Converting Object Graphs to XML/JSON: Handling Partial Models
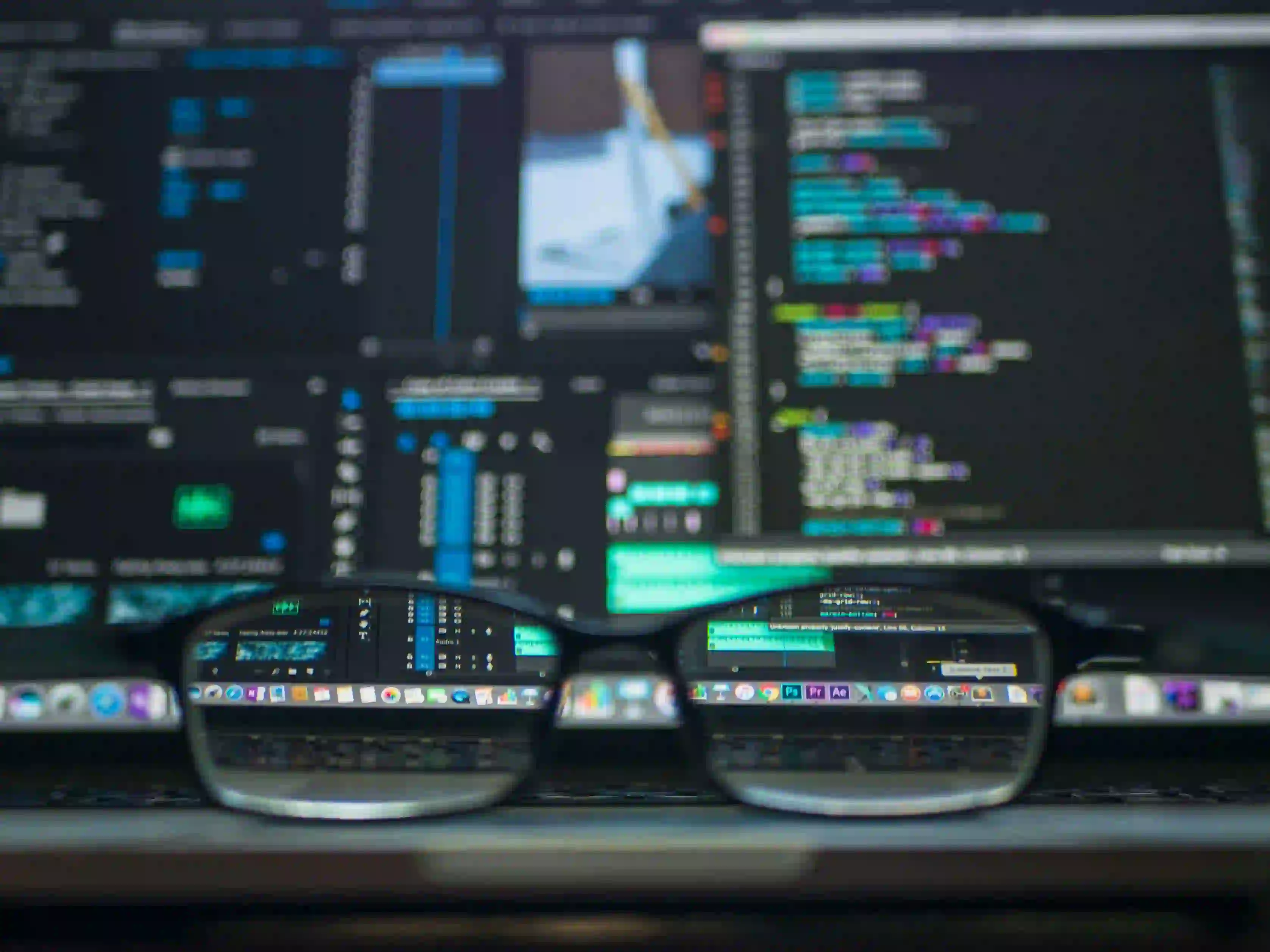
Converting Object Graphs to XML/JSON: Handling Partial Models.
