Building Real-Time Big Data Applications
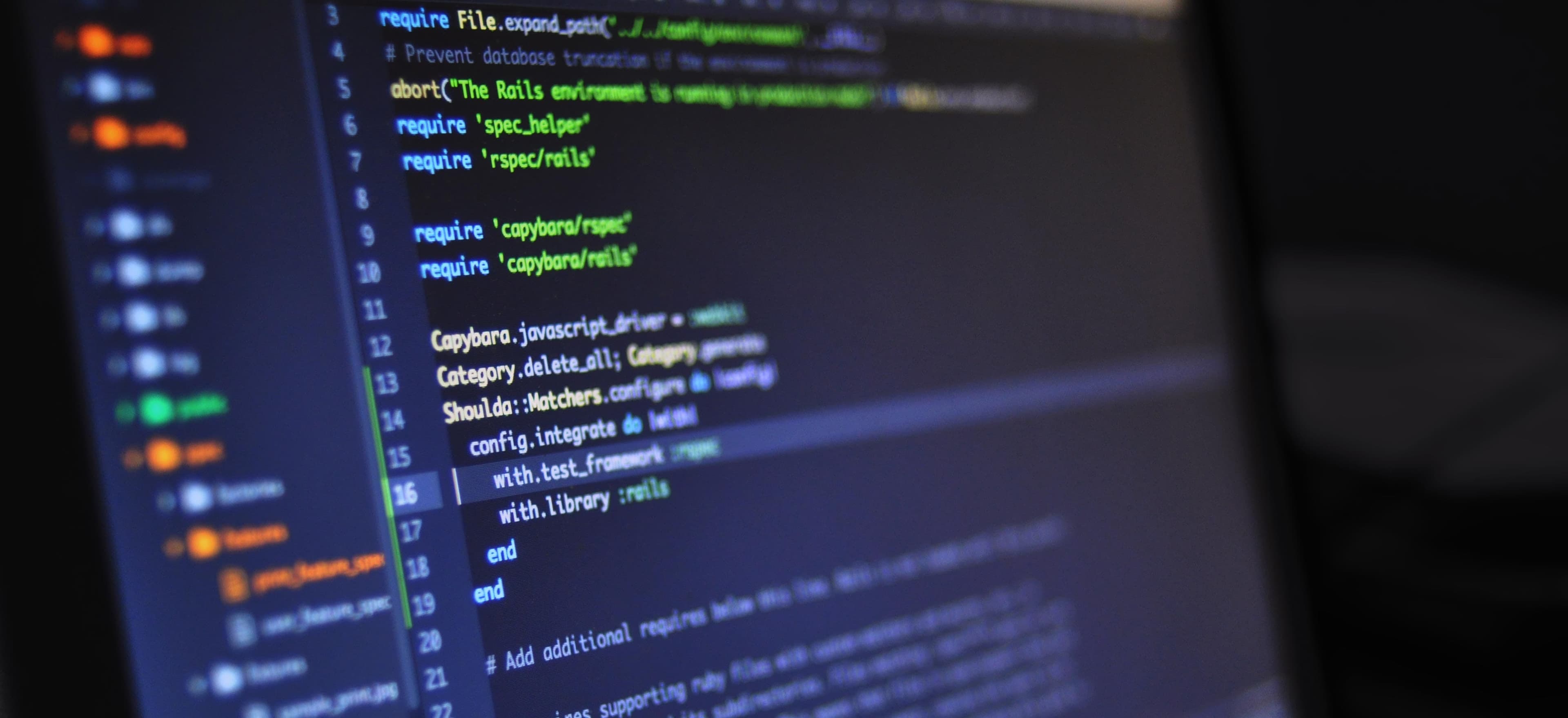
- Published on
Building Real-Time Big Data Applications with Java
In today's data-driven world, the need for real-time big data processing has become crucial for various industries. Java, with its scalability, reliability, and performance, has emerged as a top choice for building real-time big data applications. In this article, we'll explore how Java can be used to develop real-time big data applications, focusing on its key strengths and best practices.
