Optimizing Iteration in Scala for Better Performance
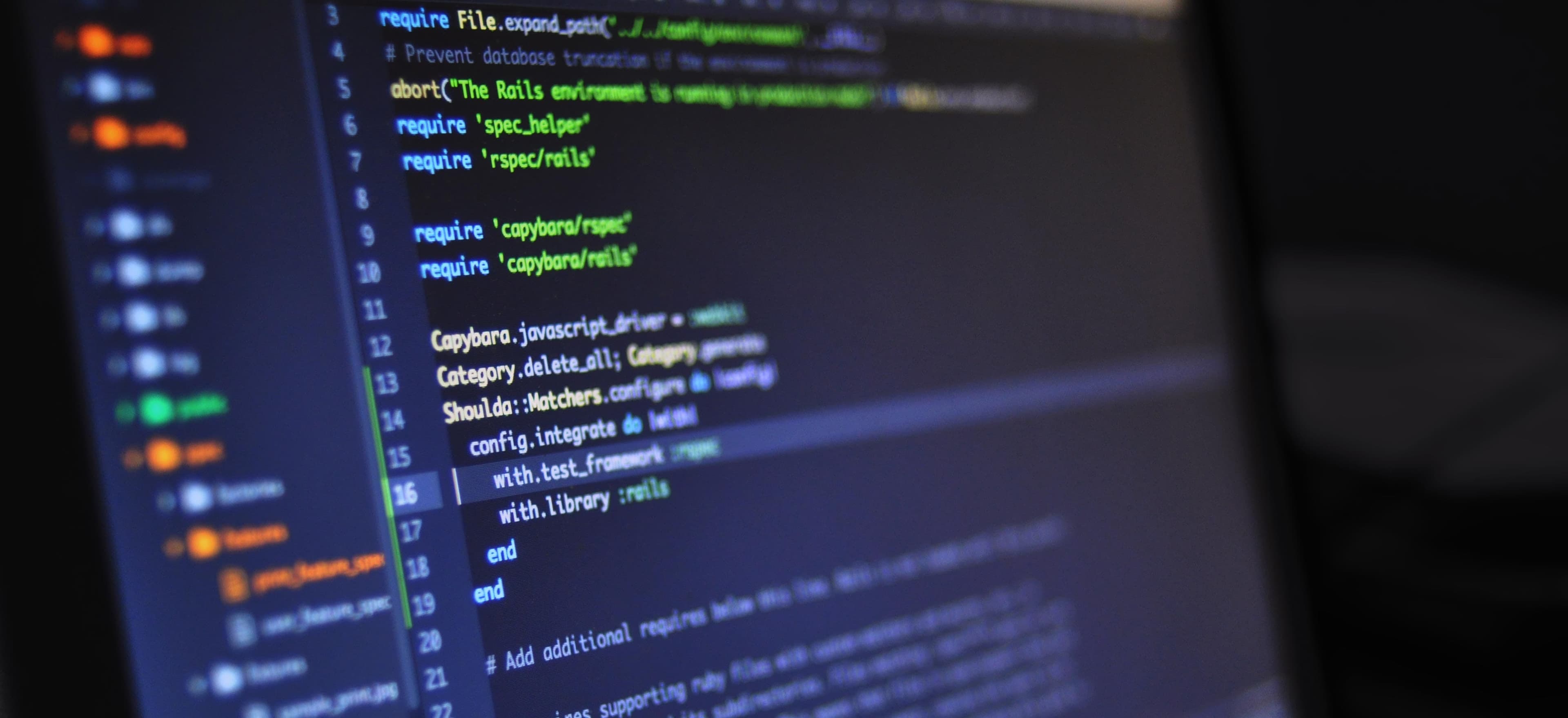
- Published on
Optimizing Iteration in Scala for Better Performance
When writing Scala code, it's essential to consider performance optimization for efficient execution. One area where performance can be significantly improved is through optimized iteration techniques. This blog post will focus on exploring different iteration strategies and techniques in Scala to achieve better performance.
