Managing Proxies in JDK 11
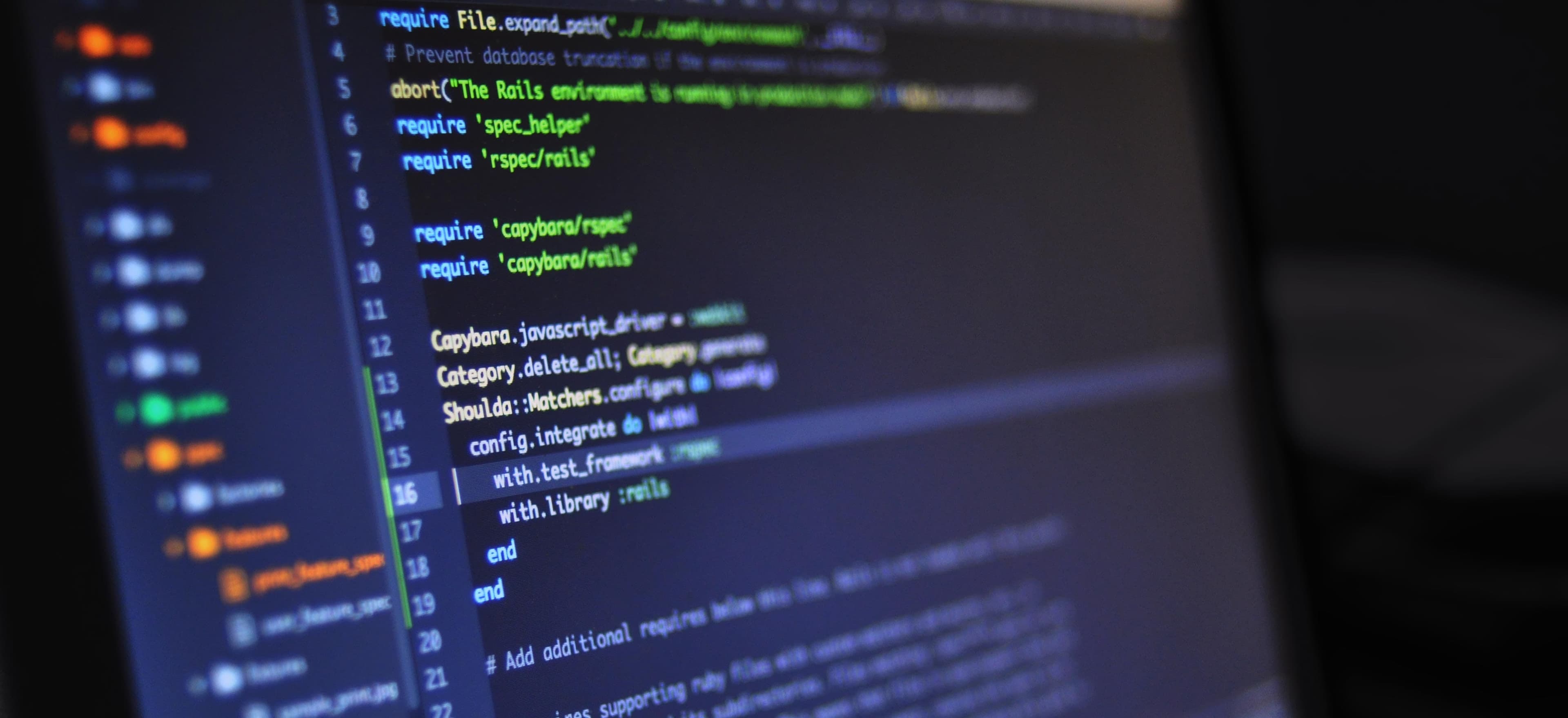
- Published on
A Complete Guide to Managing Proxies in JDK 11
In Java programming, a proxy acts as an intermediary between a client and a server. When it comes to JDK 11, efficiently managing proxies is essential for seamless communication between applications. In this comprehensive guide, we will delve into the intricacies of managing proxies in JDK 11, exploring the underlying concepts, best practices, and exemplary code snippets.
