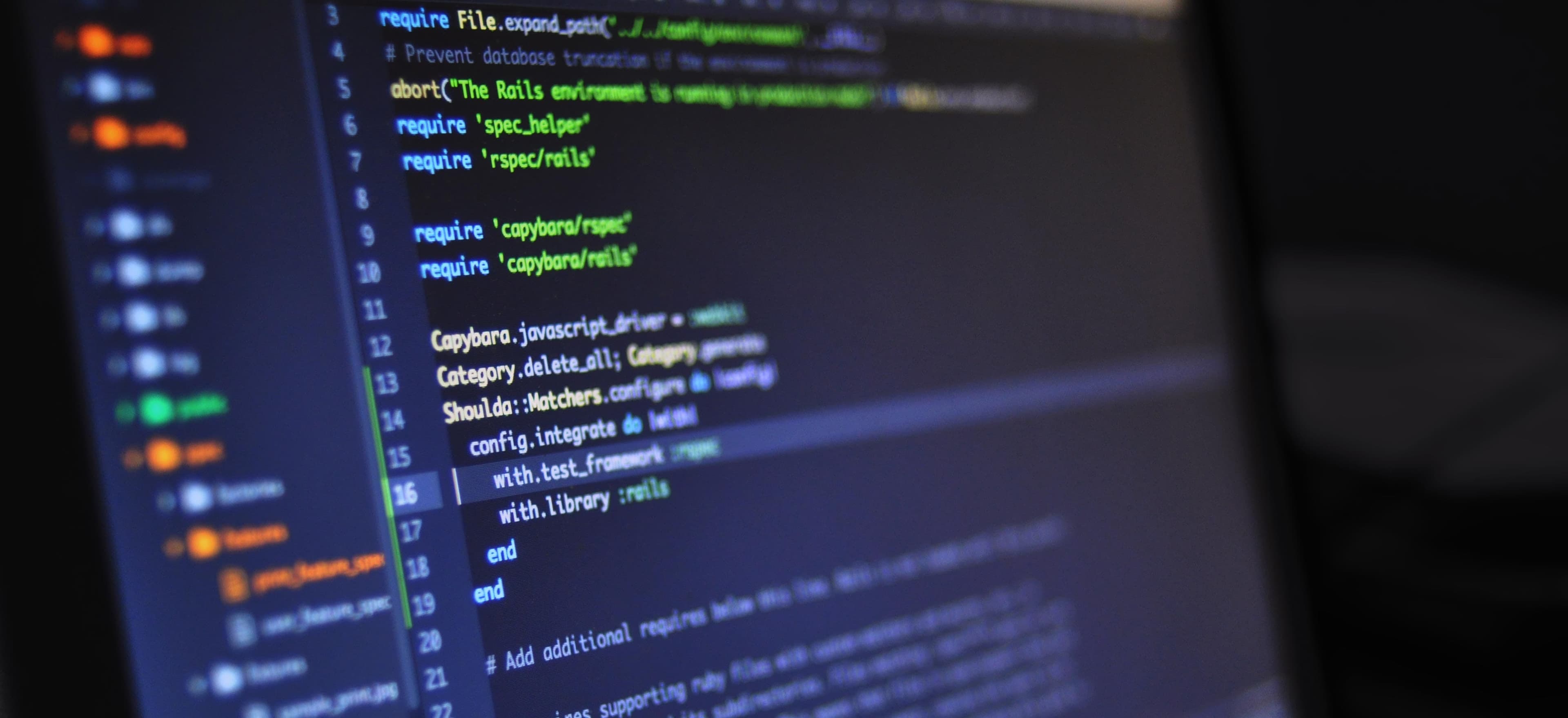
- Published on
Maximizing Code Reusability with Kleisli Composition
In Java programming, code reusability is a crucial principle that allows developers to write and maintain efficient, clean, and scalable code. One powerful technique for achieving code reusability is through functional composition, specifically using Kleisli composition.
This article will delve into the concept of Kleisli composition, explain its significance in achieving code reusability, and provide practical examples of how it can be implemented in Java.
Understanding Kleisli Composition
Kleisli composition is a functional programming concept that enables the composition of functions that return a monadic value. In simpler terms, it allows developers to combine functions that operate on values wrapped in a context (such as Optional, CompletableFuture, or any other monad).
The Kleisli composition has the signature (A) -> M<B>
, where A
is the input type, B
is the output type, and M
is the monadic context. It essentially allows for the chaining of monadic operations in a concise and expressive manner.
