Maximize Performance with Java 7 Fork/Join Framework Tips
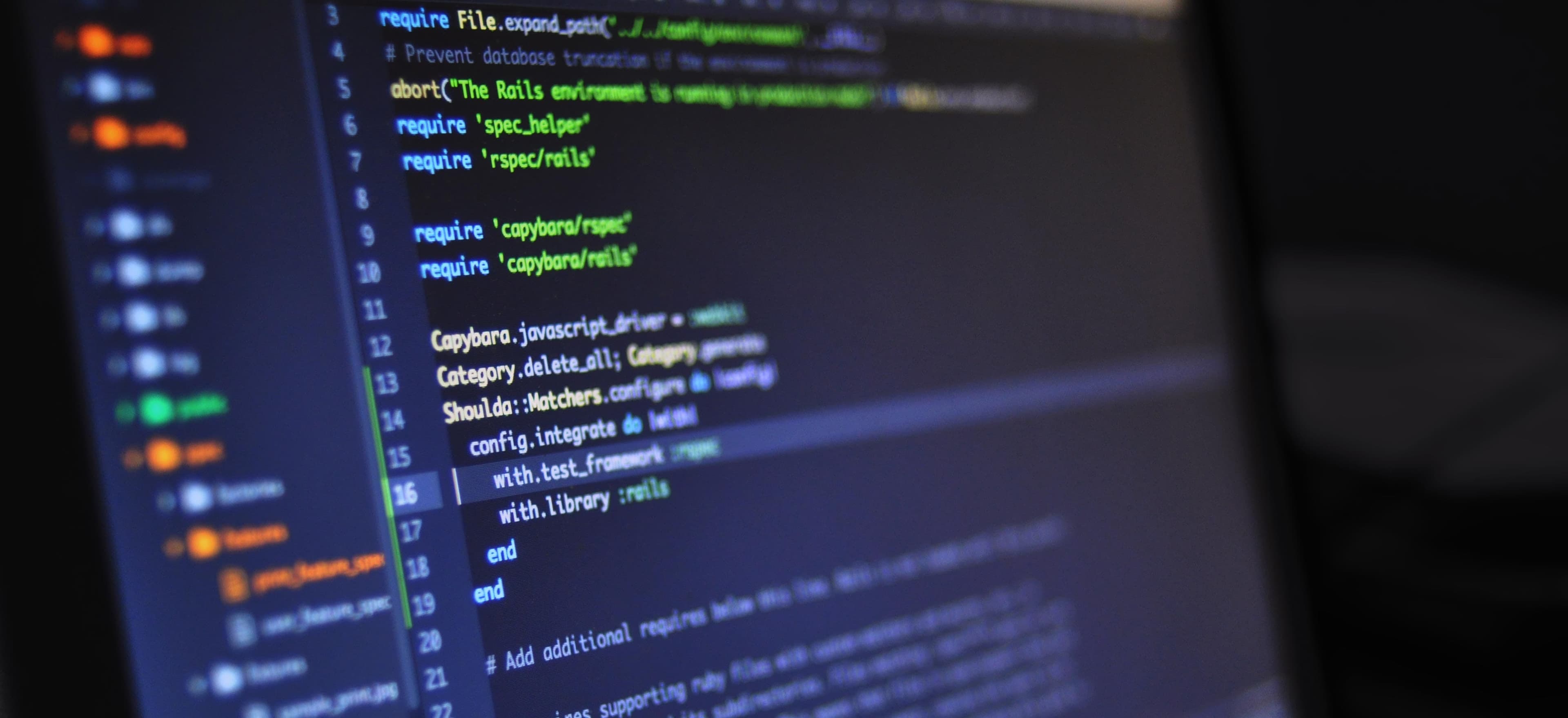
- Published on
Maximize Performance with the Java 7 Fork/Join Framework: Tips and Strategies
Java has always been a language that promotes performance and efficiency. With the introduction of the Fork/Join Framework in Java 7, parallel processing of tasks became much simpler and more efficient. This framework is designed to take advantage of multi-core processors by optimizing work distribution among different threads.
In this post, we will explore the main components of the Fork/Join Framework, how it works, and essential tips to maximize performance when using it.
Understanding the Fork/Join Framework
The Fork/Join Framework is based on the concept of "divide and conquer". This means that a computation task can be broken down into smaller sub-tasks that can be executed concurrently. The framework deals with the challenges of managing threads and synchronization, allowing developers to focus on task division and combination.
Key Components
-
ForkJoinPool: This is the heart of the framework. It manages the worker threads and facilitates task execution.
-
RecursiveTask and RecursiveAction: These classes represent tasks that can return a result and those that do not, respectively.
