Managing Data Consistency in Java EE Microservices
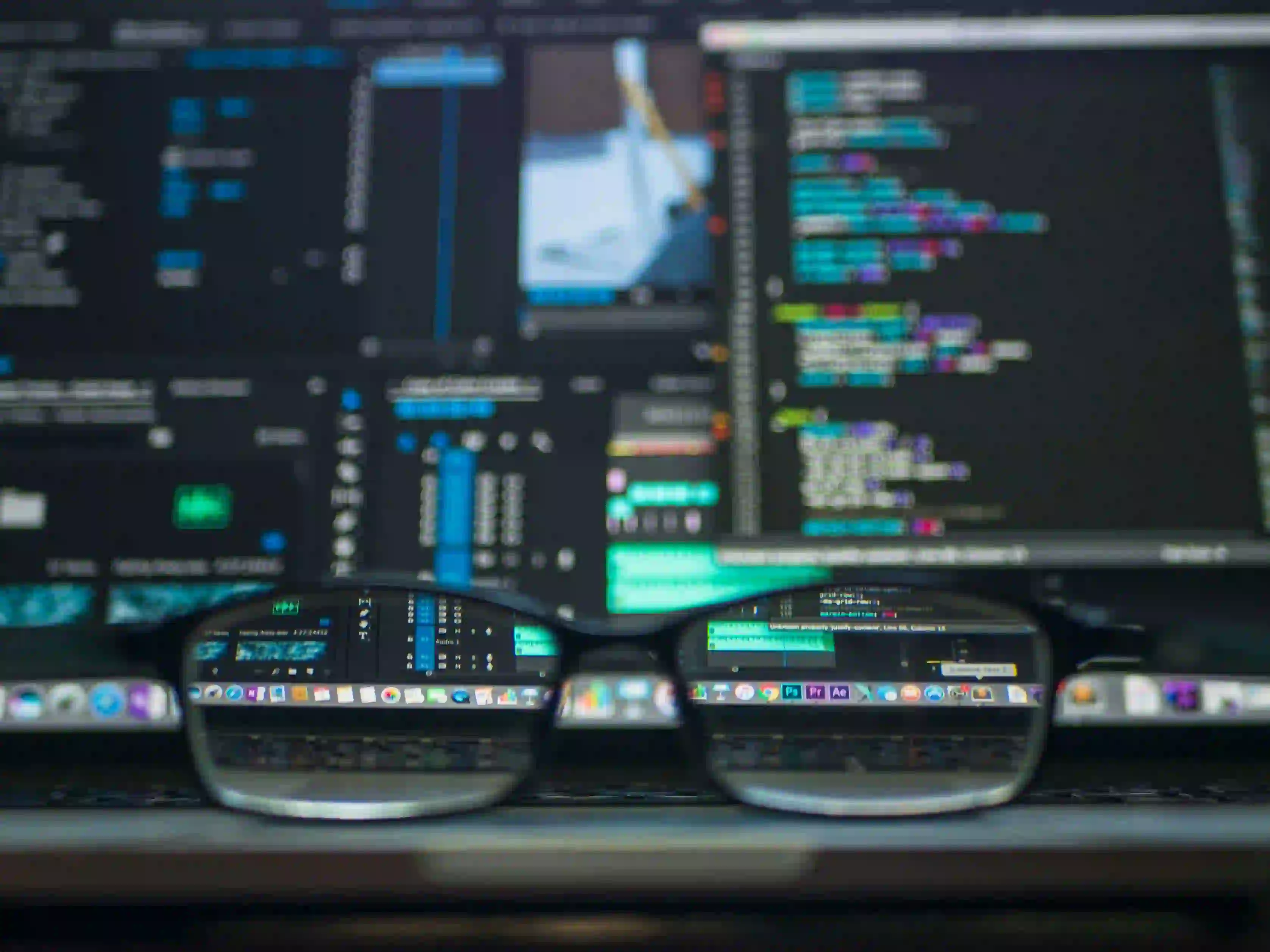
Managing Data Consistency in Java EE Microservices
In a microservices architecture, the data consistency between services becomes a crucial challenge. Java EE provides various mechanisms to handle data consistency in a distributed environment. In this article, we'll explore different strategies to manage data consistency in Java EE microservices.
