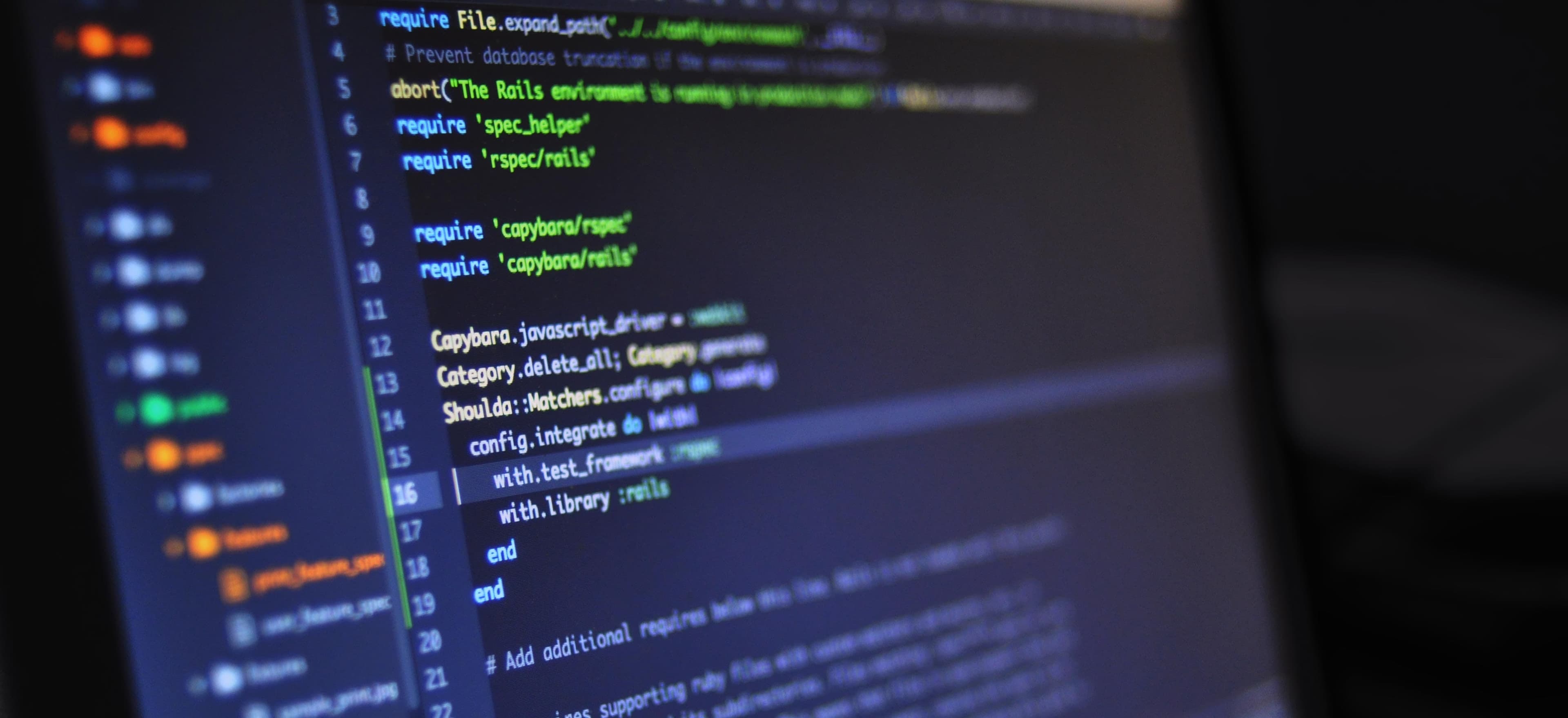
- Published on
Securing JAX-RS Endpoints with JAAS
Securing Java API for RESTful Web Services (JAX-RS) endpoints is paramount in ensuring the safety and integrity of the data being served. Java Authentication and Authorization Service (JAAS) provides a robust framework for providing authentication and authorization functionality in Java applications. In this blog post, we will delve into securing JAX-RS endpoints using JAAS, discussing its implementation, benefits, and best practices.
Understanding JAAS
JAAS, as the name suggests, comprises two main components: authentication and authorization.
- Authentication: Involves the process of validating the identity of a user.
- Authorization: Focuses on determining whether a user has the required permissions to access a particular resource.
This framework enables the decoupling of the application code from the authentication and authorization mechanisms, thus promoting modularity and reusability.
Implementing JAAS for JAX-RS Endpoints
Setup
Firstly, ensure that you have the necessary JAAS configuration set up. This typically involves defining the LoginModule and associating it with a Realm. A LoginModule is responsible for authenticating users, while a Realm provides access to user and role information.
Configuration
// JAAS Configuration
MyApp {
com.example.auth.CustomLoginModule required;
};
// Associate the application with the configuration
System.setProperty("java.security.auth.login.config", "/path/to/jaas.config");
Integration with JAX-RS
In a JAX-RS context, you can leverage JAAS by creating a custom SecurityInterceptor that intercepts requests and performs the necessary authentication and authorization checks.
@Provider
public class SecurityInterceptor implements ContainerRequestFilter {
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
// Perform authentication
if (!authenticationSucceeds) {
requestContext.abortWith(Response.status(Response.Status.UNAUTHORIZED).build());
return;
}
// Perform authorization
if (!authorizationSucceeds) {
requestContext.abortWith(Response.status(Response.Status.FORBIDDEN).build());
}
}
}
Benefits of Using JAAS with JAX-RS
By integrating JAAS with JAX-RS, you can achieve the following benefits:
-
Modularity: JAAS promotes separation of concerns by handling authentication and authorization separately from the application logic. This allows for easier maintenance and promotes code reusability.
-
Standardization: JAAS provides a standardized approach to authentication and authorization, ensuring that best practices are followed and potential security vulnerabilities are mitigated.
-
Scalability: With JAAS, it becomes simpler to scale an application, as the authentication and authorization logic is handled independently, allowing for easier integration with other systems.
Best Practices
Role-Based Access Control
Employ role-based access control (RBAC) to enforce authorization. Specify role-based permissions within your application and ensure that only authorized users can access specific resources.
Secure Configuration
Ensure that sensitive configuration related to JAAS, such as login module details and realm settings, is adequately secured. Avoid hardcoding sensitive information and consider encryption where appropriate.
Error Handling
Implement robust error handling mechanisms within the SecurityInterceptor to provide clear and informative responses to failed authentication and authorization attempts.
Logging and Monitoring
Integrate comprehensive logging and monitoring to track authentication and authorization activities. This facilitates the identification of suspicious behavior and aids in auditing.
Wrapping Up
Incorporating JAAS with JAX-RS endpoints is a crucial step in bolstering the security of your RESTful services. By following best practices and diligently implementing JAAS, you can ensure that only authenticated and authorized users gain access to your resources, thereby fortifying your application against unauthorized access.
To delve deeper into the world of JAAS, you can refer to the official documentation. Additionally, for a more hands-on approach, explore the JAAS Tutorial provided by Oracle for practical insights and implementation guidance.
Secure your JAX-RS endpoints with JAAS today and fortify your application's defenses against unauthorized access!
Remember, in the world of web services, security is not a feature; it's a necessity.