Preventing Java Program Vulnerabilities
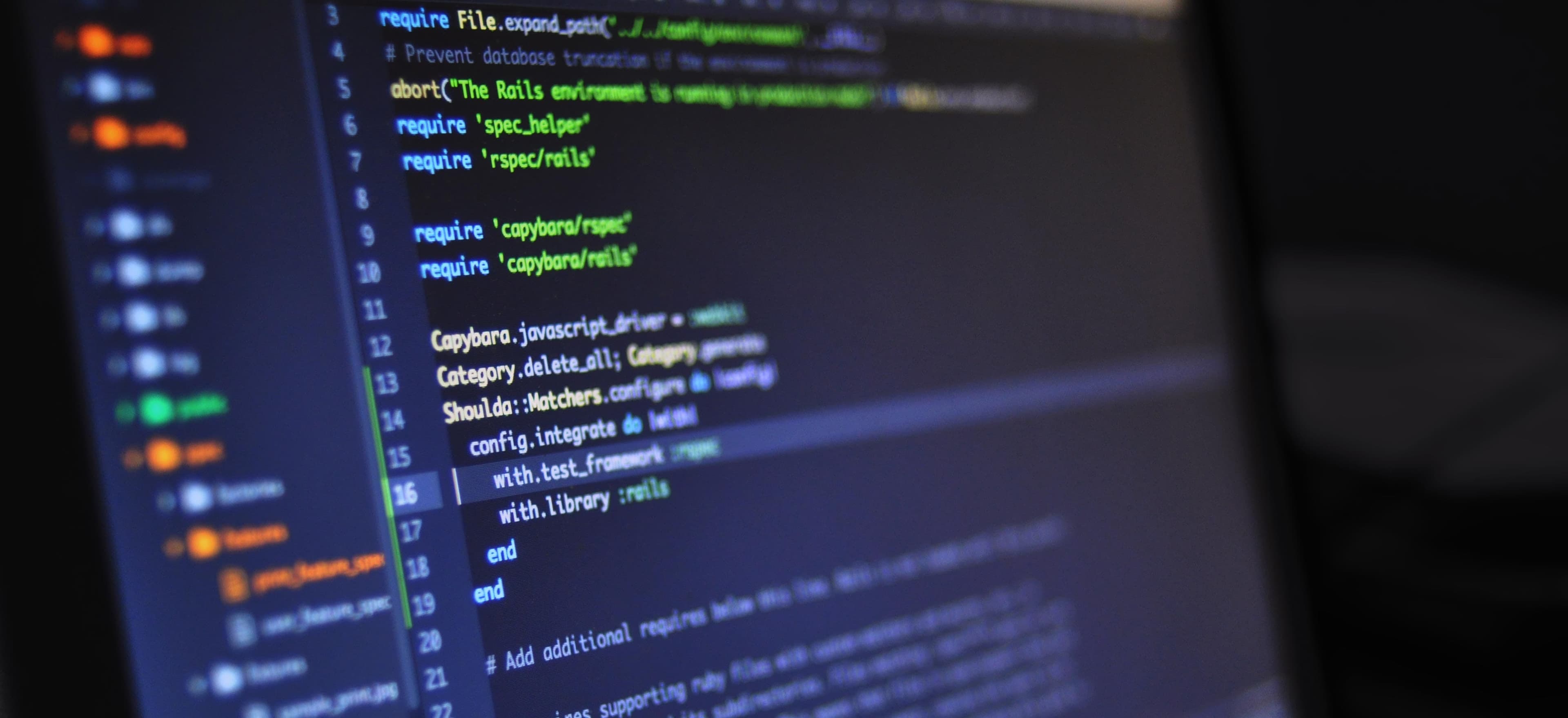
- Published on
The Importance of Preventing Java Program Vulnerabilities
Java is a powerful and versatile programming language used to develop a wide range of applications, ranging from mobile and web-based to enterprise software. However, despite its popularity, Java applications are not immune to vulnerabilities. In this blog post, we'll explore the importance of preventing vulnerabilities in Java programs and discuss best practices to secure your code.
Understanding Java Vulnerabilities
Before delving into prevention strategies, it's crucial to understand the types of vulnerabilities that can affect Java programs. Common vulnerabilities include:
-
Injection Attacks: These occur when untrusted data is sent to an interpreter as part of a command or query, leading to the execution of unintended commands.
-
Cross-Site Scripting (XSS): XSS vulnerabilities allow attackers to inject client-side scripts into web pages viewed by other users.
-
Insecure Deserialization: When untrusted data is deserialized by a Java application, it can lead to remote code execution or other attacks.
-
Insecure Direct Object References: This vulnerability occurs when an application exposes internal implementation objects to users.
Best Practices for Preventing Java Vulnerabilities
Input Sanitization
One of the fundamental strategies for preventing vulnerabilities in Java programs is input sanitization. By validating and sanitizing user input, you can prevent injection attacks and other security threats.
public class InputSanitization {
public String sanitizeInput(String input) {
// Perform input validation and sanitization
return sanitizedInput;
}
}
By enforcing strict input validation, you can ensure that only safe and expected data is processed by your Java application.
Secure Coding Practices
Adhering to secure coding practices is essential for preventing vulnerabilities. This involves using secure APIs and libraries, implementing proper error handling, and following coding standards such as avoiding hardcoded passwords and sensitive information in the code.
public class SecureCoding {
private static final String DATABASE_PASSWORD = "secure_password";
// Avoid hardcoded passwords in the code
public String getDatabasePassword() {
return DATABASE_PASSWORD;
}
}
By following secure coding principles, you can minimize the risk of introducing vulnerabilities into your Java programs.
Use of Security Libraries
Utilizing established security libraries like Apache Shiro or Spring Security can significantly enhance the security of your Java applications. These libraries provide robust authentication, authorization, and encryption capabilities that help mitigate common security risks.
// Example of using Apache Shiro for authentication
Subject currentUser = SecurityUtils.getSubject();
if (!currentUser.isAuthenticated()) {
UsernamePasswordToken token = new UsernamePasswordToken(username, password);
currentUser.login(token);
}
Integrating security libraries into your Java projects can bolster your defenses against potential attacks and vulnerabilities.
Regular Security Testing
Conducting regular security assessments and penetration testing of your Java applications is paramount for identifying and remedying vulnerabilities. By employing automated scanning tools and manual testing techniques, you can proactively uncover and address security weaknesses before they are exploited by malicious actors.
Keeping Dependencies Up to Date
Maintaining up-to-date dependencies is crucial for preventing vulnerabilities in Java programs. Regularly updating third-party libraries and components helps patch known security flaws and ensures that your application is not susceptible to outdated vulnerabilities.
Final Considerations
In conclusion, preventing vulnerabilities in Java programs is a critical aspect of developing secure and resilient software. By implementing input sanitization, secure coding practices, leveraging security libraries, conducting regular security testing, and staying abreast of dependency updates, you can significantly reduce the risk of security breaches and safeguard your Java applications against potential threats.
Ensuring the security of your Java programs not only protects your organization and users but also fosters trust and confidence in the quality of your software. With a proactive approach to security, you can fortify your Java applications and mitigate the ever-evolving landscape of cyber threats.
For additional insights into Java security best practices, consider referring to the OWASP Java Project and Oracle's Secure Coding Guidelines for Java SE.
Remember, the proactive prevention of vulnerabilities today ensures the resilience and reliability of your Java applications tomorrow.
Secure your Java code and stay ahead of potential threats!