Mastering Java 8: Common Pitfalls in Grouping Collections
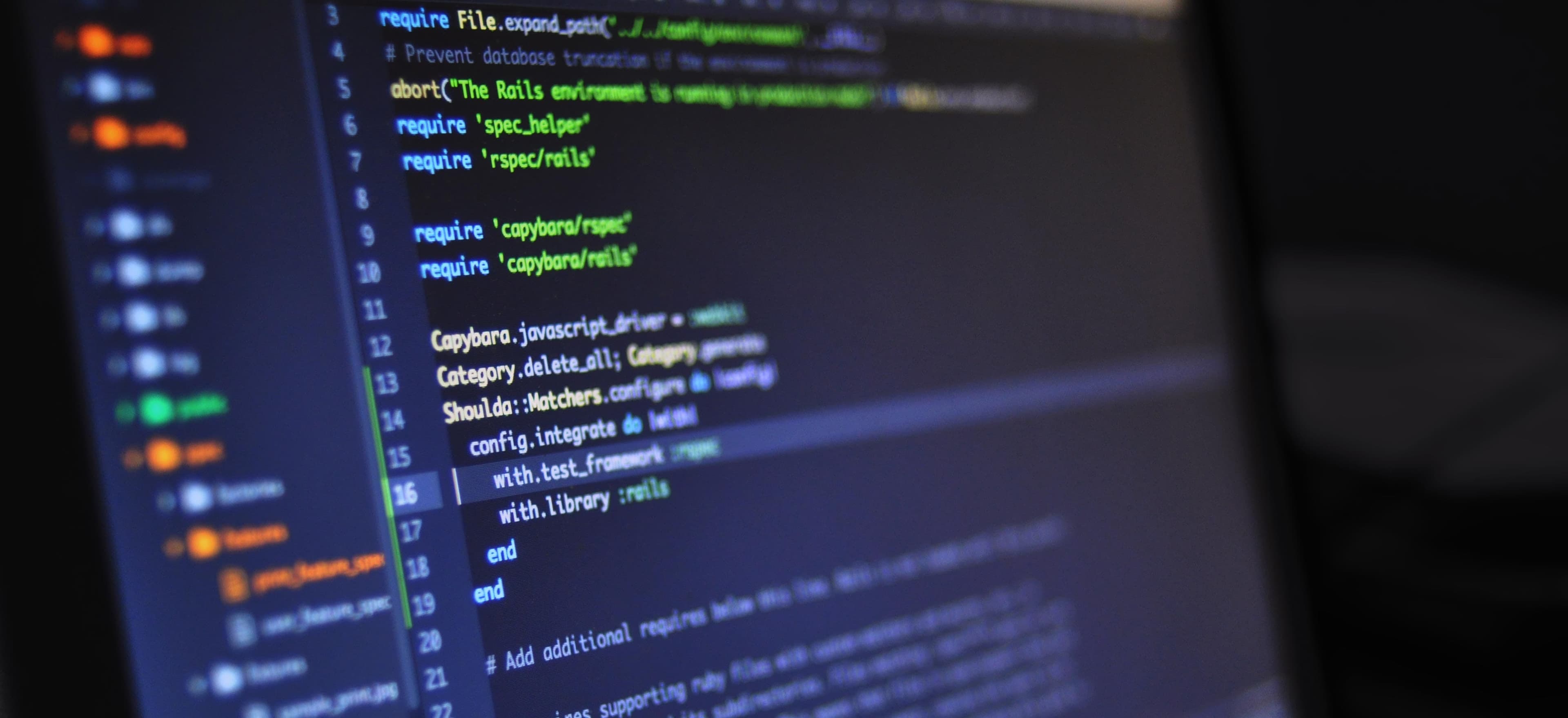
- Published on
Mastering Java 8: Common Pitfalls in Grouping Collections
Java 8 introduced a powerful Stream API that revolutionized the way we handle collections. With the introduction of functional programming concepts and the ability to process collections in a declarative manner, Java developers can now approach data manipulation in more efficient ways. However, while grouping collections may seem straightforward, developers often encounter several pitfalls that can lead to bugs, performance issues, and unexpected behavior. In this blog post, we will explore common pitfalls in grouping collections in Java 8 and how to avoid them.
