Managing Blocking in Java Concurrency
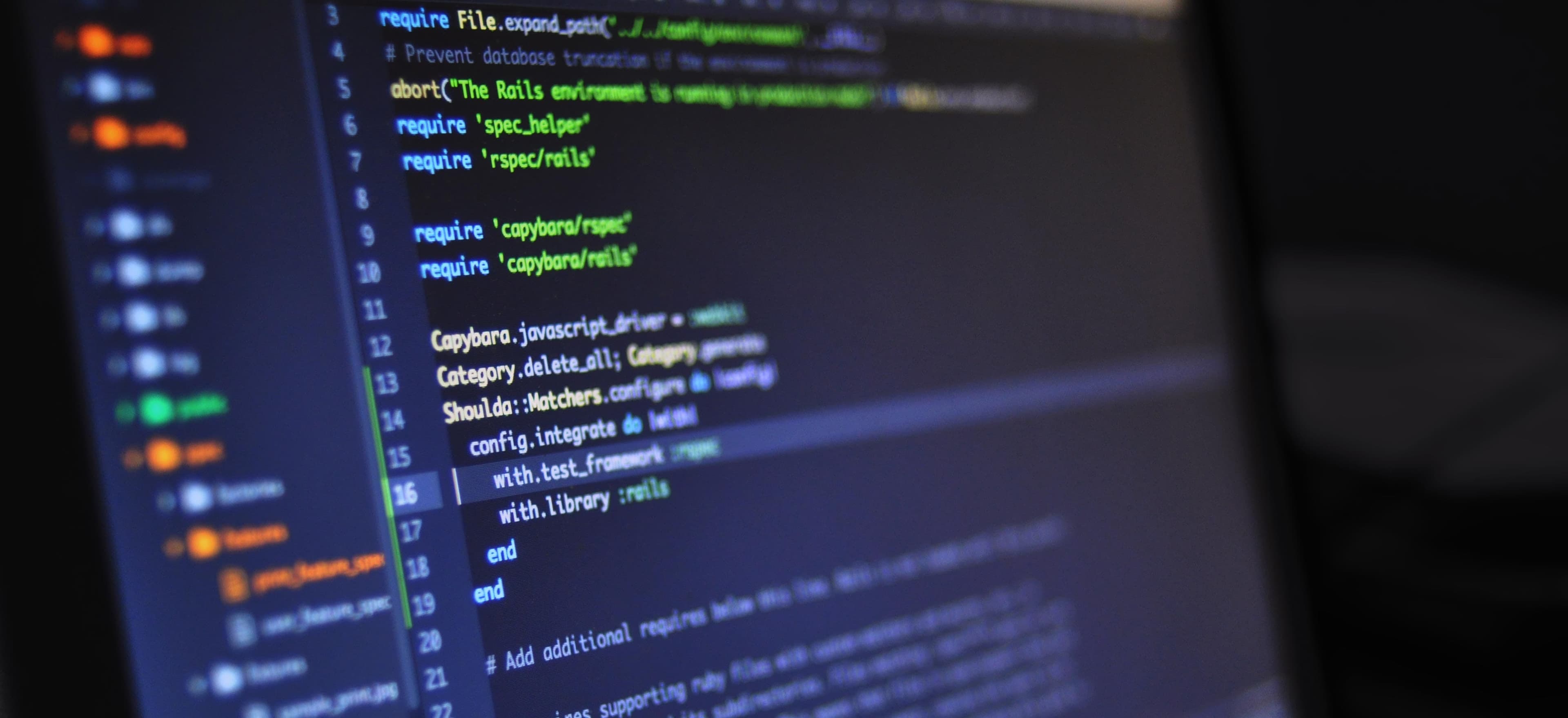
- Published on
In Java, concurrency allows for the execution of multiple threads in a program, enabling efficient utilization of system resources. However, as with any multi-threaded environment, issues like blocking can arise. Blocking occurs when a thread is prevented from performing further work until a certain condition is satisfied, which can lead to performance bottlenecks and other undesirable outcomes. This post delves into the concept of blocking in Java concurrency and explores strategies for effective management.
