Handling Matrix Variables in Spring 3.2: Parsing and Using User-Input Data
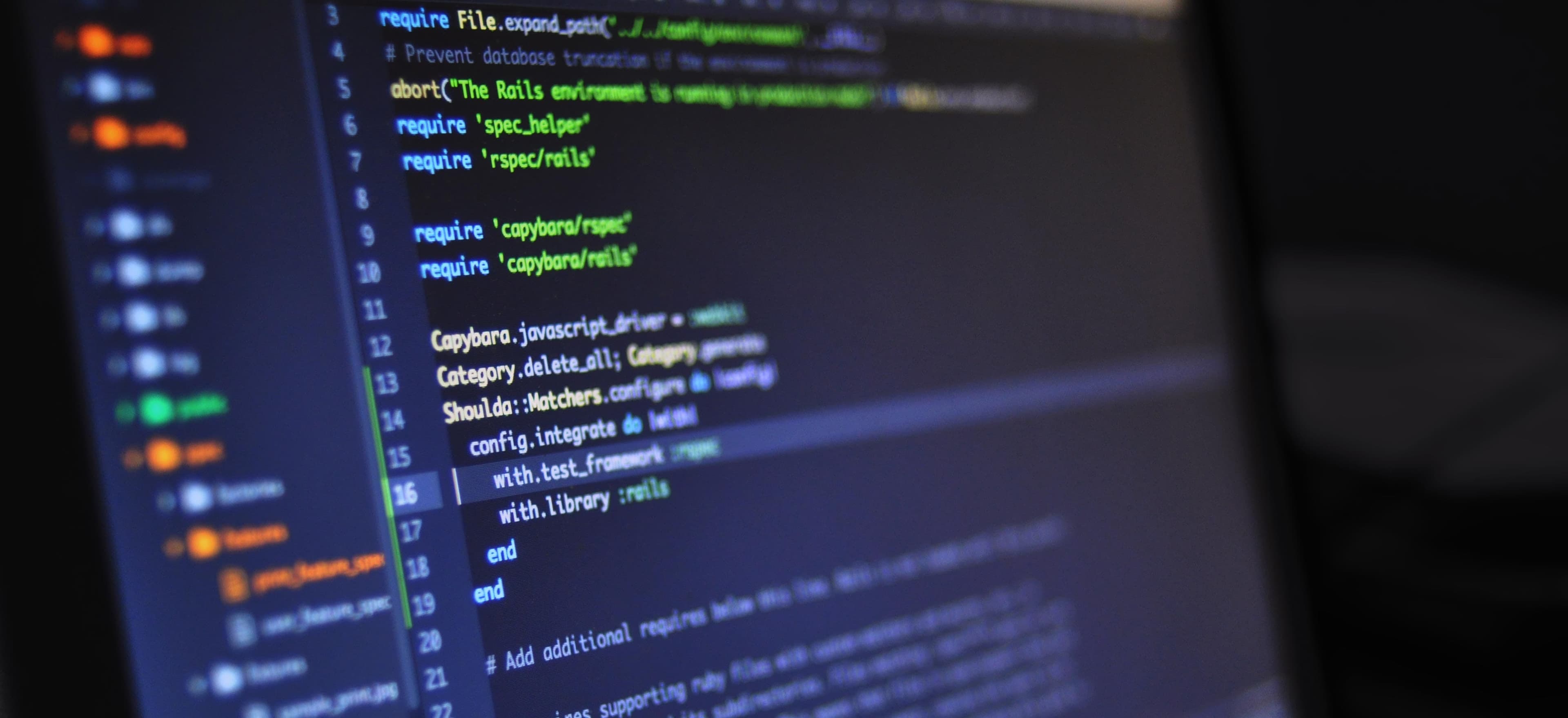
- Published on
Handling Matrix Variables in Spring 3.2: Parsing and Using User-Input Data
When building web applications, it's crucial to handle user-input data effectively. In Spring 3.2, a powerful feature called matrix variables allows developers to parse and use complex, user-input data conveniently. This blog post will delve into the concept of matrix variables in Spring 3.2 and guide you through the process of parsing and utilizing them effectively.
What are Matrix Variables?
Matrix variables are essentially a set of key-value pairs embedded in a URI path segment. They provide a way to pass multiple parameters to a single path segment in a URL. For instance, consider the following URL:
https://www.example.com/products;brand=apple;color=red/123
In this URL, brand=apple
and color=red
are matrix variables associated with the products
path segment.
Why Use Matrix Variables?
Matrix variables can be particularly useful in RESTful APIs, where they offer a cleaner and more expressive way to pass parameters related to a specific resource. They can also improve the readability and SEO-friendliness of URLs by structuring the parameters directly within the path.
Parsing Matrix Variables in Spring 3.2
In Spring 3.2, parsing matrix variables is straightforward. Let's consider a simple example where we have a REST endpoint that accepts matrix variables for filtering products.
@RestController
@RequestMapping("/products")
public class ProductController {
@GetMapping("/{category}")
public String getProductsByCategory(@MatrixVariable Map<String, List<String>> matrixVars,
@PathVariable String category) {
// Process and use the matrixVars to filter products
return "Filtered products for category: " + category;
}
}
In the above code, the @MatrixVariable
annotation is used to parse the matrix variables. The matrixVars
map will contain the key-value pairs parsed from the matrix variables in the URL.
Accessing Specific Matrix Variables
Sometimes, you may need to access specific matrix variables within the mapping code. In such cases, you can use the @MatrixVariable
annotation directly on method parameters.
@GetMapping("/{category}")
public String getProductsByCategory(@MatrixVariable(name="brand") List<String> brands,
@MatrixVariable(name="color") List<String> colors,
@PathVariable String category) {
// Use the brands and colors to filter products
return "Filtered products for category: " + category;
}
By specifying the name
attribute in @MatrixVariable
, you can retrieve specific matrix variables as method parameters.
Utilizing Matrix Variables in Query Mapping
Matrix variables can also be used in conjunction with @RequestMapping
to create powerful query mappings.
@GetMapping("/search/{location}")
public String searchProducts(@MatrixVariable(pathVar="location") Map<String, List<String>> filters,
@PathVariable String location) {
// Use the matrix variables to perform location-based product search
return "Products available at location: " + location;
}
In this example, matrix variables associated with the location
path segment are used to filter products based on location.
The Bottom Line
In Spring 3.2, matrix variables provide an elegant way to pass and parse user-input data within the URI path. By leveraging matrix variables, you can create more expressive and SEO-friendly URLs while effectively handling complex parameters related to specific resources.
To dive deeper into Spring 3.2 and matrix variables, refer to the official Spring documentation and explore the various capabilities and best practices associated with this feature.