Streamline Your Java Development: Docker for Local Environments
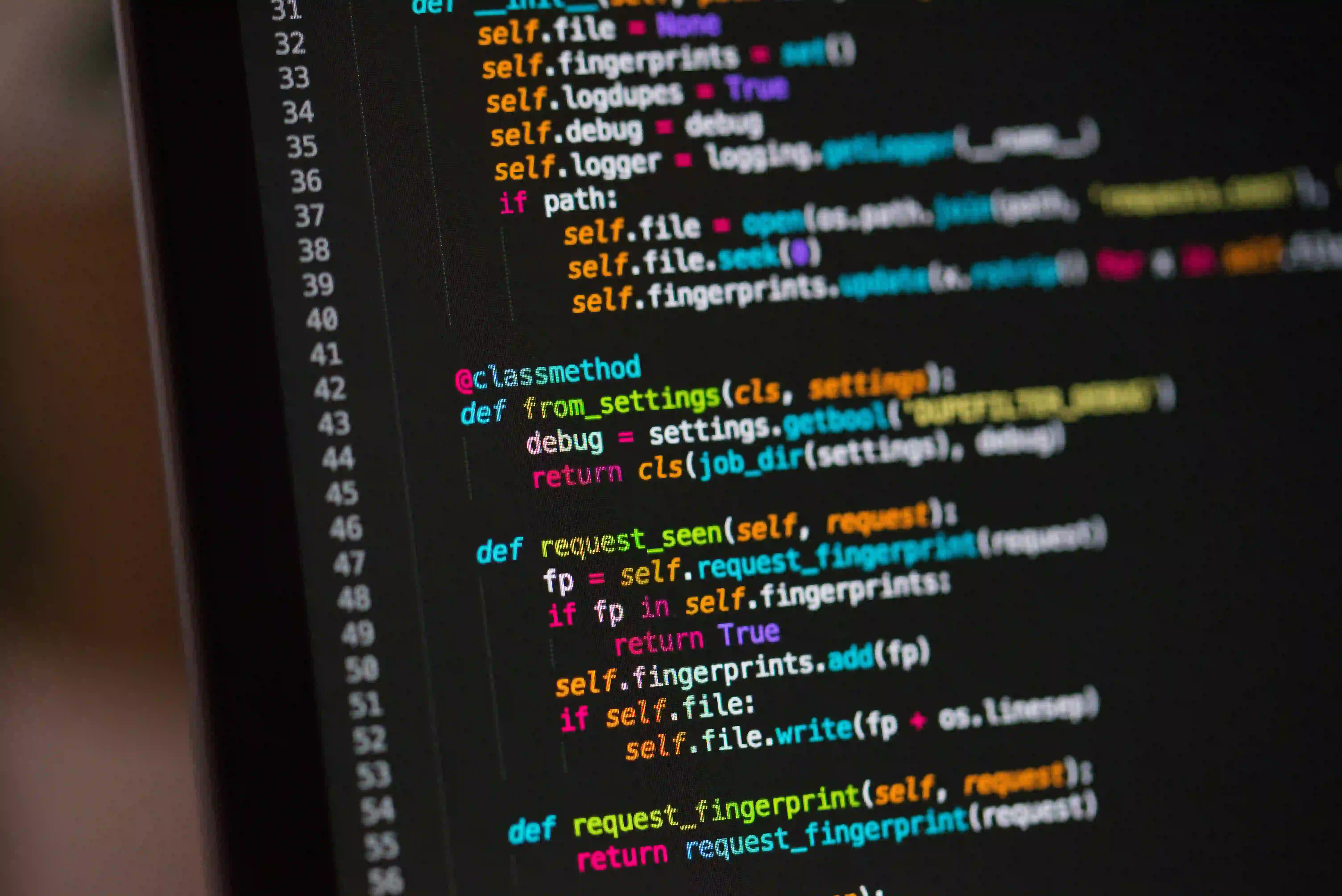
Streamline Your Java Development: Docker for Local Environments
In recent years, containerization has transformed the way developers approach application deployment and local development setups. Docker, in particular, has emerged as a pivotal tool enabling developers to run applications in isolated environments. For Java developers, Docker offers a variety of benefits, including environment consistency, simplified setup, and easier deployment. In this blog post, we will explore how you can streamline your Java development using Docker for local environments.
Why Use Docker in Java Development?
