Challenges in Non-Sequential AI Programming
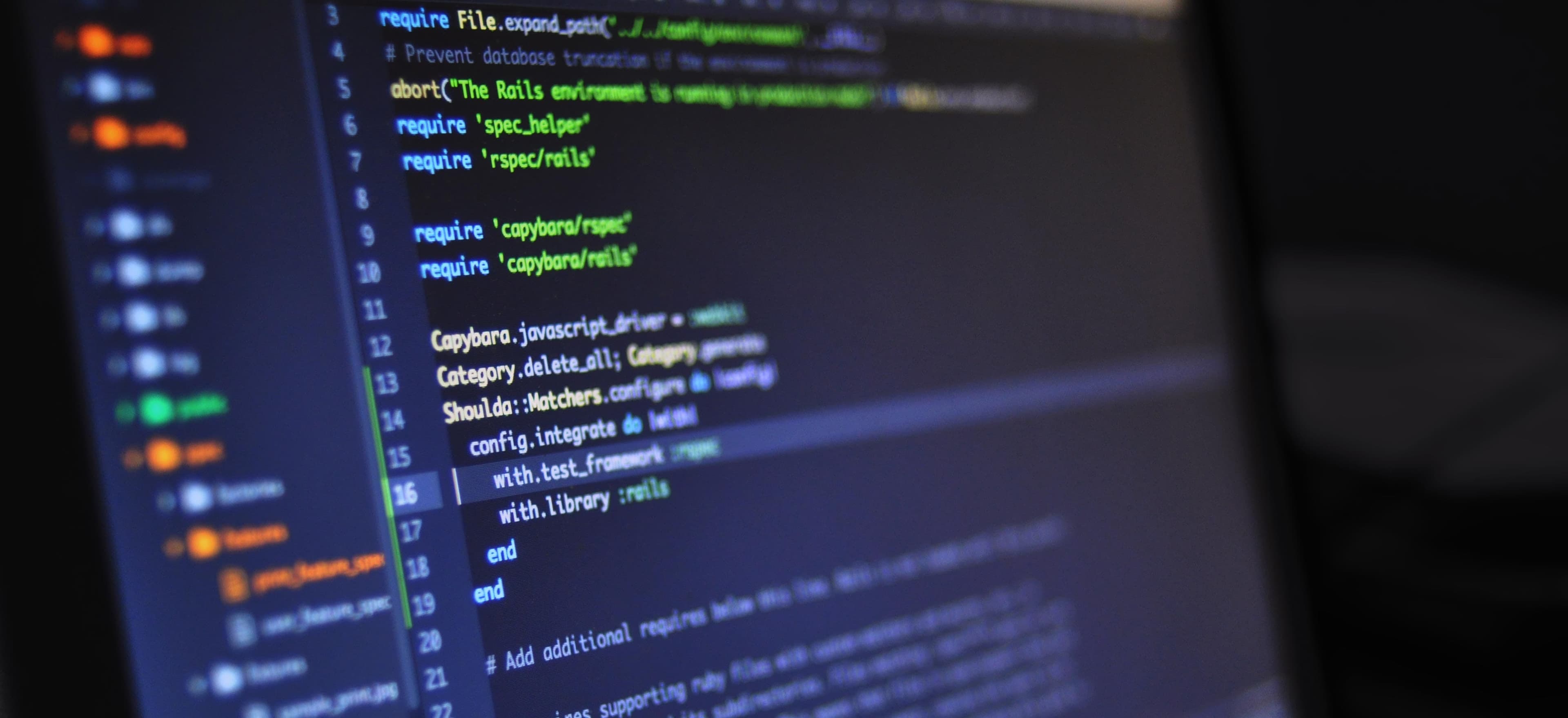
- Published on
Mastering Non-Sequential AI Programming in Java
Artificial Intelligence (AI) has made significant advancements in recent years, revolutionizing various industries. With Java being a popular programming language, it has become crucial to understand and master non-sequential AI programming in Java. Non-sequential AI involves creating intelligent systems that can process information in parallel, making it a challenging yet exciting field to explore.
Understanding Non-Sequential AI Programming
Non-sequential AI programming focuses on developing AI systems that can perform multiple tasks simultaneously. Unlike traditional sequential programming, where instructions are executed one after the other, non-sequential AI leverages parallel processing to handle complex tasks efficiently.
