Choosing Between Java Lambda Streams and Groovy Closures
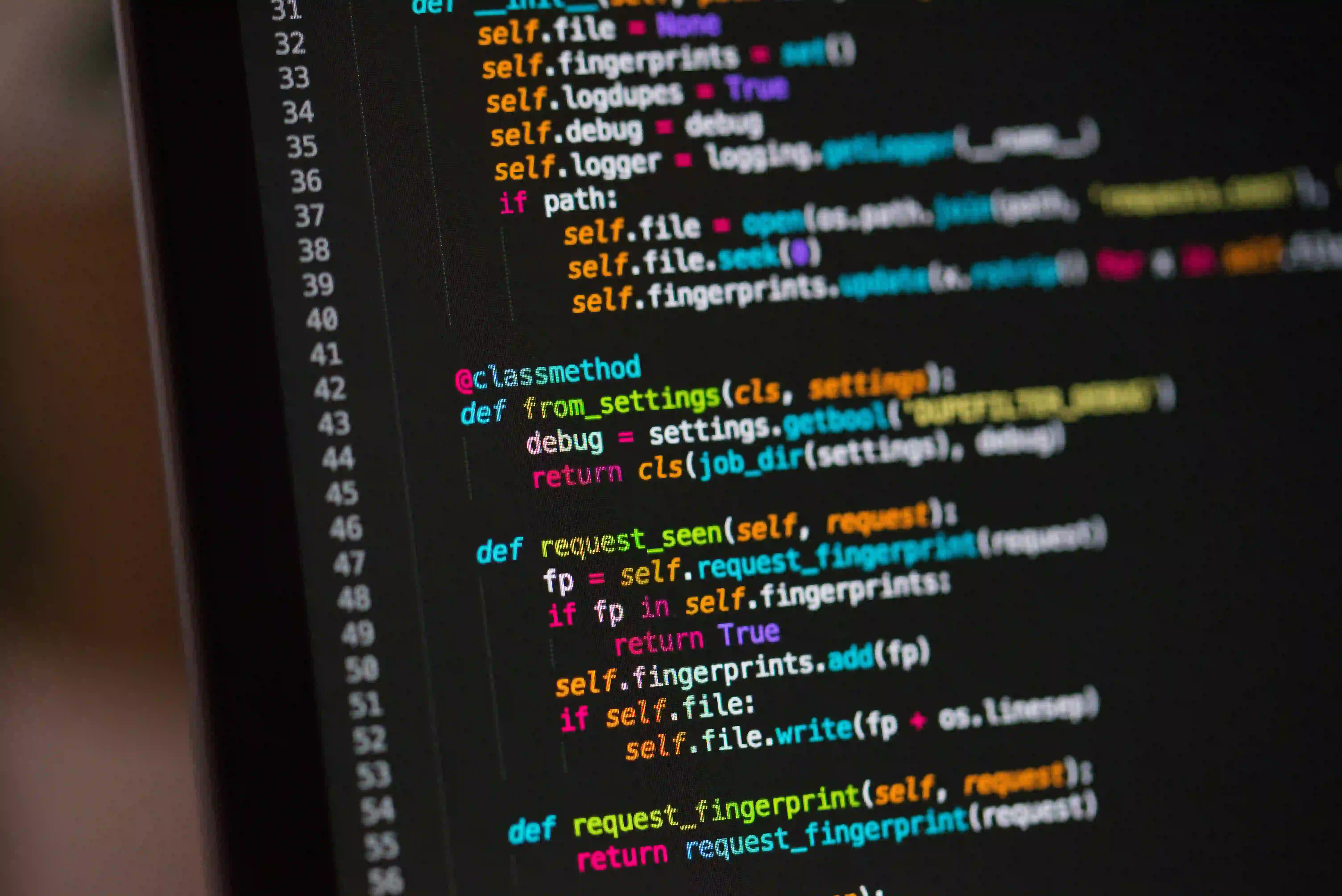
Understanding Java Lambda Streams and Groovy Closures
When it comes to functional programming in Java and Groovy, developers often find themselves faced with a choice between Java Lambda Streams and Groovy Closures. Both of these features enable a more concise and expressive way to work with collections and data manipulation. In this blog post, we'll explore the differences between these two approaches, when each is most appropriate, and how to use them effectively in your code.
Java Lambda Streams
Java 8 introduced Lambda expressions and the Stream API, which revolutionized the way Java developers work with collections. Lambda expressions allow you to write anonymous functions concisely, while the Stream API provides a fluent way to process collections of data.
Let's take a look at an example of using Java Lambda Streams to filter a list of numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6, 8, 10]
In this example, we use the stream()
method to convert the list of numbers into a Stream. Then, we use the filter()
method with a lambda expression to only keep the even numbers. Finally, the collect()
method gathers the filtered numbers into a new list. The result is a concise and clear way to filter a list.
Groovy Closures
Groovy, a dynamic language for the Java Virtual Machine, includes a powerful feature called Closures. Closures in Groovy are similar to Lambda expressions in Java, offering a concise syntax for defining anonymous functions.
Let's see a similar example using Groovy Closures to achieve the same result as the Java example:
def numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def evenNumbers = numbers.findAll { it % 2 == 0 }
println evenNumbers // Output: [2, 4, 6, 8, 10]
In this Groovy example, we use the findAll
method with a closure to filter the list of numbers and keep only the even numbers. The syntax is more concise compared to the Java example, making it easier to read and understand the intent of the code.
When to Use Java Lambda Streams
Java Lambda Streams are a natural choice when working with Java collections and APIs. They integrate seamlessly with the existing Java libraries and provide a consistent way to manipulate data.
Use Java Lambda Streams when:
- Working with Java collections or arrays
- Taking advantage of the rich set of Stream API operations like
map
,filter
, andreduce
- Needing compatibility with existing Java code and libraries
When to Use Groovy Closures
Groovy Closures are well-suited for scenarios where you want a more expressive and concise syntax for working with collections and data transformations. Groovy's dynamic nature and syntactic sugar make it a strong choice for concise and readable code.
Use Groovy Closures when:
- Dealing with data manipulation and transformation tasks
- Wanting a more expressive and readable syntax for collection operations
- Working on Groovy projects or with Groovy-based libraries and frameworks
Comparing Performance
It's essential to consider performance when choosing between Java Lambda Streams and Groovy Closures.
Java Lambda Streams are optimized and benefit from the underlying improvements in the Java compiler and runtime. They provide good performance, especially when working with large data sets.
On the other hand, Groovy Closures, being part of a dynamic language, may come with some performance overhead compared to Java Lambda Streams. While Groovy offers powerful features, it's important to measure and profile performance when using closures extensively, especially with large data sets.
Key Takeaways
In conclusion, Java Lambda Streams and Groovy Closures both offer powerful ways to work with collections and perform data manipulations. Java Lambda Streams are a natural fit for Java projects, providing a consistent and optimized way to work with collections. Groovy Closures, on the other hand, offer a more expressive and concise syntax, making them a great choice for Groovy projects and scenarios where readability and conciseness are valued.
Understanding the strengths and use cases of each approach allows you to make informed decisions when choosing between Java Lambda Streams and Groovy Closures in your projects. Regardless of the choice, both Java and Groovy provide powerful tools for functional programming and working with collections, offering developers flexibility and expressiveness in their code.