Why Use Java for Real-Time Big Data Applications
Scalability and Performance
Java's multi-threading and concurrency support make it well-suited for handling big data processing tasks in real-time. The use of frameworks like Apache Kafka and Apache Flink further enhances Java's capabilities for building scalable and performant real-time data processing pipelines.
Robust Ecosystem
Java boasts a rich ecosystem of libraries, frameworks, and tools for big data processing, such as Apache Hadoop, Apache Spark, and Apache Storm. These tools provide powerful capabilities for real-time data ingestion, processing, and analysis.
Enterprise Support
Java is widely adopted in enterprise environments, making it a natural choice for building real-time big data applications that need to meet enterprise-level requirements for reliability, security, and maintainability.
Key Components of Real-Time Big Data Applications
Apache Kafka for Data Ingestion
Apache Kafka is a distributed streaming platform that provides a scalable and fault-tolerant solution for collecting and processing real-time data streams. Java's robust support for Kafka through its client libraries makes it an ideal choice for building real-time data ingestion pipelines.
Properties props = new Properties();
props.put("bootstrap.servers", "kafka-broker1:9092,kafka-broker2:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
In this snippet, we initialize a Kafka producer in Java to publish data to a Kafka topic. The use of Java's strong typing and the Kafka client library's integration showcases the ease of building real-time data ingestion components.
Apache Flink for Real-Time Processing
Apache Flink is a powerful stream processing framework that offers low-latency and high-throughput processing of real-time data streams. Java's native support for Flink's APIs and data types enables developers to build complex event processing and analytics applications with ease.
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<String> dataStream = env.addSource(new FlinkKafkaConsumer<>("topic", new SimpleStringSchema(), properties));
Here, we demonstrate the use of Java to create a Flink data stream from a Kafka topic, highlighting the seamless integration between Java and Flink for real-time data processing.
Apache Spark for Real-Time Analytics
Apache Spark's structured streaming APIs and in-memory processing capabilities make it an ideal choice for building real-time analytics applications. Java's interoperability with Spark's rich set of APIs allows developers to harness the full potential of Spark for real-time data analysis.
SparkSession spark = SparkSession.builder()
.appName("RealTimeAnalytics")
.getOrCreate();
Dataset<Row> rawData = spark
.readStream()
.format("kafka")
.option("kafka.bootstrap.servers", "kafka-broker1:9092,kafka-broker2:9092")
.load();
In this example, we use Java to create a Spark session and ingest data from Kafka for real-time analytics, showcasing the seamless integration between Java and Spark for building real-time big data applications.
Best Practices for Developing Real-Time Big Data Applications in Java
Use of Design Patterns
Utilize design patterns such as the observer pattern, reactor pattern, and actor model to architect resilient and scalable real-time big data applications in Java. These patterns help manage the complexities of handling concurrent data streams and processing.
Fault Tolerance and Recovery
Implement fault-tolerant strategies using Java's exception handling and resilience patterns to ensure the reliability of real-time big data applications. This includes handling network failures, data inconsistencies, and other transient errors.
Performance Optimization
Leverage Java's profiling and performance tuning tools to identify and optimize performance bottleneks in real-time big data processing pipelines. This includes efficient resource utilization, minimizing garbage collection overhead, and optimizing parallel execution.
The Closing Argument
Java's robustness, scalability, and rich ecosystem make it a compelling choice for building real-time big data applications. With its seamless integration with leading big data processing frameworks such as Apache Kafka, Apache Flink, and Apache Spark, Java empowers developers to create high-performance, real-time big data applications that meet the demands of modern data processing requirements.
By following best practices in design, fault tolerance, and performance optimization, developers can harness the full potential of Java for building real-time big data applications that drive insights and value from large-scale data streams.
In conclusion, Java stands as a formidable contender for developing real-time big data applications, and its versatility and efficiency in this domain make it a key player in the ever-evolving landscape of big data processing.