When working with Java, you may often encounter the need to convert complex object graphs to XML or JSON. This is a common requirement, especially when dealing with data serialization for web services, file persistence, or inter-process communication. However, it becomes even more challenging when you need to handle partial models, where only certain parts of the object graph should be converted.
In this blog post, we'll explore how to tackle this challenge in Java by leveraging various libraries and best practices. We'll discuss the concept of partial models, explore different approaches to handle them during conversion, and delve into practical examples using popular Java libraries such as Jackson and JAXB.
Understanding Partial Models
A partial model refers to a subset of an object graph that needs to be converted into XML or JSON. This can occur for various reasons, such as:
- Privacy concerns: When sensitive data should not be exposed.
- Performance optimization: Only transmitting necessary data.
- View-specific requirements: Customizing output for different use-cases.
Handling partial models involves selecting specific fields or sub-objects within the object graph for serialization while ignoring others.
Approach with Jackson Library
Jackson is a widely-used Java library for JSON serialization and deserialization. It provides various annotations and configuration options to control the serialization process.
Using Jackson's ObjectMapper
// Create ObjectMapper instance
ObjectMapper objectMapper = new ObjectMapper();
// Define a custom mix-in to handle partial serialization
class PartialModelMixin {
@JsonIgnore
private String sensitiveField;
}
// Register the mix-in with the ObjectMapper
objectMapper.addMixIn(YourClass.class, PartialModelMixin.class);
// Serialize the object to JSON
String json = objectMapper.writeValueAsString(yourObject);
In the above example, we create a custom mix-in class PartialModelMixin
with annotations like @JsonIgnore
to exclude specific fields from serialization. We then register this mix-in with the ObjectMapper
to handle partial models during serialization.
Using JSON Views
Jackson also provides a concept called "JSON Views" to support different perspectives of the same data model.
// Define a view interface
public class Views {
public interface Public {}
public interface Internal extends Public {}
}
// Annotate fields with view-specific annotations
public class YourClass {
@JsonView(Views.Public.class)
private String publicField;
@JsonView(Views.Internal.class)
private String internalField;
}
// Serialize the object using a specific view
String json = objectMapper.writerWithView(Views.Public.class).writeValueAsString(yourObject);
By using @JsonView
annotations, we can define different views within our data model and selectively serialize fields based on these views.
Dealing with Partial Models Using JAXB
JAXB is the Java Architecture for XML Binding, which provides a convenient way to map Java classes to XML representations.
Using XmlAccessType.NONE
// Define the class with XmlAccessType.NONE
@XmlAccessorType(XmlAccessType.NONE)
public class YourClass {
@XmlElement
private String publicField;
@XmlElement
private String internalField;
}
In this example, we set XmlAccessType.NONE
to exclude automatic mapping of fields and then explicitly specify which fields should be included for XML serialization using @XmlElement
.
Best Practices for Handling Partial Models
When working with partial models, it's essential to follow best practices to ensure maintainability and readability of the codebase.
- Consistent Naming Conventions: Use intuitive names for views or mix-in classes to indicate their purpose clearly.
- Document the Views: Provide documentation about the purpose of each view and the intended use-cases.
- Avoid Overcomplicating Views: Keep the views simple and focused on specific use-cases to prevent confusion.
The Bottom Line
Handling partial models during the conversion of object graphs to XML or JSON is a common requirement in Java development. By leveraging libraries such as Jackson and JAXB, and following best practices, developers can effectively manage and customize the serialization process based on the specific needs of their applications.
In this blog post, we've explored various techniques for handling partial models and provided practical examples to demonstrate the implementation with Jackson and JAXB. Understanding these concepts and applying them judiciously can significantly enhance the flexibility and performance of your Java applications when dealing with complex object graphs and serialization requirements.