Optimizing Database Performance in MyBatis with Spring
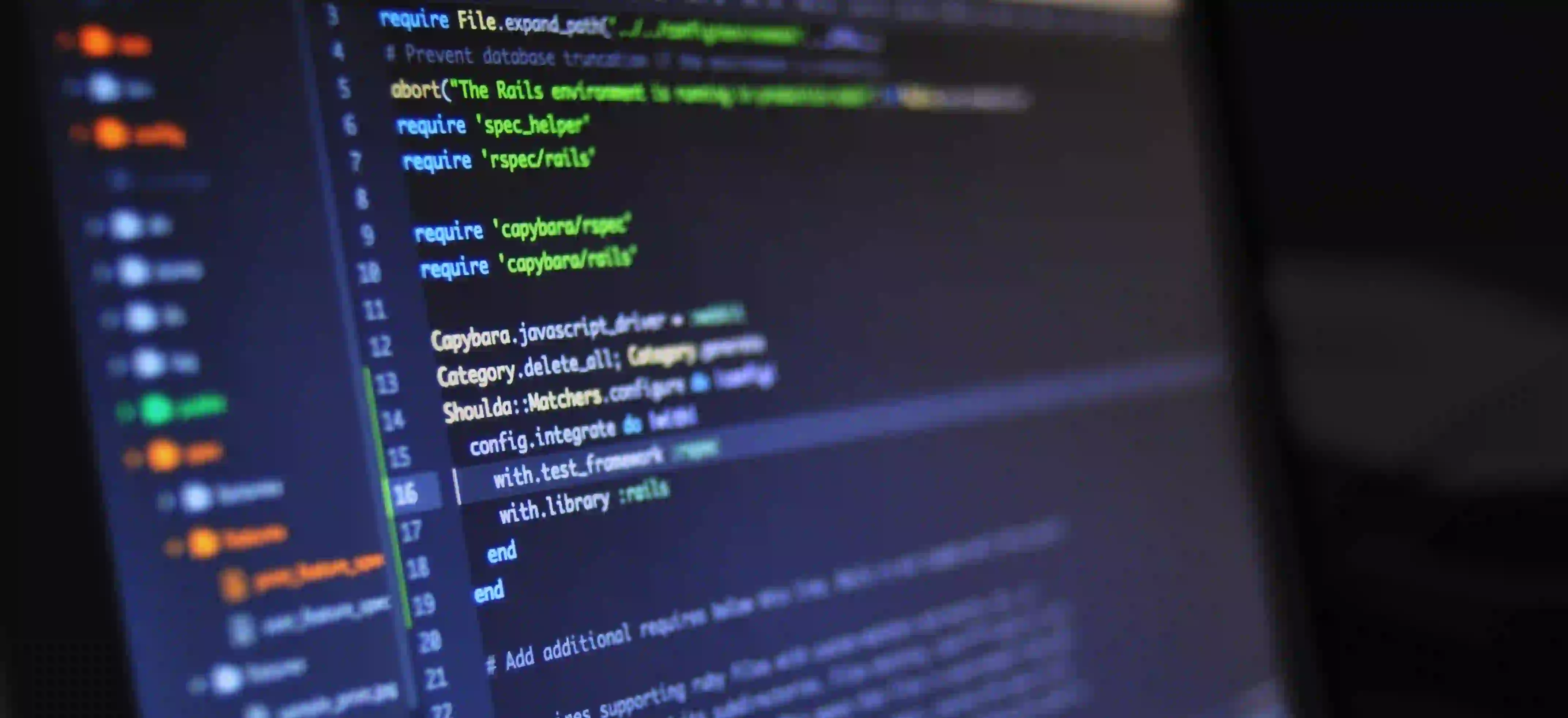
Optimizing Database Performance in MyBatis with Spring
When it comes to building efficient and performant Java applications that interact with databases, developers often turn to MyBatis, a popular persistence framework. MyBatis provides a way to map Java methods to SQL statements using annotations or XML configuration. When combined with the Spring framework, MyBatis becomes a powerful tool for building scalable and high-performance database interactions.
In this article, we will explore some best practices and techniques for optimizing database performance when using MyBatis with Spring. We will cover topics such as caching, batch processing, and tuning SQL queries to improve overall application performance.
Setting Up MyBatis with Spring
Before we dive into performance optimization, let’s briefly review how to set up MyBatis with Spring. First, you need to include the necessary dependencies in your pom.xml
if you are using Maven:
<dependencies>
<!-- MyBatis and Spring dependencies -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.8</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.5</version>
</dependency>
<!-- Other dependencies -->
</dependencies>
If you are using Gradle, you can include the dependencies in your build.gradle
file:
dependencies {
// MyBatis and Spring dependencies
implementation 'org.mybatis:mybatis:3.5.8'
implementation 'org.mybatis.spring.boot:mybatis-spring-boot-starter:2.1.5'
// Other dependencies
}
Next, you need to configure MyBatis in your Spring application, typically by creating a SqlSessionFactory
bean and a MapperScannerConfigurer
. You can refer to the official MyBatis-Spring documentation for detailed configuration steps.
Leveraging Caching in MyBatis
One of the most effective ways to improve database performance is through caching. MyBatis provides caching mechanisms that can significantly reduce the number of trips to the database, especially for frequently accessed data.
First-level Cache
MyBatis comes with a first-level cache, which is the default cache and is associated with a SqlSession. This cache is effective within the scope of a single SqlSession, meaning if you query the same data within the same SqlSession, MyBatis will return the cached result without hitting the database.