-
Fork and Join Methods: The
fork()
method is used to initiate the execution of a task asynchronously, while thejoin()
method is used to retrieve the result.
Implementing the Fork/Join Framework
Let’s start with a simple example where we use the Fork/Join Framework to calculate the sum of a large array. This will help illustrate the functionality and the way tasks are split and managed.
Example Code
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class SumTask extends RecursiveTask<Long> {
private static final int THRESHOLD = 1000; // Threshold to split the task
private final long[] numbers;
private final int start;
private final int end;
public SumTask(long[] numbers, int start, int end) {
this.numbers = numbers;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
// Check if the task is small enough to compute directly
if (end - start <= THRESHOLD) {
long sum = 0;
for (int i = start; i < end; i++) {
sum += numbers[i];
}
return sum;
}
// Split the task into two subtasks
int middle = (start + end) / 2;
SumTask leftTask = new SumTask(numbers, start, middle);
SumTask rightTask = new SumTask(numbers, middle, end);
// Fork the left task
leftTask.fork();
// Compute the right task and wait for the left task to complete
long rightResult = rightTask.compute();
long leftResult = leftTask.join(); // Combine results
return leftResult + rightResult;
}
public static void main(String[] args) {
long[] numbers = new long[100000];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1; // Initialize array with numbers from 1 to 100000
}
ForkJoinPool pool = new ForkJoinPool(); // Create the ForkJoinPool
SumTask task = new SumTask(numbers, 0, numbers.length); // Create the task
long result = pool.invoke(task); // Invoke the task
System.out.println("The sum is: " + result);
}
}
Code Commentary
-
ForkJoinPool: This is created with the default settings, but you can configure it for your specific needs (e.g., thread count).
-
Threshold: The
THRESHOLD
constant determines the point at which the algorithm stops splitting tasks. A higher threshold means fewer tasks but more work per task. A lower threshold can lead to many small tasks. -
Divide and Conquer: The
compute
function checks whether the current workload can be executed directly (if small enough). If it's not, it divides the task into two parts, forks the left task, and computes the right task. -
Results Combination: It retrieves the result from the left task with
join()
after computing the right task.
Benefits of Using Fork/Join Framework
-
Efficiency: Utilizing multiple cores improves performance and reduces execution time.
-
Simplicity: The Fork/Join Framework abstracts away the complexities of manual thread management.
-
Improved Resource Utilization: It leverages idle CPU cores, which enhances overall productivity.
Tips to Maximize Performance
While the Fork/Join Framework is powerful, there are specific strategies you can employ to maximize its performance.
1. Optimize Task Granularity
Choosing the right granularity is crucial for performance. If tasks are too small, the overhead of managing them might outweigh the benefits of parallelism. If they're too large, you won’t fully utilize the available cores. Finding the right balance requires testing and profiling.
2. Reduce Contention
Contention occurs when multiple threads attempt to access shared resources simultaneously. It can be minimized by:
-
Avoiding Shared State: Design your tasks to operate primarily on local state data.
-
Immutable Objects: Use immutable objects where possible, which can be shared safely between tasks.
3. Use Work Stealing Appropriately
ForkJoinPool implements a work-stealing algorithm where idle threads can "steal" tasks from busy threads. However, this works best when tasks are of relatively equal size. If you have widely varying task sizes, consider modifying your splitting strategy to yield more uniform tasks which enables effective work stealing.
4. Monitor and Tune
Use Java Performance Monitoring tools to analyze performance. Profile your application to identify bottlenecks and make adjustments.
-
Java VisualVM: A great tool for monitoring Java applications.
-
JProfiler: It can help identify performance issues within your Fork/Join tasks.
5. Leverage Fork/Join Pool Common Pool
The ForkJoinPool provides a common pool, which can be shared across different parts of your application. Reusing the same pool can help manage resources more effectively and avoid thread exhaustion.
Here’s how you can create a shared instance:
import java.util.concurrent.ForkJoinPool;
public class SharedForkJoinPool {
private static final ForkJoinPool COMMON_POOL = new ForkJoinPool();
public static ForkJoinPool getCommonPool() {
return COMMON_POOL;
}
}
To Wrap Things Up
The Fork/Join Framework has opened the doors to simpler and more efficient parallel programming in Java. By understanding its components and best practices, you can significantly improve the performance of your applications.
When you design your tasks, always aim for optimal granularity, avoid excessive contention, and use monitoring tools to guide your adjustments. With these practices, you can take full advantage of what Java 7 has to offer in terms of concurrent programming.
Further Reading
- Java Concurrency in Practice by Brian Goetz
- Oracle's Official Documentation on Fork/Join Framework
Feel free to dive deeper into the techniques described above and continuously refine your parallel programming skills. Happy coding!