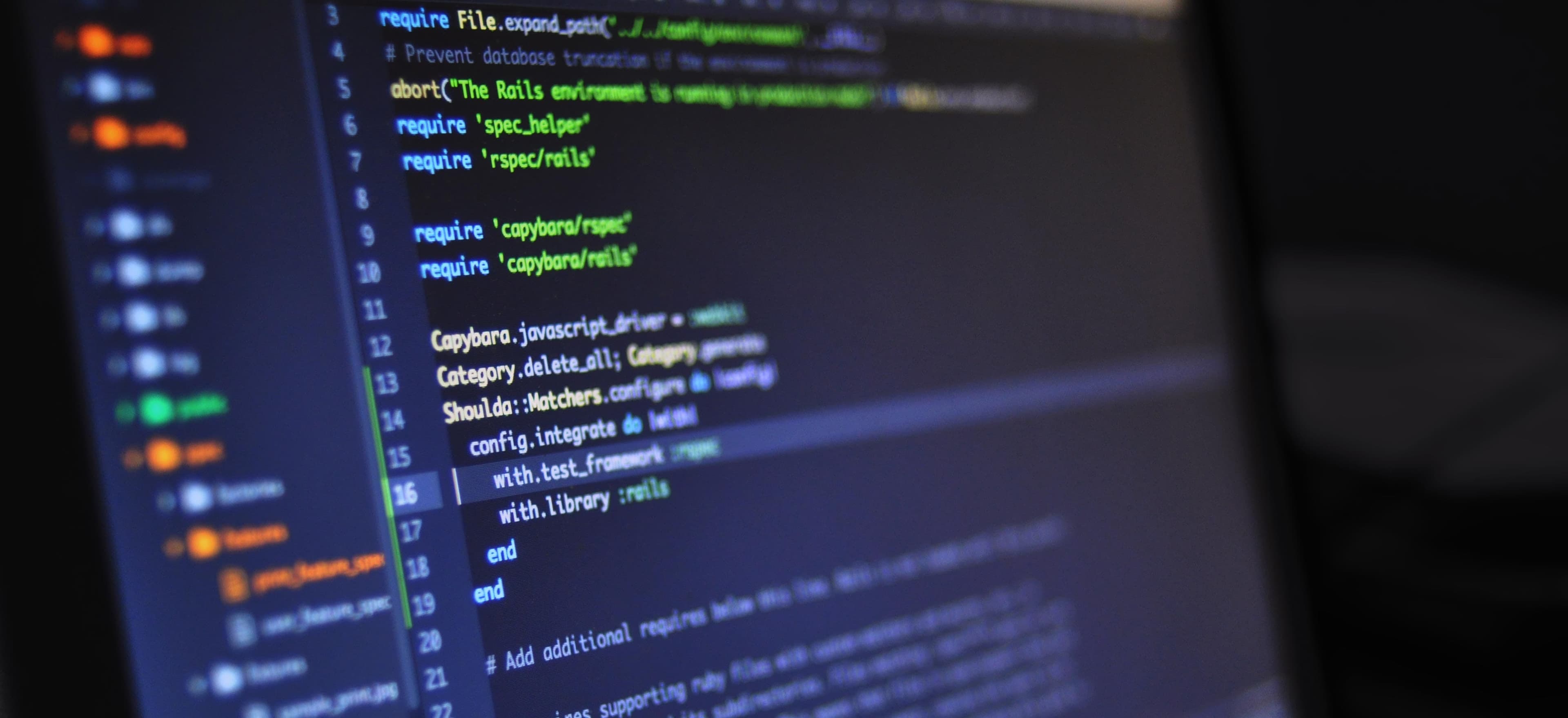
- Published on
The Challenge of Implementing SSO for Seamless Cloud Access
Single Sign-On (SSO) is a crucial identity and access management feature that allows users to access multiple applications with a single set of credentials. In the context of cloud computing, SSO becomes even more significant, as it provides seamless access to various cloud services without the need for multiple logins. In this article, we will explore the challenges and considerations involved in implementing SSO for seamless cloud access and discuss how Java can be used to address these challenges.
The Importance of SSO in Cloud Computing
In a cloud environment, users interact with a multitude of services, such as email, document storage, collaboration tools, and more. Each of these services typically requires separate authentication, leading to password fatigue and security risks. SSO simplifies this process by allowing users to authenticate once and access multiple services without the need to re-enter credentials.
Challenges in Implementing SSO for Cloud Access
1. Security Concerns:
- SSO introduces a single point of failure, and if compromised, all connected services become vulnerable. This necessitates robust security measures to protect user credentials and authentication tokens.
2. Integration with Multiple Services:
- Cloud environments often consist of an assortment of services from different providers, each with its own authentication mechanisms. Integrating SSO with these diverse services can be complex.
3. User Experience:
- The SSO implementation should seamlessly integrate into the user experience, ensuring that users can effortlessly navigate between various cloud services without interruptions.
Implementing SSO with Java
Java, with its strong security features and wide array of libraries, provides a robust platform for implementing SSO for cloud access.
Using OAuth 2.0 for SSO
OAuth 2.0 is a widely adopted authorization framework that enables SSO and secure data access. It allows users to grant limited access to their resources on one site to another site without having to expose their credentials. Java provides several libraries for implementing OAuth 2.0, such as Spring Security OAuth and Apache Oltu.
Below is an example of how OAuth 2.0 can be implemented with Spring Security OAuth in Java:
// Code snippet using Spring Security OAuth for SSO
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("client-id")
.secret("client-secret")
.authorizedGrantTypes("authorization_code")
.scopes("read", "write")
.redirectUris("http://localhost:8081/login/oauth2/code/custom");
}
// Other OAuth 2.0 configurations
}
In this example, the AuthorizationServerConfig class configures the client details for OAuth 2.0, including client id, client secret, grant types, and scopes.
Integrating with SAML for SSO
Security Assertion Markup Language (SAML) is another popular choice for implementing SSO. It enables secure SSO across different domains or organizations. Java provides libraries like OpenSAML for implementing SAML-based SSO.
Here's a brief example of how SAML can be integrated using OpenSAML in Java:
// Code snippet illustrating SAML integration for SSO
public class SAMLIntegration {
public void initiateSSO(String serviceProviderURL, String identityProviderURL) {
// SAML initiation logic
}
// Other SAML integration methods
}
Managing User Sessions
In a cloud environment, managing user sessions becomes crucial for seamless SSO. Java provides features like session management in Spring Security that can be utilized to maintain user sessions across multiple cloud services.
The Last Word
Implementing SSO for seamless cloud access presents significant challenges, including security concerns, integration with multiple services, and maintaining a seamless user experience. Java, with its robust security features and extensive libraries, provides a solid foundation for addressing these challenges. By leveraging technologies like OAuth 2.0, SAML, and session management features, Java developers can build secure and seamless SSO solutions for cloud environments.
SSO not only simplifies the user experience but also enhances the overall security posture of cloud-based systems. As cloud computing continues to proliferate, the need for effective SSO implementations in Java will only become more critical.
To dive deeper into SSO implementations in Java, check out the official documentation and explore popular Java frameworks like Spring Security and Apache Shiro.