Getting Started with JavaSlang: Handling Null Values
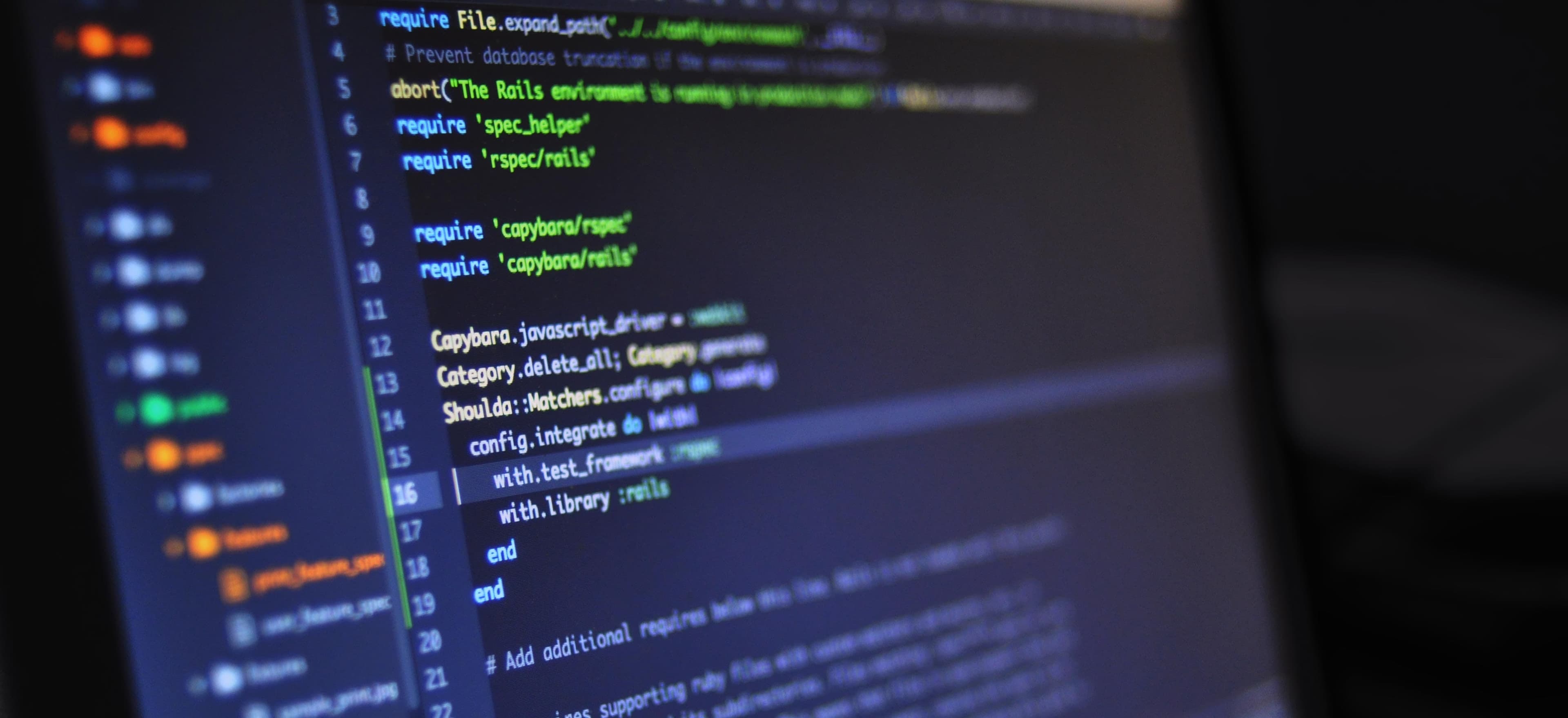
- Published on
A Guide to Null-Safe Programming in Java with JavaSlang
Handling null values in Java has always been error-prone and a source of numerous bugs. JavaSlang, a functional programming library for Java, offers powerful features to work with null values safely. In this guide, we will explore how to leverage JavaSlang to handle null values effectively, leading to more robust and maintainable code.
What are Null Values and the Challenges They Pose?
