Avoid These Common Core Java Interview Mistakes
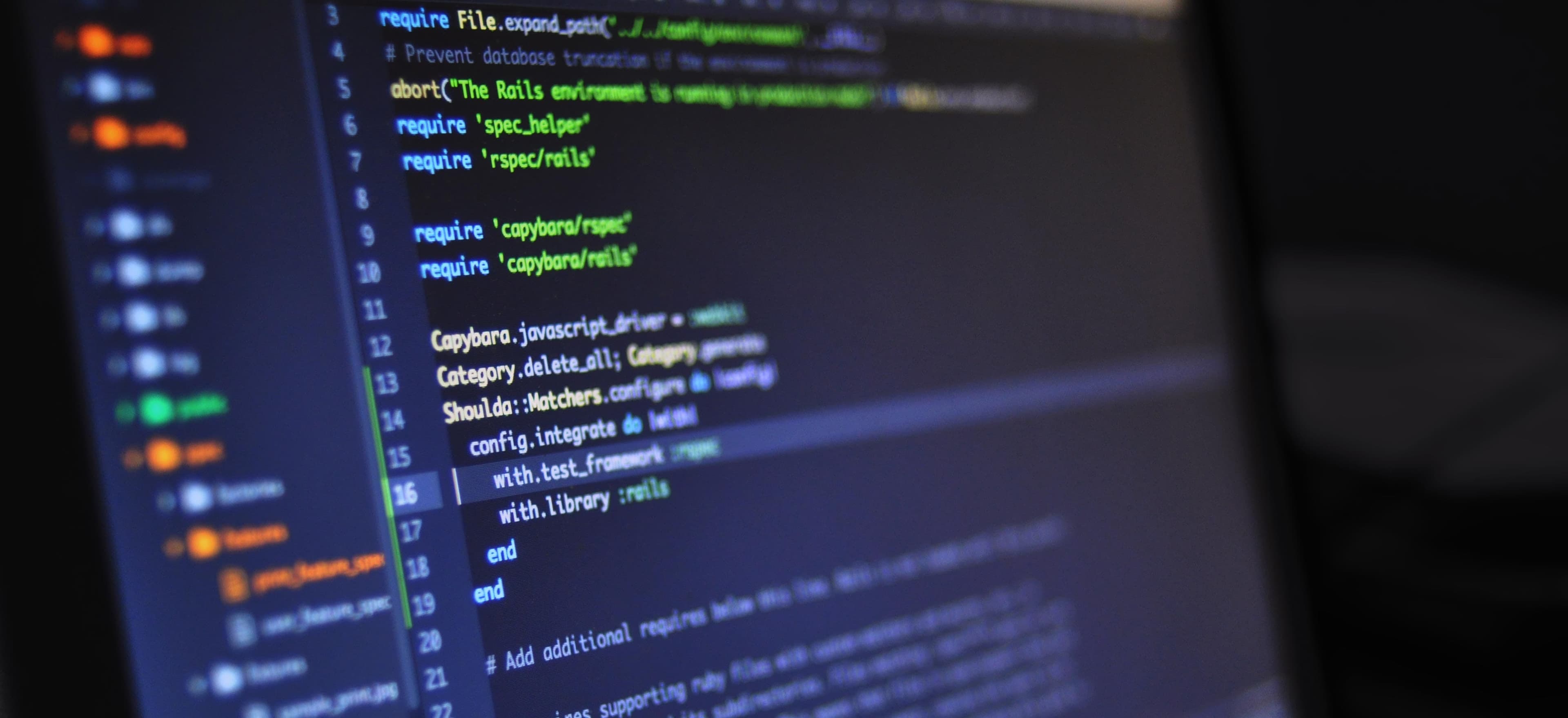
- Published on
Key Mistakes to Avoid in Core Java Interviews
Are you preparing for a core Java interview? Whether you're a fresh graduate or an experienced developer, it's essential to be well-prepared for common questions and concepts. However, there are some common mistakes that candidates make during core Java interviews that can hinder their chances of landing the job. In this post, we'll discuss some of the key mistakes to avoid in core Java interviews and provide insights on how to steer clear of them.