Immutable vs Mutable Collections
When performing iteration operations, the choice between immutable and mutable collections can have a significant impact on performance. Immutable collections are thread-safe but can result in higher memory allocation and can be slower when iterating over large datasets. On the other hand, mutable collections can offer better performance due to their in-place modification capabilities.
However, in functional programming, immutability is encouraged for its safety and ease of reasoning about code. But there are scenarios where mutability can be leveraged for performance optimization.
// Immutable Collection
val immutableList = List(1, 2, 3, 4, 5)
// Mutable Collection
val mutableList = collection.mutable.ListBuffer(1, 2, 3, 4, 5)
While using mutable collections can improve performance, it's crucial to handle mutability carefully to avoid potential side effects.
Advanced Iterator Methods
Scala provides a rich set of iterator methods that can be used to optimize iteration performance and streamline code. These methods include map
, filter
, flatMap
, fold
, and reduce
, among others. Choosing the right iterator method for the task at hand can result in more efficient code execution.
val numbers = Seq(1, 2, 3, 4, 5)
// map - Applies the given function to each element in the list
val squaredNumbers = numbers.map(x => x * x)
// filter - Selects elements that satisfy a given predicate
val evenNumbers = numbers.filter(x => x % 2 == 0)
// flatMap - Similar to map, but it flattens the result
val nestedNumbers = List(List(1, 2), List(3, 4))
val flattenedNumbers = nestedNumbers.flatMap(x => x)
By utilizing these advanced iterator methods, the code can be optimized to achieve better performance by leveraging the built-in optimizations provided by these methods.
Lazy Evaluation
One powerful feature in Scala is its support for lazy evaluation. By using lazy evaluation, computations are deferred until their results are actually needed. This can lead to significant performance improvements, especially when dealing with large datasets or complex computation pipelines.
val numbers = (1 to 1000000).toList
val result = numbers
.view
.map(_ * 2)
.filter(_ % 3 == 0)
.take(100)
.toList
In this example, view
creates a lazy view of the collection, and the subsequent operations are lazily evaluated. This means that the map
, filter
, and take
operations are only performed as the elements are accessed, avoiding unnecessary computations.
Parallel Collections
When dealing with computationally intensive tasks that can be parallelized, Scala's parallel collections can be utilized to achieve significant performance gains by leveraging multi-core processors.
val numbers = (1 to 1000000).toList
val result = numbers.par
.map(_ * 2)
.filter(_ % 3 == 0)
.take(100)
.toList
By simply using .par
on the collection, the operations are automatically parallelized, distributing the workload across available CPU cores.
It's important to note that parallel collections are most effective for operations that are CPU-bound rather than I/O-bound, as the overhead of parallelization can outweigh the benefits for I/O-bound tasks.
Inline Foreach
In certain cases, using a foreach
loop can offer better performance compared to other iterator methods, especially when the goal is to perform side effects for each element in the collection without producing a new collection.
val numbers = Seq(1, 2, 3, 4, 5)
numbers.foreach(println)
In this example, using foreach
directly on the collection avoids creating an intermediate collection, resulting in better performance for side-effect operations.
Benchmarking and Profiling
It's important to benchmark and profile different iteration strategies to assess their actual impact on performance. Tools like JMH (Java Microbenchmarking Harness) and YourKit can be valuable for measuring and analyzing the execution time and memory usage of different iteration approaches.
The Last Word
Optimizing iteration in Scala is crucial for achieving better performance in applications. By understanding the trade-offs between different iteration techniques, leveraging advanced iterator methods, lazy evaluation, parallel collections, and inline foreach, developers can write more efficient and performant Scala code.
By carefully selecting the most appropriate iteration strategy for a given task and continuously benchmarking and profiling the code, it becomes possible to achieve significant performance improvements in Scala applications.
In conclusion, by deeply understanding the characteristics of various iteration techniques, developers can streamline their code for optimal performance, which is a critical aspect of professional Scala development.
To explore more about Scala iteration and performance optimization, you can dive into the official Scala documentation and Scala Performance Tips.
Happy optimizing!