Building Highly Scalable Applications in Vert.x
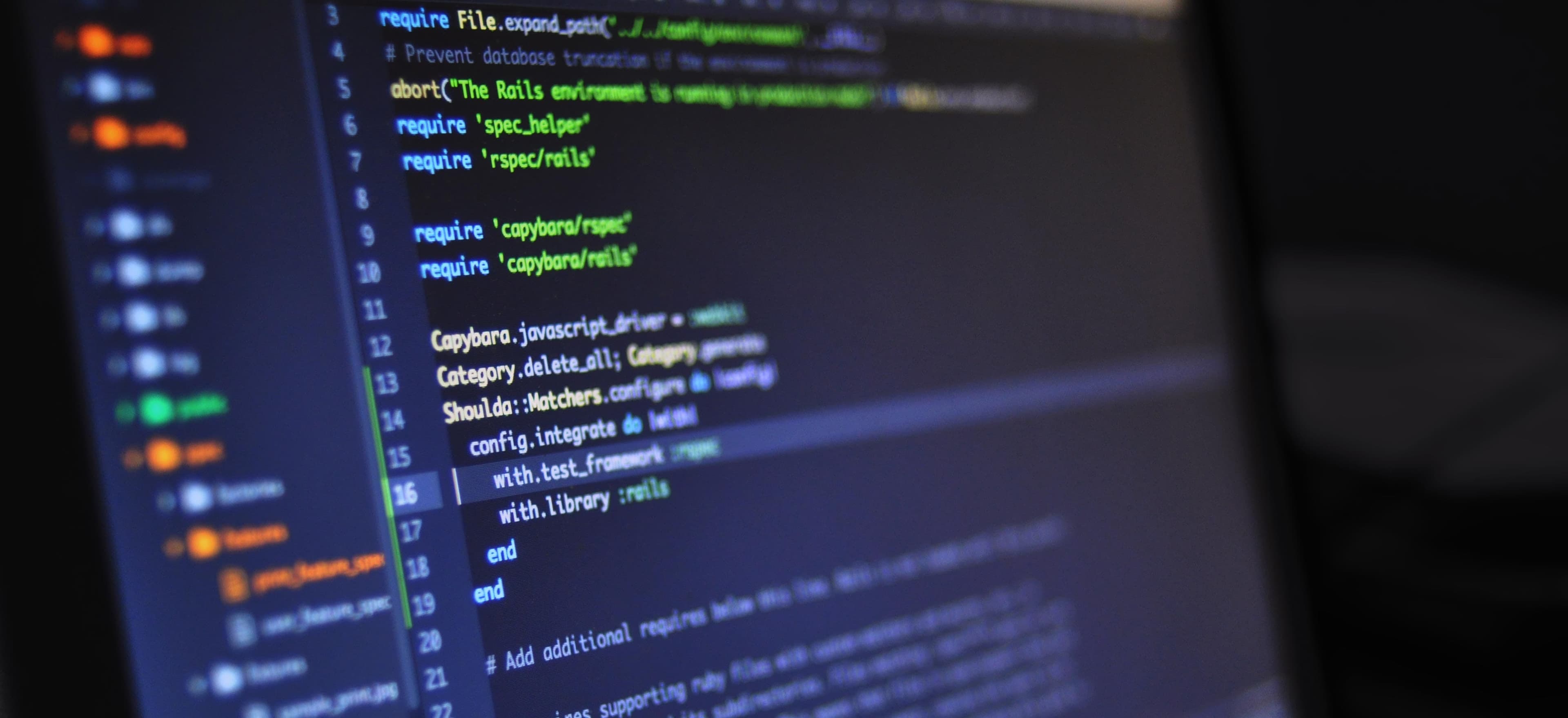
- Published on
Building Highly Scalable Applications in Vert.x
When it comes to building highly scalable applications in Java, developers often search for frameworks that provide the necessary tools and flexibility. Vert.x is one such framework that has gained popularity for its ability to build fast, scalable, and reactive applications. In this post, we will explore how Vert.x can be used to build highly scalable applications and discuss some best practices for achieving scalability.
