Simplify Java Development: Dockerize Your Spring Boot Apps
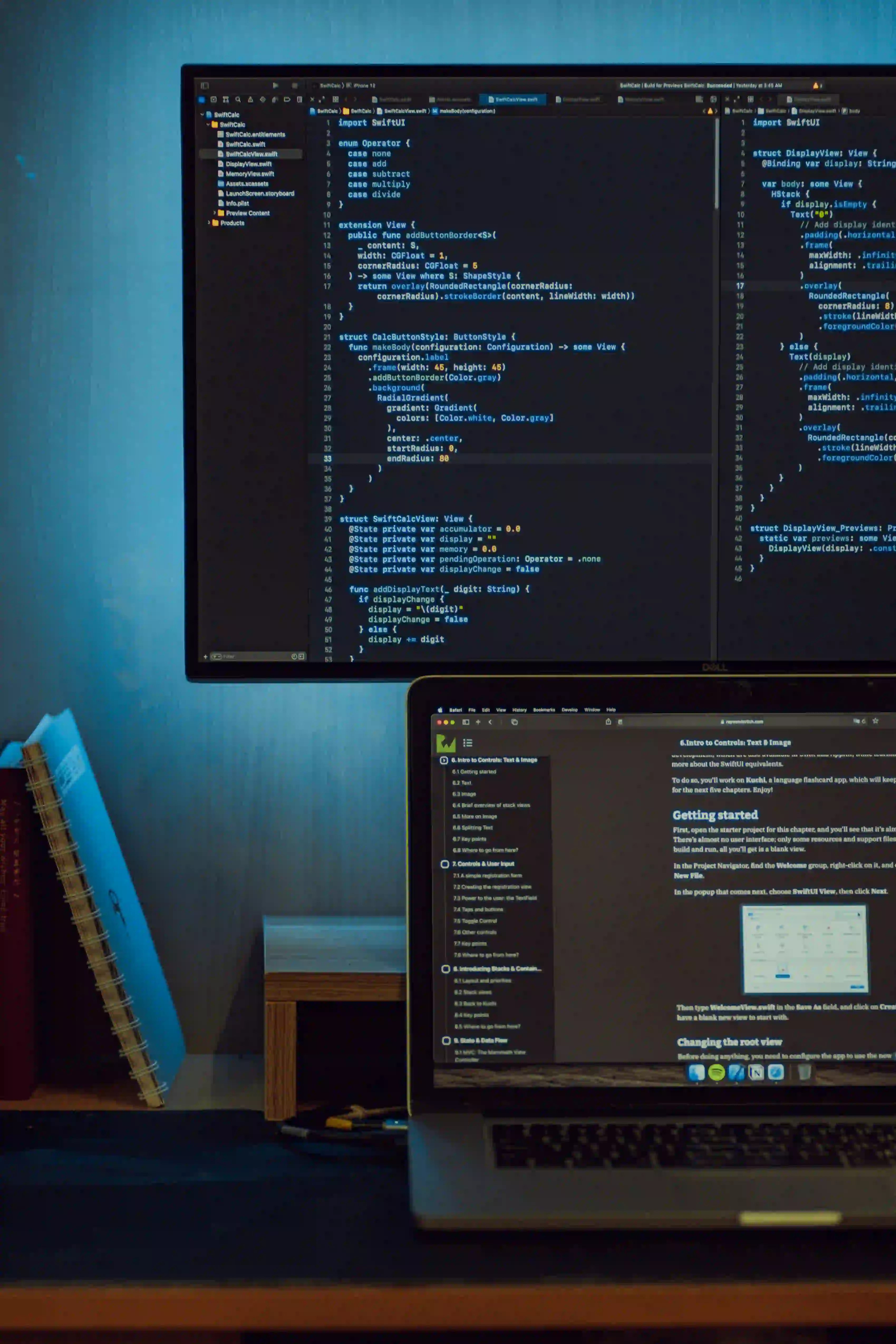
Simplify Java Development: Dockerize Your Spring Boot Apps
Java developers often encounter challenges when packaging and deploying applications. With the introduction of Docker, this has become an excellent solution for streamlining development. In this blog post, we will explore how to Dockerize your Spring Boot applications effectively, simplifying the entire development process.
