Handling Date and Time in Java 8
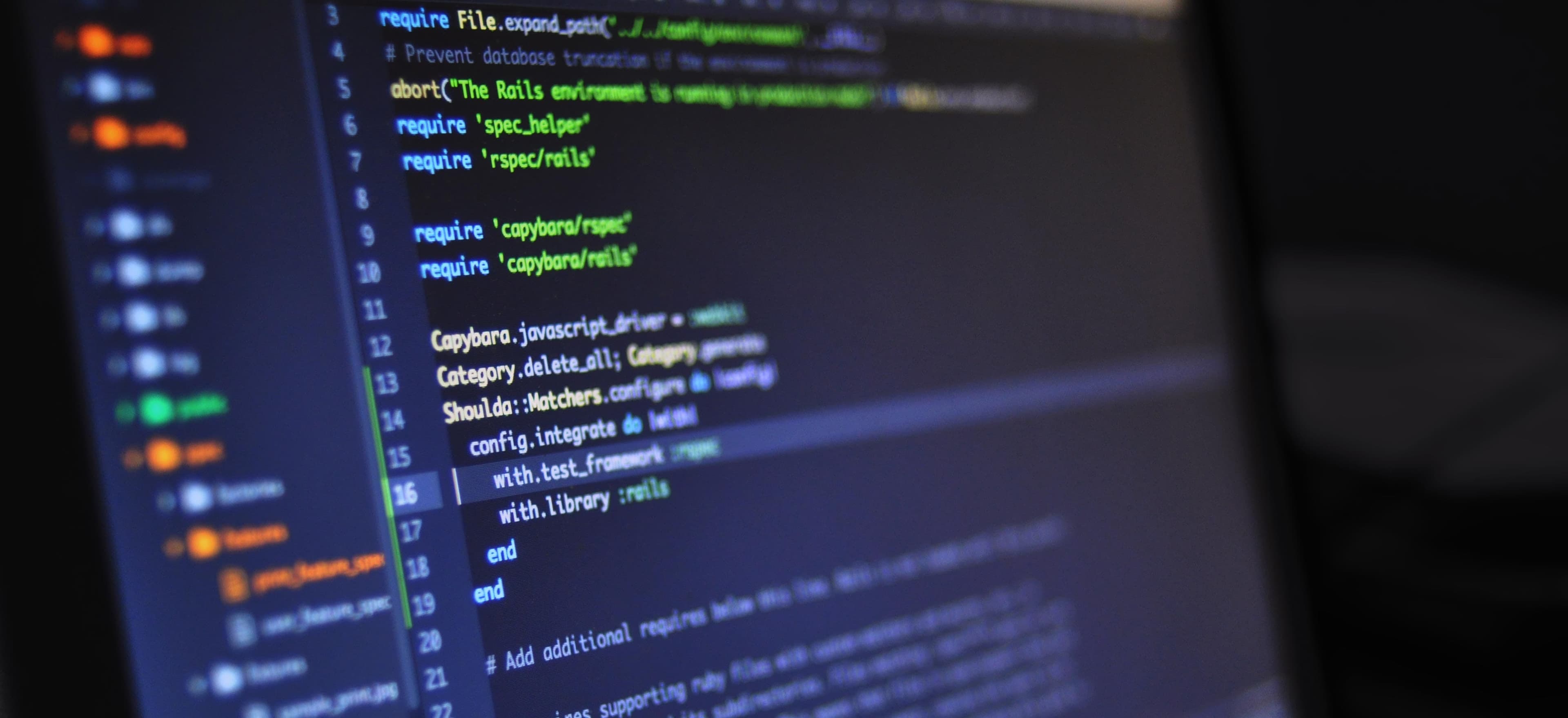
- Published on
Mastering Date and Time Handling in Java 8
When it comes to handling date and time in Java, there has been a significant improvement with the introduction of the Date and Time API in Java 8. This new API, defined in the java.time
package, provides a more comprehensive approach to working with dates and times compared to the previous java.util.Date
and java.util.Calendar
classes. In this article, we will delve into the various features of the Java 8 Date and Time API, including date and time representation, manipulation, formatting, and parsing.
