Streamlining Java Interactions with Solana Instruction Data
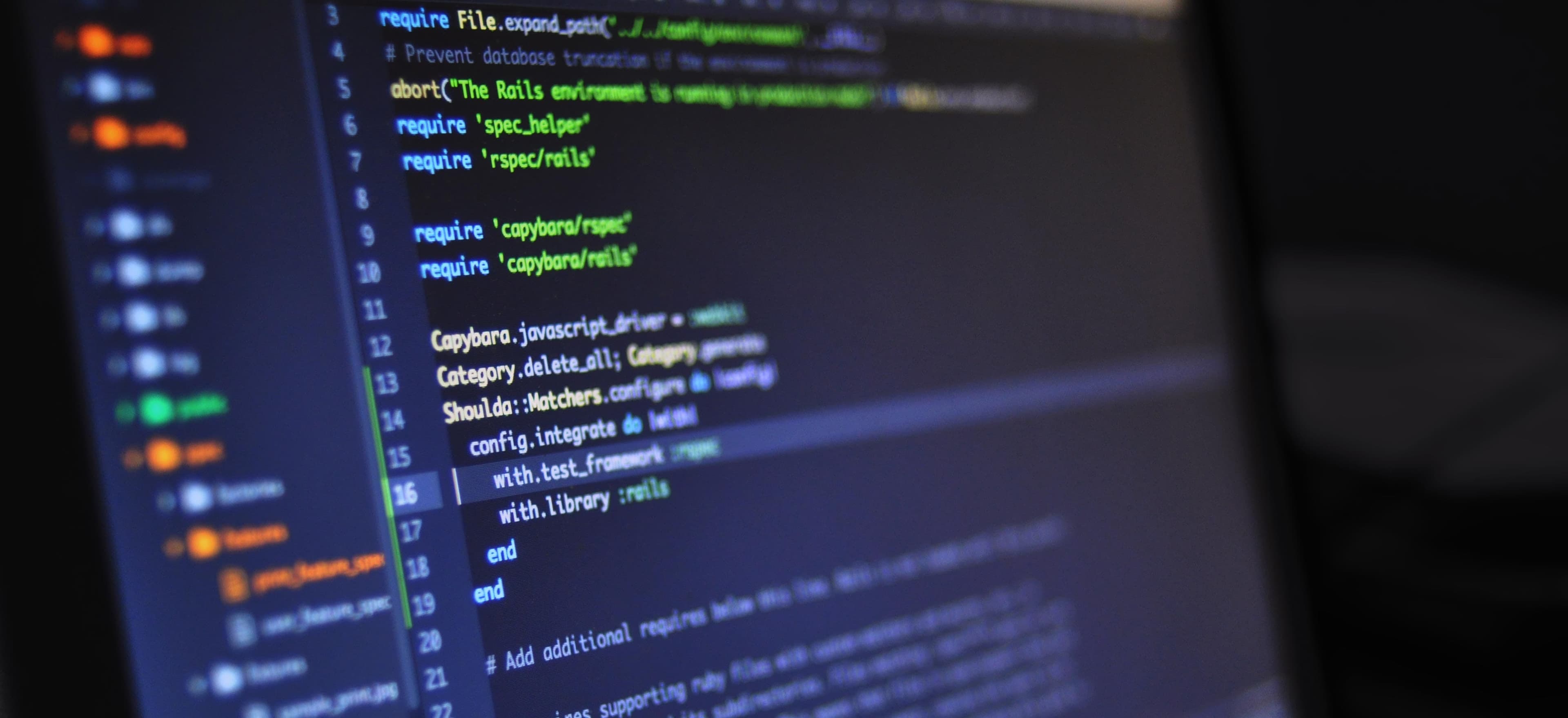
- Published on
Streamlining Java Interactions with Solana Instruction Data
As blockchain technology continues to revolutionize various sectors, developers are increasingly turning to Solana for its high throughput and low transaction costs. Solana's robust architecture allows it to execute a staggering number of transactions per second, making it a popular choice among developers. However, interacting with Solana, especially for custom instructions, can be challenging. In this blog post, we'll explore how to streamline Java interactions with Solana Instruction Data, building on concepts introduced in the article "Sending Custom Instructions Using Solana Instruction Data" available at infinitejs.com.
Understanding Solana Instruction Data
Before diving into code, it’s vital to understand the significance of instruction data in Solana. In essence, instruction data allows developers to send specific commands to the Solana blockchain. These commands are crucial for executing operations such as creating new accounts, transferring tokens, or interacting with smart contracts (often referred to as programs in Solana).
When you craft a transaction in Solana, you're packaging this instruction data along with other parameters, such as accounts involved and user signatures. This combination is sent to the network for processing. Java, being a robust and versatile programming language, can effectively manage these complexities, allowing for smoother interactions with Solana.