In Java, non-sequential AI programming often involves leveraging libraries and frameworks that provide support for parallel processing, such as the java.util.concurrent
package and the Fork/Join framework. These tools enable developers to create AI systems that can take advantage of multi-core processors and distributed computing environments.
Dealing with Asynchronous Events
One of the key challenges in non-sequential AI programming is handling asynchronous events. In traditional sequential programming, developers are accustomed to writing code that follows a linear execution flow. However, in non-sequential AI programming, events can occur simultaneously and independently, requiring careful consideration of concurrency and synchronization.
Java provides powerful tools for managing asynchronous events, such as the CompletableFuture
class. By utilizing CompletableFuture
and its methods, developers can design AI systems that can efficiently handle asynchronous tasks and coordinate their outcomes.
Example:
import java.util.concurrent.CompletableFuture;
public class AsyncEventExample {
public static void main(String[] args) {
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> performTask("Task 1"));
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> performTask("Task 2"));
future1.thenAcceptBoth(future2, (result1, result2) -> {
System.out.println("Result of Task 1: " + result1);
System.out.println("Result of Task 2: " + result2);
});
}
private static String performTask(String taskName) {
// Simulating a time-consuming task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Completed " + taskName;
}
}
In this example, two tasks (Task 1
and Task 2
) are executed asynchronously, and their results are combined using the thenAcceptBoth
method. This demonstrates how Java's CompletableFuture
can be used to manage and coordinate asynchronous events in non-sequential AI programming.
Leveraging Parallelism for Performance
Another significant aspect of non-sequential AI programming is leveraging parallelism to improve performance. Parallel processing allows AI systems to divide complex tasks into smaller sub-tasks that can be executed concurrently, leading to faster execution times and efficient resource utilization.
In Java, the Fork/Join framework plays a vital role in enabling parallelism for AI applications. By using the ForkJoinPool
and related constructs, developers can parallelize computationally intensive operations, enabling AI systems to benefit from multi-core architectures and achieve significant performance gains.
Example:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ParallelismExample {
public static void main(String[] args) {
ForkJoinPool forkJoinPool = new ForkJoinPool();
long[] data = { 3, 5, 2, 8, 6, 9, 4, 1 };
ParallelSumCalculator task = new ParallelSumCalculator(data, 0, data.length);
long result = forkJoinPool.invoke(task);
System.out.println("Sum: " + result);
}
static class ParallelSumCalculator extends RecursiveTask<Long> {
private final long[] data;
private final int start;
private final int end;
public ParallelSumCalculator(long[] data, int start, int end) {
this.data = data;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= 2) {
long sum = 0;
for (int i = start; i < end; i++) {
sum += data[i];
}
return sum;
} else {
int mid = start + (end - start) / 2;
ParallelSumCalculator leftTask = new ParallelSumCalculator(data, start, mid);
ParallelSumCalculator rightTask = new ParallelSumCalculator(data, mid, end);
leftTask.fork();
long rightResult = rightTask.compute();
long leftResult = leftTask.join();
return leftResult + rightResult;
}
}
}
}
In this example, a parallel sum calculator is implemented using the Fork/Join framework to demonstrate how parallelism can be harnessed for improved performance in non-sequential AI programming.
To Wrap Things Up
Mastering non-sequential AI programming in Java requires a deep understanding of parallel processing, asynchronous event handling, and the effective utilization of concurrency constructs. By leveraging the unique features and tools provided by Java, developers can build intelligent AI systems that can efficiently process information in parallel, paving the way for groundbreaking advancements in AI-driven applications.
As the field of AI continues to evolve, mastering non-sequential AI programming in Java will undoubtedly become a valuable skill, empowering developers to tackle complex AI challenges and drive innovation in various domains.
In conclusion, the future of AI is non-sequential, and Java provides the necessary tools and capabilities to thrive in this exciting frontier.
For further insights into the world of non-sequential AI programming and Java's role in it, you can explore the following resources:
- Java Concurrency in Practice - A comprehensive book on mastering concurrency and parallelism in Java.
- The Official Java Documentation on Concurrency - In-depth documentation on Java's concurrency utilities and best practices.
Stay curious, keep coding, and embrace the non-sequential future of AI programming with Java!