The Old Way vs. The Java 8 Way
Before Java 8, working with dates and times in Java was often cumbersome. The java.util.Date
class was fraught with issues, including mutability and poor design choices, while java.util.Calendar
wasn't much better. This led to the infamous challenges in handling date and time, which were error-prone and hard to use effectively.
Java 8 addressed these issues by introducing a new Date and Time API, based on the ISO calendar system, making it easier to work with dates and times in a more intuitive and less error-prone manner.
The Core Classes of Java 8 Date and Time API
The java.time package introduced several core classes, but the most important ones to be familiar with are:
LocalDate
: Represents a date without a time component.LocalTime
: Represents a time without a date component.LocalDateTime
: Combines date and time, but without a time-zone, ideal for representing events or transactions when the time zone is irrelevant.ZonedDateTime
: Represents a date and time with a time-zone.Instant
: Represents a specific point in time on the time-line.Duration
andPeriod
: Represents a time-based amount, such as "2 hours and 30 minutes."
Basic Date and Time Operations
Let's start by exploring some basic operations for handling dates and times in Java 8.
Creating Date and Time objects
Creating date and time objects is straightforward:
LocalDate currentDate = LocalDate.now();
LocalTime currentTime = LocalTime.now();
LocalDateTime currentDateTime = LocalDateTime.now();
ZonedDateTime currentZonedDateTime = ZonedDateTime.now();
Manipulating Dates and Times
Manipulating dates and times becomes simple using the fluent API of the Java 8 Date and Time API:
LocalDate tomorrow = LocalDate.now().plusDays(1);
LocalDateTime nextHour = LocalDateTime.now().plusHours(1);
Formatting and Parsing
The Date and Time API provides a built-in way to format and parse dates as strings:
LocalDateTime dateTime = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = dateTime.format(formatter);
LocalDateTime parsedDateTime = LocalDateTime.parse(formattedDateTime, formatter);
Dealing with Timezones
Working with timezones is often a crucial aspect of handling dates and times. In Java 8, the ZoneId
class represents a time-zone and can be used along with ZonedDateTime
to manage time-zones explicitly.
ZoneId zone = ZoneId.of("Europe/Paris");
ZonedDateTime parisDateTime = ZonedDateTime.now(zone);
Converting between Legacy Date/Time and Java 8 Date/Time
It's common to encounter legacy code still using the old java.util.Date and java.util.Calendar classes. Java 8 provides utility methods to convert between these and the new Date and Time API.
From java.util.Date
to LocalDate
or LocalDateTime
Date legacyDate = new Date();
LocalDate localDate = legacyDate.toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
LocalDateTime localDateTime = legacyDate.toInstant().atZone(ZoneId.systemDefault()).toLocalDateTime();
From LocalDate
or LocalDateTime
to java.util.Date
LocalDate localDate = LocalDate.now();
Date legacyDate = Date.from(localDate.atStartOfDay(ZoneId.systemDefault()).toInstant());
The Java 8 Date and Time API simplifies these conversions, making your code more readable and less error-prone.
Final Considerations
The Java 8 Date and Time API provides a robust and comprehensive way to handle dates and times in Java applications. Its introduction has addressed the long-standing issues with the old Date and Time classes and has made working with date and time a much more pleasant experience. Understanding and mastering the Java 8 Date and Time API is crucial for any Java developer to write efficient and maintainable code when dealing with dates and times.
In conclusion, embracing the Java 8 Date and Time API will greatly benefit your Java projects, making date and time operations more intuitive and less error-prone.
Incorporating Java 8's Date and Time API into your applications can greatly improve the management of date and time-related operations. Embracing these modern practices contributes to the overall robustness and efficiency of your Java applications.