Mistake 1: Lack of Understanding of Basic Concepts
One of the most prevalent mistakes in core Java interviews is the lack of a solid understanding of basic concepts. It's crucial for candidates to have a strong grasp of fundamental concepts such as inheritance, polymorphism, encapsulation, and abstraction. Without a clear understanding of these concepts, candidates may struggle to answer even the most straightforward questions.
Example of basic concept:
// Example of inheritance in Java
class Parent {
void display() {
System.out.println("Parent class method");
}
}
class Child extends Parent {
void show() {
System.out.println("Child class method");
}
}
In the above example, the Child
class inherits the display
method from the Parent
class, demonstrating the concept of inheritance in Java.
Mistake 2: Overlooking Exception Handling
Exception handling is a critical aspect of Java programming, and candidates often make the mistake of overlooking its importance during interviews. Understanding how to properly use try-catch blocks, throw and throws keywords, and handling checked and unchecked exceptions is essential. Failing to demonstrate proficiency in exception handling can raise red flags for interviewers.
Example of exception handling:
// Example of try-catch block for handling exceptions
try {
int result = num1 / num2;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
}
In this example, the try
block attempts to divide num1
by num2
, and if an ArithmeticException
occurs, it is caught and handled in the catch
block.
Mistake 3: Inadequate Knowledge of Collections Framework
Another common mistake is a lack of knowledge about the Java Collections framework. Candidates should be well-versed in various collection classes such as ArrayList, LinkedList, HashMap, and understand their differences and use cases. Additionally, knowledge of key interfaces like List, Set, and Map is crucial for demonstrating competence in working with collections.
Example of using a Collection in Java:
// Example of using ArrayList to store and retrieve elements
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
System.out.println("Names: " + names);
Here, an ArrayList is used to store a list of names and then retrieve and display them.
Mistake 4: Lack of Multithreading Understanding
Multithreading is a powerful feature of Java, and candidates often falter when discussing its concepts and implementation. Understanding the basics of threads, synchronization, and concurrent programming is essential for demonstrating proficiency in multithreading. Without a solid understanding of these concepts, candidates may struggle to showcase their ability to develop efficient, concurrent Java applications.
Example of multithreading in Java:
// Example of creating a simple multithreaded program
class MyThread extends Thread {
public void run() {
System.out.println("MyThread running");
}
}
public class Main {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyThread thread2 = new MyThread();
thread1.start();
thread2.start();
}
}
In this example, two instances of MyThread
are created and started, showcasing a basic multithreaded program in Java.
Mistake 5: Neglecting OOP Principles
Object-oriented programming (OOP) is at the core of Java development, and candidates often neglect to showcase their understanding of OOP principles such as encapsulation, inheritance, polymorphism, and abstraction. Failing to demonstrate how these principles are applied in code can indicate a lack of fundamental understanding of Java programming.
Example illustrating OOP principles:
// Example demonstrating encapsulation in Java
class Student {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, the name
attribute is encapsulated within the Student
class, and access is provided through getter and setter methods, demonstrating encapsulation.
Mistake 6: Forgetting to Discuss Java Memory Management
Memory management is a critical aspect of Java, and candidates frequently overlook the importance of discussing topics such as garbage collection, memory allocation, and the significance of the finalize()
method. Understanding how Java manages memory and the impact of inefficient memory usage is crucial for showcasing a holistic understanding of Java development.
Additional Resources
For a deeper understanding of Java memory management, refer to the official Oracle documentation on Java Platform, Standard Edition (Java SE).
Closing Remarks
In conclusion, avoiding these common mistakes in core Java interviews can significantly improve your chances of impressing the interviewers. By solidifying your grasp of basic concepts, exception handling, collections framework, multithreading, OOP principles, and memory management, you can showcase your proficiency in core Java development and stand out as a strong candidate. Remember to not only understand these concepts but also be able to apply them in practical scenarios, as interviewers often emphasize real-world problem-solving abilities. Good luck with your core Java interview preparation!