Significance in Achieving Code Reusability
One of the key benefits of Kleisli composition is its ability to promote code reusability. By composing functions that operate on monadic values, developers can create modular and reusable components that can be combined to form larger, more complex operations. This not only results in more concise and readable code but also reduces the duplication of logic, leading to easier maintenance and debugging.
Practical Example: Using Optional for Kleisli Composition
Let's consider a practical example of using Optional for Kleisli composition. Suppose we have three functions, each of which returns an Optional result:
Optional<String> retrieveDataA() {
// retrieve and return data
}
Optional<String> processA(String data) {
// process the data and return a result
}
Optional<Integer> analyze(String processedData) {
// analyze the processed data and return an integer result
}
Instead of nesting the calls to these functions and handling the Optional values at each step, we can use Kleisli composition to create a composed function that encapsulates the entire operation:
Function<String, Optional<String>> retrieveAndProcess =
retrieveDataA.andThen(opt -> opt.flatMap(this::processA));
Function<String, Optional<Integer>> retrieveProcessAndAnalyze =
retrieveAndProcess.andThen(opt -> opt.flatMap(this::analyze));
In this example, retrieveAndProcess
and retrieveProcessAndAnalyze
represent composed functions that encapsulate the entire retrieval, processing, and analysis process.
By using Kleisli composition, we have effectively created reusable, composable functions that can be easily integrated into different parts of our codebase, promoting code reusability and maintainability.
Why Use Kleisli Composition in Java?
- Kleisli composition provides a clean and concise way to compose functions that operate on monadic values, such as Optional, CompletableFuture, and others.
- It promotes modular and reusable code, reducing duplication and improving maintainability.
- The composition of monadic operations can lead to more expressive and readable code, as it abstracts away the handling of monadic values at each step.
Implementing Kleisli Composition in Java
In Java, the Function
interface can be leveraged to implement Kleisli composition. The andThen
and compose
methods of the Function
interface allow for the composition of functions, making it a suitable candidate for implementing Kleisli composition.
Here's an example of implementing Kleisli composition using the Function
interface in Java:
import java.util.Optional;
import java.util.function.Function;
public class KleisliCompositionExample {
public static void main(String[] args) {
Function<String, Optional<String>> retrieveDataA = data -> Optional.of("Retrieved: " + data);
Function<String, Optional<String>> processA = data -> Optional.of("Processed: " + data);
Function<String, Optional<Integer>> analyze = data -> Optional.of(data.length());
Function<String, Optional<String>> retrieveAndProcess =
retrieveDataA.andThen(opt -> opt.flatMap(processA));
Function<String, Optional<Integer>> retrieveProcessAndAnalyze =
retrieveAndProcess.andThen(opt -> opt.flatMap(analyze));
Optional<Integer> result = retrieveProcessAndAnalyze.apply("input");
System.out.println(result.orElse(-1));
}
}
In this example, we define functions retrieveDataA
, processA
, and analyze
, and then use Kleisli composition to create retrieveAndProcess
and retrieveProcessAndAnalyze
. Finally, we apply retrieveProcessAndAnalyze
to an input and retrieve the result.
Using Libraries for Kleisli Composition
While Java's standard library provides the necessary tools for implementing Kleisli composition, there are also libraries such as Vavr and FunctionalJava that offer additional utilities for functional programming, including support for Kleisli composition and other functional constructs.
These libraries provide a rich set of functional abstractions that simplify the implementation of functional composition, making it easier to write expressive and composable code in Java.
To Wrap Things Up
Kleisli composition is a powerful technique that promotes code reusability and modularity by enabling the composition of functions that operate on monadic values. In Java, the Function
interface provides the foundation for implementing Kleisli composition, allowing developers to create modular, reusable, and expressive code.
By leveraging Kleisli composition, developers can maximize code reusability, reduce duplication, and create more maintainable and readable codebases. Understanding and applying Kleisli composition can significantly enhance the functional programming capabilities of Java applications, making them more robust and scalable.
In conclusion, Kleisli composition is a valuable tool in a Java developer's functional programming arsenal, and mastering its use can greatly elevate the quality and maintainability of their code.
For further exploration on functional programming in Java and the concept of Kleisli composition, consider referring to the following resources:
- Vavr Documentation
- FunctionalJava GitHub Repository
Start implementing Kleisli composition in your Java applications to experience the benefits of code reusability and expressive functional programming firsthand. Happy coding!