Understanding Data Consistency
In a microservices architecture, each service has its own database, and multiple services often need to collaborate to fulfill a single operation. Maintaining data consistency ensures that all data across services is accurate and up-to-date. Inconsistencies can arise due to failures, network latency, and concurrent updates, making it essential to implement measures to manage data consistency effectively.
Synchronous Communication and Transactions
Using synchronous communication and distributed transactions can ensure strong data consistency in microservices. However, this approach introduces increased latency and complexity. Java EE provides the Java Transaction API (JTA) for handling distributed transactions, allowing multiple resources to be coordinated in a single transaction.
@TransactionAttribute(TransactionAttributeType.REQUIRED)
public void performTransaction() {
// Business logic performing database updates
}
The @TransactionAttribute
annotation in Java EE specifies the required transaction attribute for a method. It ensures that the method executes within a transaction, and if no transaction is active, a new transaction is started.
Asynchronous Communication and Eventual Consistency
An alternative approach to managing data consistency is through asynchronous communication and eventual consistency. Eventual consistency acknowledges that data updates may take time to propagate across services, allowing for temporary inconsistencies. Java EE provides support for asynchronous messaging through JMS (Java Message Service) and CDI (Contexts and Dependency Injection).
@Inject
private JMSContext jmsContext;
public void sendMessage(String message) {
jmsContext.createProducer().send(destination, message);
}
In this example, the sendMessage
method sends a message to a JMS destination asynchronously, allowing the sender to continue its operation without waiting for a response. The eventual consistency is achieved by processing the message at the receiver's end and updating the data asynchronously.
CQRS (Command Query Responsibility Segregation)
Command Query Responsibility Segregation (CQRS) is another approach to manage data consistency in microservices. It separates the read and write operations, using different models for each. Java EE supports CQRS through its support for creating separate command and query APIs using JAX-RS (Java API for RESTful Web Services) for write operations and JPA (Java Persistence API) for read operations, effectively segregating the responsibilities.
@Path("/commands")
@Stateless
public class CommandResource {
@POST
public Response createEntity(Entity entity) {
// Logic for creating entity
}
}
In this example, the CommandResource
class handles the write (create) operation using JAX-RS, while the read operations can be handled by separate resources using JPA.
Optimistic Concurrency Control
Optimistic Concurrency Control (OCC) is a strategy to manage data consistency by allowing concurrent access to data and detecting conflicts during updates. When using Java EE, JPA provides support for optimistic locking, where each entity has a version attribute that is used to detect conflicting updates.
@Version
private Long version;
By annotating a version attribute in an entity class, JPA automatically manages the versioning during updates. When a concurrent update is detected, an exception is thrown, allowing custom handling of the conflict.
Implementing Compensation Actions
In a distributed system, failures are inevitable, and managing data consistency during failure scenarios is critical. Java EE microservices can implement compensation actions to handle such scenarios. Compensation actions are designed to reverse the effects of a failed operation, ensuring that the system remains consistent despite failures.
@TransactionAttribute(TransactionAttributeType.REQUIRES_NEW)
public void compensate() {
// Logic to compensate for a failed operation
}
The @TransactionAttribute(TransactionAttributeType.REQUIRES_NEW)
annotation in Java EE ensures that the compensate
method runs within a new transaction, allowing it to undo the effects of a failed operation without affecting other ongoing transactions.
Final Thoughts
Managing data consistency in Java EE microservices demands a thorough understanding of the available strategies and their implications. Depending on the specific requirements of a microservices architecture, developers can choose the most suitable approach or a combination of approaches to ensure data consistency without compromising performance and reliability.
By leveraging the features provided by Java EE, such as JTA, JMS, CDI, JAX-RS, and JPA, developers can effectively implement data consistency mechanisms tailored to the needs of their microservices.
Implementing a combination of synchronous and asynchronous communication, utilizing CQRS, employing optimistic concurrency control, and incorporating compensation actions enables Java EE microservices to maintain data consistency while adapting to the distributed nature of the architecture.
Ultimately, Java EE empowers developers to design robust, scalable, and consistent microservices that form the foundation of modern, distributed systems.
For further information on Java EE and microservices, you can refer to the official Java EE documentation and explore the MicroProfile initiative for additional enhancements in building microservices using Java.