Setting Up the Java Environment
To begin, ensure you have the following set up in your Java environment:
- Java Development Kit (JDK): Make sure you have JDK 11 or later installed on your system.
- Maven: For managing dependencies and compiling our project.
- Solana SDK for Java: While Solana doesn’t have an official Java SDK, you can use libraries like
solana-web3j
which provides the necessary tools to interact with Solana via Java.
To include solana-web3j
in your project, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.nachain</groupId>
<artifactId>solana-web3j</artifactId>
<version>1.0.0</version> <!-- Check for the latest version -->
</dependency>
Crafting Custom Instructions for Solana
Creating a Connection to the Solana Cluster
The first step to interact with Solana is establishing a connection to a cluster. You can connect to either the mainnet, testnet, or devnet, depending on your needs.
import org.web3j.protocol.Web3j;
import org.web3j.protocol.http.HttpService;
public class SolanaConnector {
private Web3j web3j;
public SolanaConnector(String endpoint) {
// Initialize web3j with the Solana cluster endpoint
this.web3j = Web3j.build(new HttpService(endpoint));
}
public Web3j getWeb3j() {
return web3j;
}
}
// Usage
SolanaConnector connector = new SolanaConnector("https://api.testnet.solana.com");
In this example, we define a SolanaConnector
class that initializes a connection to a Solana cluster using the HttpService
. This allows you to send requests to the Solana blockchain securely and efficiently.
Forming Custom Instructions
Let's delve into how to create custom instructions using the solana-web3j
library. Consider a scenario where you want to transfer some tokens from one address to another:
import org.web3j.protocol.core.methods.response.TransactionResponse;
import org.web3j.protocol.core.Request;
import org.web3j.protocol.core.methods.response.TransactionReceipt;
public class TokenTransfer {
private SolanaConnector connector;
public TokenTransfer(SolanaConnector connector) {
this.connector = connector;
}
public TransactionResponse transferTokens(String sender, String recipient, long amount) throws Exception {
// Define the custom instruction for token transfer
String instructionData = createTransferInstruction(sender, recipient, amount);
// Execute the transaction
TransactionResponse response = sendTransaction(instructionData);
return response;
}
private String createTransferInstruction(String sender, String recipient, long amount) {
// Create and return the instruction data as a string
return "Transfer Instruction: " + sender + " -> " + recipient + ": " + amount;
}
private TransactionResponse sendTransaction(String instructionData) throws Exception {
// Send the transaction and return the response
Request<?, TransactionReceipt> request = connector.getWeb3j().ethSendTransaction(null);
return request.send();
}
}
Explanation of Code Snippet
In the above example, we define a TokenTransfer
class that includes methods to create a transfer instruction and send it to the Solana network.
-
createTransferInstruction: This method constructs the instruction needed to transfer tokens. For simplification, this example returns a formatted string. In practice, you would serialize this instruction into a format understood by the Solana network.
-
sendTransaction: This method will send the transaction by invoking the
ethSendTransaction
method from thesolana-web3j
library. Ensure to handle exceptions where necessary to catch any potential errors the Solana network might throw.
Signing Transactions
Before transactions can be processed, they need to be signed. Signing asserts the authenticity of the transaction and ensures that it corresponds to the owner's private key.
public String signTransaction(String instructionData, String privateKey) {
// Add logic to sign the transaction
// ...
return signedTransaction;
}
Error Handling
It’s essential to incorporate robust error handling to gracefully manage any issues during interaction with the Solana network. Consider wrapping your blockchain calls in try-catch blocks and logging meaningful error messages. An example snippet would be:
try {
TransactionResponse response = transferTokens(senderAddress, recipientAddress, amount);
} catch (Exception e) {
System.err.println("Error during token transfer: " + e.getMessage());
}
Best Practices
While coding, adhere to these best practices for a more efficient and maintainable codebase:
- Modular Code: Break your components into smaller classes that perform distinct tasks.
- Logging: Utilize a logging framework to log important application events, aiding in debugging and monitoring.
- Security: Keep your private keys secure, and consider environment variables or secure vaults for sensitive configurations.
The Last Word
As blockchain technology continues to gain prominence, the need for streamlined interactions with networks like Solana is ever-increasing. By following the outlined steps and principles, Java developers can create efficient, reliable applications that leverage Solana's capabilities effectively. If you seek a deeper understanding of Solana instruction data, don't hesitate to check out the article "Sending Custom Instructions Using Solana Instruction Data" at infinitejs.com.
By embracing these practices, developers can elevate their Solana interactions, streamline their transactions, and ensure that their applications work seamlessly. Happy coding!
Checkout our other articles