What Is Docker?
Docker is a platform that allows developers to automate the deployment of applications inside lightweight, portable containers. These containers encapsulate everything your application needs to run, including code, runtime, libraries, and system tools. This ensures your applications run consistently across various environments.
Why Use Docker with Spring Boot?
- Consistency: Docker ensures your application behaves the same way in production as it does in development.
- Isolation: Each container runs independently, avoiding conflicts with other applications on your machine.
- Scalability: Docker makes it easy to scale applications horizontally by replicating containers.
- Simplified CI/CD: Continuous integration and deployment become more straightforward with Docker, as you can build, test, and deploy images seamlessly.
Setting Up Your Spring Boot Application
Before we dive into Dockerizing your Spring Boot application, let’s set up a sample Spring Boot project. Follow these simple steps to create a basic Spring Boot REST application.
1. Create a New Spring Boot Project
You can create a Spring Boot application using Spring Initializr. Select the following options:
- Project: Maven Project
- Language: Java
- Spring Boot Version: 2.5.4 (or the latest version)
- Add Dependencies: Spring Web
Once you generate and download the project, unzip it and navigate to the project directory.
2. Develop Your Application
Now, create a simple REST controller in your project. Here’s an example:
package com.example.demo.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Docker!";
}
}
Why this code? This code snippet defines a REST controller with a single endpoint /hello
that returns a greeting message. It serves as a starting point for our application.
3. Build Your Application
To build the application, navigate to the project directory in your terminal and run the following command:
./mvnw clean package
This command compiles the application and packages it into a JAR file.
Dockerizing Your Spring Boot Application
Now, let’s create a Docker container for our Spring Boot application.
1. Create a Dockerfile
In the root directory of your project, create a file named Dockerfile
. The Dockerfile is a set of instructions Docker uses to assemble the image.
Here's a sample Dockerfile:
# Use a base image with Java
FROM openjdk:11-jre-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the packaged JAR file into the container
COPY target/demo-0.0.1-SNAPSHOT.jar app.jar
# Specify the command to run the JAR file
ENTRYPOINT ["java", "-jar", "app.jar"]
Why this Dockerfile?
- FROM: This line specifies the base image. We're using a slim version of OpenJDK to keep the image lightweight.
- WORKDIR: This sets
/app
as the working directory inside the container. - COPY: We copy the JAR file from our target directory into the container.
- ENTRYPOINT: This command runs when the container starts, executing our JAR file.
2. Build the Docker Image
Once you have created the Dockerfile, you can build the Docker image using the following command:
docker build -t demo-app .
This command tells Docker to build an image with the tag demo-app
using the Dockerfile in the current directory.
3. Run the Docker Container
After building the image, you can run it with the following command:
docker run -d -p 8080:8080 demo-app
Explanation:
-d
: Runs the container in detached mode (in the background).-p 8080:8080
: Maps port 8080 of the host to port 8080 on the container.
You can now access your application by navigating to http://localhost:8080/hello
in your web browser. You should see "Hello, Docker!" displayed.
Benefits of Dockerizing Your Spring Boot Application
1. Easy Environment Management: Docker allows you to specify dependencies and configurations, eliminating the "works on my machine" problem.
2. Quick Deployments: Docker images can be deployed quickly, making it easier to roll out updates or scale applications.
3. Version Control: You can version your Docker images, allowing you to roll back to a previous version if needed.
Further Resources
For further insights into using Docker for development, you can refer to the article titled Ease Your Local Dev: Run Odoo in Docker Effortlessly. It provides an in-depth exploration of leveraging Docker for local development workflows, enhancing your overall productivity.
Closing Remarks
Dockerizing your Spring Boot applications not only simplifies the deployment process but also promotes best practices in software development. By encapsulating all dependencies within a Docker container, you streamline both development and operational workflows.
As you embark on this journey, remember that Docker offers extensive documentation and a vibrant community. Don't hesitate to explore and experiment with different configurations and scenarios to enhance your project further.
Happy coding!