Understanding the Stream API
Before diving into the pitfalls, it is essential to grasp the basics of the Stream API. A Stream in Java is a sequence of elements supporting sequential and parallel aggregate operations.
Example Code Snippet
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
Stream<String> nameStream = names.stream();
In the snippet above, we create a stream from a list of names. The Stream API allows us to perform various operations, such as filtering, mapping, and, importantly, grouping.
Grouping with Collectors
The Collectors
utility class provides several pre-defined implementations to collect elements from a stream. The most relevant for grouping are groupingBy()
and partitioningBy()
.
Example Code Snippet
Map<Integer, List<String>> groupedByLength = names.stream()
.collect(Collectors.groupingBy(String::length));
The code above groups names based on their lengths, resulting in a Map
where the key is the length, and the value is a list of names of that length.
Now that we understand the stream and grouping basics, let’s discuss the common pitfalls developers face.
Common Pitfalls in Grouping Collections
1. Overusing Grouping
One common mistake is overusing groupingBy()
. This may lead to unnecessary complexity and performance overhead, especially in large datasets. Grouping can create a significant amount of intermediate collections.
Solution
Limit the use of grouping when you do not need additional processing of the grouped data. Instead, use methods like filter()
, map()
, or perform the operation independently when appropriate.
2. Confusing Grouping and Partitioning
While both groupingBy()
and partitioningBy()
serve to classify elements, the latter splits elements into two groups based on a predicate. Developers often confuse them, leading to incorrect implementations.
Example Code Snippet
Map<Boolean, List<String>> partitionedNames = names.stream()
.collect(Collectors.partitioningBy(name -> name.length() > 3));
The above example correctly partitions names based on whether their length is greater than 3, resulting in a map with a boolean key indicating the condition.
Solution
Always assess whether your use case fits grouping or partitioning. Use partitioning for binary conditions.
3. Ignoring Null Values
Null values can create unintended consequences when using the Stream API. If your collection contains nulls, they may cause NullPointerExceptions
, especially during grouping.
Solution
Filter out nulls before processing, as shown below:
Map<Integer, List<String>> groupedNames = names.stream()
.filter(Objects::nonNull) // Filtering nulls
.collect(Collectors.groupingBy(String::length));
4. Not Using Collectors.toMap
While groupingBy()
returns a map, sometimes you may need to customize the type of the result. Failing to use Collectors.toMap()
correctly can result in IllegalStateException
due to duplicate keys.
Example Code Snippet
Map<String, Integer> nameLengthMap = names.stream()
.distinct()
.collect(Collectors.toMap(name -> name, String::length));
This code snippet creates a map where each name is mapped to its length, avoiding duplicates with distinct()
.
Solution
Always ensure your key generator produces unique keys, or handle collisions by providing a merge function:
Map<String, Integer> nameLengthMap = names.stream()
.collect(Collectors.toMap(name -> name, String::length, (existing, replacement) -> existing));
5. Forgetting Parallel Streams
While streams can improve performance, using them in parallel without understanding can lead to unexpected behavior. Grouping collections in parallel can yield inconsistent results if state is maintained.
Solution
When using parallel streams, ensure that the operations being used are thread-safe, or limit side effects.
Map<Integer, List<String>> parallelGroupedNames = names.parallelStream()
.collect(Collectors.groupingBy(String::length));
6. Using Immutable Collections
By default, the collections returned by groupingBy()
are mutable. This can lead to unintended modifications unless you appropriately handle the returned structures.
Solution
If you require an immutable collection, consider using Collectors.toUnmodifiableList()
:
Map<Integer, List<String>> unmodifiableGroupedNames = names.stream()
.collect(Collectors.groupingBy(String::length, Collectors.toUnmodifiableList()));
Wrapping Up
Mastering the grouping of collections in Java 8 can significantly streamline your data processing tasks. By understanding common pitfalls—from overusing grouping to managing null values—you can ensure your code remains efficient, clean, and effective.
For further reading, you can check out the official Java documentation on Streams and Java Collectors.
With these insights in hand, you are well on your way to mastering Java 8's grouping capabilities. Stay vigilant about these pitfalls and continue experimenting with the Stream API. Happy coding!