// Example of leveraging first-level cache
SqlSession session = sqlSessionFactory.openSession();
try {
User user1 = session.selectOne("getUser", 1);
User user2 = session.selectOne("getUser", 1); // Retrieves from cache
// Both user1 and user2 will be the same object without additional database queries
} finally {
session.close();
}
Second-level Cache
In addition to the first-level cache, MyBatis also supports a second-level cache, which is shared across SqlSession instances within the same application. This can be particularly useful when you have multiple SqlSession instances and want to share cached data between them.
To enable the second-level cache, you need to configure it in your MyBatis configuration file (usually mybatis-config.xml
) and also enable caching for specific mapper XML files.
<!-- Enabling second-level cache in mybatis-config.xml -->
<settings>
<setting name="cacheEnabled" value="true"/>
</settings>
<!-- Enabling cache for specific mapper XML -->
<mapper namespace="com.example.UserMapper">
<cache/>
</mapper>
By caching query results, MyBatis avoids the overhead of repetitive database calls, leading to improved performance and reduced load on the database.
For more in-depth information on configuring caching in MyBatis, refer to the official MyBatis documentation on caching.
Batch Processing for Efficient Writes
When dealing with large datasets or performing bulk insert/update operations, MyBatis provides support for batch processing, which can significantly improve write performance by reducing the number of round trips to the database.
Using Batch Inserts
MyBatis offers a foreach
element that allows you to perform bulk insert operations efficiently. This is accomplished by grouping multiple insert statements into a single batch, reducing the overhead of executing individual queries.
<!-- Example of batch insert with MyBatis -->
<insert id="insertUsers" parameterType="list">
<foreach collection="users" item="user" separator=";">
INSERT INTO users (id, name) VALUES (#{user.id}, #{user.name})
</foreach>
</insert>
In the above example, the foreach
element iterates over a list of users and generates a batch of insert statements. When executed, this can be more efficient than individual insert statements, especially for inserting a large number of records.
Using Batch Updates
Similar to batch inserts, MyBatis allows for batch update operations using the foreach
element. When updating multiple records with similar changes, batching the update statements can greatly reduce the overall update time.
<!-- Example of batch update with MyBatis -->
<update id="updateUsers" parameterType="list">
<foreach collection="users" item="user" separator=";">
UPDATE users SET name = #{user.name} WHERE id = #{user.id}
</foreach>
</update>
By leveraging batch processing for bulk inserts and updates, you can minimize the overhead of database communication and achieve significant performance improvements.
For more details on batch processing in MyBatis, check the official MyBatis documentation on batch processing.
Tuning SQL Queries for Performance
Apart from leveraging caching and batch processing, optimizing the SQL queries themselves can have a profound impact on database performance. By writing efficient and optimized SQL queries, you can minimize resource consumption and maximize database throughput.
Indexing for Query Optimization
Properly indexing your database tables can significantly improve query performance, especially for tables with a large number of records. Indexes help the database engine locate and retrieve specific rows quickly, thereby reducing the time taken to execute queries.
You can analyze query execution plans and identify potential candidates for indexing using database management tools like pgAdmin for PostgreSQL or MySQL Workbench for MySQL.
Here’s an example of adding an index to a table using MyBatis migration script:
<changeSet author="you" id="add-index">
<addIndex tableName="users" indexName="idx_name" unique="false" schemaName="public">
<column name="name"/>
</addIndex>
</changeSet>
Indexing relevant columns based on query patterns can lead to substantial improvements in query execution times.
Using Parameterized Queries
Another crucial aspect of SQL query optimization is the use of parameterized queries to prevent SQL injection and enhance query plan caching. Parameterized queries allow the database engine to reuse query execution plans, reducing the overhead of query parsing and optimization.
Here’s an example of a parameterized query using MyBatis:
<!-- Example of parameterized query with MyBatis -->
<select id="getUserById" parameterType="java.lang.Long" resultType="User">
SELECT * FROM users WHERE id = #{id}
</select>
By using parameterized queries, you not only enhance security but also contribute to improved query performance through plan caching and reuse.
Monitoring and Profiling Database Queries
It's essential to monitor and profile database queries to identify bottlenecks and optimize the performance of your MyBatis-Spring application. Leveraging tools like Spring Boot Actuator, which provides metrics and insights into database interactions, can aid in monitoring and identifying areas of improvement.
By integrating Spring Boot Actuator into your application, you can gain visibility into database query execution times, number of database connections, and other critical metrics, enabling you to pinpoint performance issues and optimize accordingly.
Closing Remarks
Optimizing database performance in MyBatis with Spring involves a combination of leveraging caching mechanisms, employing batch processing for efficient writes, tuning SQL queries for optimal execution, and monitoring database interactions. By incorporating these best practices and techniques into your MyBatis-Spring application, you can achieve significant improvements in performance and scalability.
In this article, we've explored various approaches to enhance database performance, but it's important to note that the effectiveness of these techniques may vary based on specific use cases and database systems. It's crucial to analyze and benchmark the impact of these optimizations on your application’s performance and adjust them accordingly.
By continuously fine-tuning and optimizing database interactions, you can ensure that your MyBatis-Spring application delivers exceptional performance and responsiveness, contributing to a seamless user experience and efficient utilization of resources.
Remember, database performance optimization is an ongoing process, and staying informed about the latest best practices and tools is key to maintaining superior application performance.