Understanding Proxies in JDK 11
In JDK 11, proxies play a pivotal role in enabling communication across distributed systems. They facilitate the interception and handling of method invocations, making them indispensable for tasks such as network communication, security, and logging.
JDK 11 Proxy Class
The java.lang.reflect
package in JDK 11 provides the Proxy
class, which allows the creation of dynamic proxy instances. This class enables the generation of proxy classes and instances at runtime, empowering developers to intercept method invocations and implement custom behavior.
Let's delve into an exemplary code snippet to comprehend the usage of Proxy
class in JDK 11.
import java.lang.reflect.Proxy;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
public class ProxyHandler implements InvocationHandler {
private Object target;
public ProxyHandler(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
// Custom logic before invoking the method
Object result = method.invoke(target, args);
// Custom logic after invoking the method
return result;
}
}
public class ProxyExample {
public static void main(String[] args) {
RealObject realObject = new RealObject();
InvocationHandler handler = new ProxyHandler(realObject);
RealObject proxyObject = (RealObject) Proxy.newProxyInstance(
RealObject.class.getClassLoader(),
RealObject.class.getInterfaces(),
handler
);
proxyObject.someMethod();
}
}
In the above code snippet, we create a dynamic proxy using the Proxy.newProxyInstance
method, passing the class loader, interfaces, and an instance of InvocationHandler
implementation. This facilitates the interception of method invocations and execution of custom logic.
JDK 11 Proxy Configuration
Configuring proxies in JDK 11 is integral for enabling communication between applications and external resources. The java.net
package provides the necessary classes and methods for managing proxy configuration in JDK 11.
Let's consider an example of configuring a proxy using ProxySelector
and Proxy
classes in JDK 11.
import java.net.*;
import java.io.IOException;
public class ProxyConfigurationExample {
public static void main(String[] args) {
ProxySelector.setDefault(new ProxySelector() {
@Override
public List<Proxy> select(URI uri) {
List<Proxy> proxyList = new ArrayList<>();
Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress("proxy.example.com", 8080));
proxyList.add(proxy);
return proxyList;
}
@Override
public void connectFailed(URI uri, SocketAddress sa, IOException ioe) {
// Handle connection failure
}
});
// Perform network operations using configured proxy
// ...
}
}
In the above code snippet, we set a custom ProxySelector
using ProxySelector.setDefault
to configure a proxy for all network operations. The select
method provides the logic for selecting the appropriate proxy based on the URI, while the connectFailed
method handles any connection failures.
Best Practices for Managing Proxies in JDK 11
-
Use dynamic proxies for cross-cutting concerns: Leverage the
Proxy
class to implement cross-cutting concerns such as logging, security, and performance monitoring without modifying the original source code. -
Handle proxy authentication securely: When configuring proxies that require authentication, ensure the secure handling of credentials to prevent unauthorized access.
-
Implement robust error handling: When dealing with proxy configuration and network operations, implement robust error handling to gracefully manage failures and timeouts.
-
Regularly review proxy configurations: Regularly review and update proxy configurations to align with evolving network infrastructure and security requirements.
-
Utilize proxy auto-configuration: Explore the use of proxy auto-configuration scripts or mechanisms to dynamically adapt to changing network environments.
Final Thoughts
Managing proxies in JDK 11 is imperative for establishing robust and secure communication channels between applications. By understanding the Proxy
class, configuring proxies, and adhering to best practices, developers can effectively harness the power of proxies in JDK 11 to facilitate seamless interactions across distributed systems.
In conclusion, proactive management of proxies empowers Java developers to build resilient and adaptive software systems, ensuring streamlined communication and enhanced security across diverse network environments.
For more in-depth insights into JDK 11 and related topics, consider exploring comprehensive resources such as Oracle's official documentation and Baeldung's Java tutorials.