Understanding Blocking
At the core of Java concurrency, the synchronized
keyword is commonly used to prevent concurrent access to shared resources. For example, consider the following method:
public synchronized void incrementCounter() {
counter++;
}
In this scenario, if one thread is invoking incrementCounter()
, any other threads attempting to do the same will be blocked until the first thread completes execution. While synchronization is crucial for maintaining data integrity, it can also lead to blocking under certain circumstances, especially in high-contention scenarios where multiple threads are contending for the same lock.
Identifying Blocking Issues
To effectively manage blocking, it's essential to identify potential issues within the codebase. Tools such as VisualVM, YourKit, or Java Mission Control can assist in profiling and identifying performance bottlenecks related to blocking. Additionally, thread dump analysis can provide insights into which threads are in a blocked state and what resources they are contending for.
Dealing with Blocking: Strategies and Best Practices
1. Isolating Critical Sections:
One effective approach to minimize blocking is to isolate critical sections of code. By reducing the duration for which a lock is held, other threads can contend less for access. This can be achieved by identifying and isolating the specific portions of code that truly require synchronization, rather than keeping entire methods synchronized.
2. Using Locking Mechanisms:
In addition to intrinsic locks provided by the synchronized
keyword, Java's java.util.concurrent
package offers a range of explicit locking mechanisms such as ReentrantLock
and ReadWriteLock
. These provide finer-grained control over locking and can help mitigate issues related to excessive blocking.
Here's an example illustrating the use of ReentrantLock
:
private final ReentrantLock lock = new ReentrantLock();
public void performOperation() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
In this case, the ReentrantLock
allows for explicit locking and unlocking, giving more flexibility compared to intrinsic locks.
3. Employing Non-blocking Algorithms:
Non-blocking algorithms, such as the ones based on java.util.concurrent.atomic
package, offer alternatives to traditional lock-based synchronization. For instance, using AtomicInteger
instead of locking for simple counters can reduce contention and blocking.
Consider the following code snippet using AtomicInteger
:
private AtomicInteger counter = new AtomicInteger(0);
public void incrementCounter() {
counter.incrementAndGet();
}
The use of AtomicInteger
eliminates the need for explicit locking when performing atomic operations on the counter.
4. Asynchronous Programming:
In scenarios where blocking operations, such as I/O or network requests, are prevalent, leveraging asynchronous programming using CompletableFuture
or reactive libraries like Project Reactor and RxJava can mitigate the impact of blocking. Asynchronous programming allows threads to perform other tasks while waiting for asynchronous operations to complete, reducing overall blocking time.
Here's an example using CompletableFuture
:
public CompletableFuture<String> fetchFromRemoteService() {
return CompletableFuture.supplyAsync(() -> {
// Perform remote service call
return result;
});
}
By utilizing CompletableFuture
, the calling thread can proceed with other work while waiting for the result from the remote service.
5. Tuning Thread Pools:
In applications utilizing thread pools, tuning the pool parameters can significantly impact blocking issues. Adjusting the pool size, queue types (e.g., LinkedBlockingQueue
, SynchronousQueue
), and rejection policies can help alleviate potential blocking due to thread pool saturation.
Lessons Learned
Blocking in Java concurrency is a common challenge that requires careful consideration and management. By understanding the causes of blocking, employing effective strategies such as isolating critical sections, utilizing explicit locking mechanisms, incorporating non-blocking algorithms, embracing asynchronous programming, and tuning thread pools, developers can mitigate the impact of blocking on the performance and scalability of their concurrent applications.
Java provides a rich set of tools and techniques to address blocking issues, and mastering these approaches is crucial for building robust and efficient concurrent systems.
With a clear comprehension of blocking and the utilization of appropriate strategies, developers can ensure the smooth functioning of concurrent applications in the Java ecosystem.