Before diving into practical examples, let’s highlight a few key reasons why Docker is a game-changer for Java developers:
-
Environment Consistency: Docker ensures that your application runs in the same environment in which it was developed. It mitigates the "it works on my machine" syndrome.
-
Isolation: Each Docker container is isolated from others, allowing you to avoid conflicts between different applications and their dependencies.
-
Deployment: Docker makes it easier to move applications from development to production. You can replicate your local setup in production effortlessly.
-
Simplified Configuration: Docker reduces the need for complex setup procedures. You can define your environment using simple configuration files.
Getting Started with Docker
Prerequisites
Before you proceed, ensure you have Docker installed on your machine. You can download it from the official Docker website.
Setting Up Your Java Project
Let’s start with a simple Java application. If you don’t already have a project, create one using Maven, a popular build automation tool for Java.
mkdir hello-docker
cd hello-docker
mvn archetype:generate -DgroupId=com.example -DartifactId=hello-docker -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
cd hello-docker
This command will create a basic Java application structure under the hello-docker
folder.
Sample Java Code
In your project, navigate to src/main/java/com/example/App.java
and replace its content with the following:
package com.example;
public class App {
public static void main(String[] args) {
System.out.println("Hello, Docker!");
}
}
Building Your Docker Image
Now that you have a simple Java application, it’s time to create a Docker image. For that, you'll need to create a Dockerfile
in the project's root directory:
# Use the official Maven image to build the application
FROM maven:3.8.4-openjdk-11 AS build
# Set the working directory inside the container
WORKDIR /app
# Copy the pom.xml and install dependencies
COPY pom.xml ./
RUN mvn dependency:go-offline
# Copy the source code and build the application
COPY src ./src
RUN mvn package
# Use OpenJDK for the final image
FROM openjdk:11-jre-slim
# Copy the built jar file from the build stage
COPY --from=build /app/target/hello-docker-1.0-SNAPSHOT.jar app.jar
# Set the command to run the jar file
ENTRYPOINT ["java", "-jar", "app.jar"]
Explanation of the Dockerfile
-
FROM maven:3.8.4-openjdk-11 AS build: This line sets the base image as Maven with OpenJDK. It uses a multi-stage build approach, allowing you to create an intermediate image for building your application.
-
WORKDIR /app: Sets the working directory inside the container.
-
COPY pom.xml ./: Copies the Maven configuration file into the container.
-
RUN mvn dependency:go-offline: Downloads all dependencies without needing network access later.
-
COPY src ./src: Copies the entire source code directory.
-
RUN mvn package: Packages the application into a JAR file.
-
FROM openjdk:11-jre-slim: Specifies a new base image for the runtime to minimize the image size.
-
COPY --from=build: Copies the built JAR file from the build stage to the new image.
-
ENTRYPOINT ["java", "-jar", "app.jar"]: Specifies how the application will run inside the container.
Building the Docker Image
Once you have your Dockerfile
, you can build your Docker image by running:
docker build -t hello-docker .
Running Your Docker Container
To run your Java application in Docker, use the following command:
docker run --rm hello-docker
You should see your output:
Hello, Docker!
Volume Mounting for Development
During development, you may want to avoid rebuilding your image after every code change. Docker allows you to mount your source code as a volume:
docker run --rm -v "$PWD/src:/app/src" hello-docker
Now, changes in your src
directory will be reflected in the running container without needing to rebuild the image.
Managing Dependencies with Docker Compose
As your Java project grows, managing dependencies can become cumbersome. This is where Docker Compose comes into play. Create a docker-compose.yml
file in your project root and add the following configuration:
version: '3'
services:
app:
build:
context: .
dockerfile: Dockerfile
volumes:
- ./src:/app/src
ports:
- "8080:8080"
With this setup, running docker-compose up
will automatically build the image and start the container, and it will monitor for changes in your src
directory.
The Last Word
Using Docker in your Java development workflow provides significant advantages. From ensuring environment consistency to simplifying deployment and management of your application, Docker has something valuable for every developer.
If you want to delve deeper into how containerization can enhance your local development experience, you might find it informative to read "Ease Your Local Dev: Run Odoo in Docker Effortlessly" on infinitejs.com.
Incorporating Docker into your workflow will not only streamline your development process but also enhance your team’s efficiency by providing a reproducible environment.
Further Reading
Embrace the power of Docker in your Java development journey and watch your productivity soar!