What is Vert.x?
Vert.x is a lightweight, high-performance application framework for the JVM that’s designed for asynchronous and reactive programming. It’s polyglot, meaning it supports multiple languages such as Java, Kotlin, Groovy, and JavaScript, making it versatile for developers with diverse language preferences. One of the key features of Vert.x is its event-driven architecture, which allows for handling a large number of concurrent connections with minimal threads, making it ideal for building highly scalable applications.
Asynchronous and Reactive Programming
Vert.x's asynchronous and reactive programming model is fundamental to its scalability. By utilizing event loops and non-blocking I/O, Vert.x can efficiently handle a large number of concurrent requests without the need for a separate thread per connection. This approach is significantly more resource-efficient compared to traditional thread-based models, especially when dealing with scenarios like high-throughput web applications, IoT systems, or real-time data processing.
// Example of an asynchronous HTTP server using Vert.x
Router router = Router.router(vertx);
router.route("/api/resource")
.handler(routingContext -> {
// Simulate asynchronous operation
vertx.setTimer(100, id -> {
routingContext.response().end("Data Retrieved");
});
});
vertx.createHttpServer()
.requestHandler(router)
.listen(8080);
In this example, the HTTP server responds asynchronously, allowing the server to handle new requests without being blocked by the ongoing operation. This enables Vert.x to scale efficiently under heavy load.
Kinds of Scalability
Scalability can be both vertical and horizontal. Vertical scalability, also known as scaling up, involves adding more resources, such as CPU or memory, to a single instance of an application. Horizontal scalability, or scaling out, involves adding more instances of an application across multiple machines. Vert.x is well-suited for both forms of scalability.
Vertical Scalability
Vert.x's non-blocking and event-driven nature lends itself well to vertical scalability. By efficiently utilizing available resources and handling concurrent connections with minimal overhead, Vert.x applications can take advantage of increased CPU cores and memory in a single server setup.
Horizontal Scalability
When it comes to horizontal scalability, Vert.x provides seamless integration with distributed messaging systems such as Apache Kafka or RabbitMQ. This allows Vert.x applications to be deployed across multiple instances and communicate with each other through a message broker, enabling effortless horizontal scaling.
Best Practices for Building Highly Scalable Vert.x Applications
Embrace Asynchronous APIs
When working with Vert.x, it’s crucial to embrace asynchronous APIs for database access, I/O operations, and external service calls. Leveraging the asynchronous nature of Vert.x ensures that application threads aren’t blocked, enabling efficient handling of a large number of concurrent requests.
Reactive Composition
Utilize reactive programming paradigms in Vert.x by employing libraries like RxJava or Reactor. This enables developers to compose complex asynchronous operations in a readable and maintainable manner, leading to more scalable and responsive applications.
Effective Resource Management
Efficient resource management is crucial for scalability. Take advantage of Vert.x’s built-in support for managing resources such as connection pooling for databases, and ensure timely release of resources to prevent bottlenecks in high-traffic scenarios.
Microservices Architecture
Vert.x is well-suited for building microservices due to its lightweight nature and support for asynchronous communication between services. By adopting a microservices architecture, applications can be broken down into smaller, independent components that can be individually scaled based on demand.
Caching and In-Memory datastores
Incorporating caching mechanisms and in-memory data stores such as Redis can significantly enhance the performance and scalability of Vert.x applications by reducing the load on backend systems and accelerating data access.
Key Takeaways
In conclusion, Vert.x provides a powerful foundation for building highly scalable applications in Java. Its asynchronous and reactive programming model, support for vertical and horizontal scalability, and best practices for development make it an excellent choice for tackling scalability challenges. By leveraging Vert.x's capabilities effectively, developers can build applications that are not only highly scalable but also performant and responsive under varying workloads.
Give Vert.x a try for your next project, and experience the seamless scalability it offers for modern, high-performance applications!
If you're interested in diving deeper into Vert.x, you can explore the official Vert.x documentation for comprehensive insights into its features and capabilities.