In Java, null
is a special value that can be assigned to any reference type. It indicates the absence of a value. However, dealing with nulls can often lead to NullPointerExceptions
, causing application crashes and unexpected behavior. Traditional approaches like null checks (if (variable != null)
) can clutter the code, reducing readability and maintainability.
Enter JavaSlang
JavaSlang provides functional programming features to Java, including a rich set of immutable, functional data types and null-safe operations. One of its key components is Option
, which represents an optional value that can be either Some
containing a value or None
representing the absence of a value. Let's dive into how JavaSlang's Option
can help us handle null values effectively.
Using Option for Null-Safe Operations
To start using JavaSlang, you can add the following Maven dependency to your project:
<dependency>
<groupId>io.vavr</groupId>
<artifactId>vavr</artifactId>
<version>0.10.0</version>
</dependency>
Now, let's see an example of how Option
can be used to handle null values safely:
import io.vavr.control.Option;
public class NullHandlingExample {
public static void main(String[] args) {
String nullableValue = possiblyNullableMethod();
Option<String> option = Option.ofNullable(nullableValue);
String result = option.getOrElse("Default Value");
System.out.println(result);
}
private static String possiblyNullableMethod() {
return Math.random() < 0.5 ? "NonNullValue" : null;
}
}
In this example, we first create an Option
using Option.ofNullable(nullableValue)
, where nullableValue
may be null
. We then retrieve the value using getOrElse
, providing a default value in case the original value is None
. This approach allows us to safely handle null values without explicit null checks.
An Alternative Approach: Try for Exception Handling
In addition to Option
, JavaSlang provides the Try
type for handling exceptions and errors in a functional way. It represents a computation that may either result in an exception (Failure
) or a successful value (Success
). Let's take a look at how Try
can be used to handle exceptions gracefully:
import io.vavr.control.Try;
public class ExceptionHandlingExample {
public static void main(String[] args) {
String filePath = "example.txt";
Try<String> fileContent = Try.of(() -> readFromFile(filePath));
String result = fileContent.getOrElse("Default Content");
System.out.println(result);
}
private static String readFromFile(String filePath) throws IOException {
// Read content from file
return "File Content";
}
}
In this example, we use Try.of(() -> readFromFile(filePath))
to capture any exceptions thrown by readFromFile
and encapsulate them as a Failure
. We then retrieve the result using getOrElse
, handling the case where an exception occurs. This approach makes error handling more explicit and composable, enhancing the robustness of our code.
Chaining Operations with Option and Try
One of the powerful features of JavaSlang is the ability to chain operations fluently while preserving null-safety and exception handling. Let's consider a scenario where we need to perform a series of operations, each of which may return null
or throw an exception. Here's how we can achieve this using Option
and Try
:
import io.vavr.control.Option;
import io.vavr.control.Try;
public class ChainingOperationsExample {
public static void main(String[] args) {
String filePath = "example.txt";
String result = Option.of(readFromFile(filePath))
.map(content -> content.toUpperCase())
.getOrElse("Default Content");
System.out.println(result);
}
private static String readFromFile(String filePath) {
return Try.of(() -> {
// Read content from file
return "File Content";
})
.getOrElse(() -> null);
}
}
In this example, we first read the file content using Try
and then convert it to uppercase using map
from Option
. We handle null values and exceptions gracefully within the chain, resulting in concise and robust code.
To Wrap Things Up
JavaSlang provides powerful tools such as Option
and Try
to handle null values and exceptions in a functional and concise manner. By leveraging these constructs, developers can write more robust and maintainable code while reducing the risk of NullPointerExceptions
and errors. Embracing null-safe operations and exception handling through JavaSlang can significantly improve the reliability and quality of Java applications.
In this guide, we've scratched the surface of JavaSlang's capabilities for null-safe programming. As you dive deeper into functional programming with JavaSlang, exploring its comprehensive features and embracing its best practices will empower you to write cleaner, safer, and more efficient code.
Start leveraging JavaSlang for null-safe programming today, and experience a paradigm shift in how you handle null values in Java!
Checkout our other articles