Debugging Low Light Issues in Java-based Vision Systems
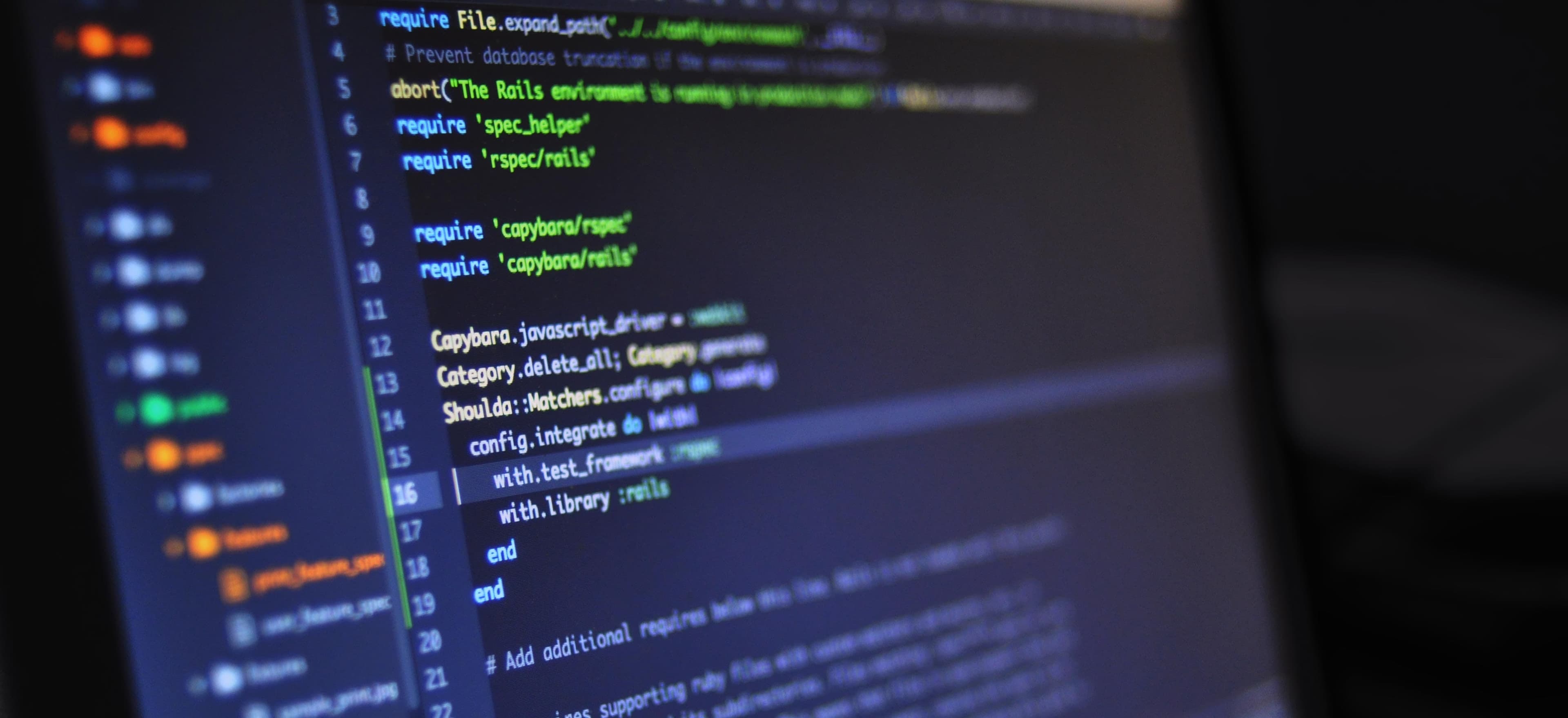
- Published on
Debugging Low Light Issues in Java-based Vision Systems
In the world of computer vision applications, dealing with low-light scenarios can be exceptionally challenging. Java developers often find themselves grappling with issues reminiscent of foggy lenses in night vision binoculars. Just as outlined in the insightful article, "Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars", understanding the components that lead to poor visibility is essential for crafting effective solutions. In this post, we’ll delve into techniques and best practices for debugging low-light issues in Java-based vision systems.
Understanding the Problem
When operating in low-light conditions, camera sensors struggle to capture sufficient light, leading to grainy images, reduced contrast, and lower overall quality. Such challenges may arise in various applications, including security systems, autonomous vehicles, and smartphone cameras.
At the core of these problems lie several issues:
- Noise: Low light conditions amplify sensor noise, resulting in pixel inconsistencies.
- Motion Blur: Longer exposure times can cause blurring when there is movement.
- Dynamic Range Limitations: The sensor may struggle with highlight and shadow detail, leading to a loss of information.
To address these challenges, understanding and implementing the right algorithms and tools is crucial.
Key Techniques for Improving Low-Light Vision in Java
1. Noise Reduction Algorithms
Noise reduction is one of the primary steps in enhancing low-light image quality. Java provides various libraries, such as OpenCV and Apache Commons Imaging, which can help in reducing noise effectively. Below is a simple example of how to apply Gaussian Blur to reduce noise:
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class NoiseReduction {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// Read the input image
Mat image = Imgcodecs.imread("input.jpg");
// Create an output image
Mat output = new Mat();
// Apply Gaussian blur
Imgproc.GaussianBlur(image, output, new Size(5, 5), 0);
// Save the output image
Imgcodecs.imwrite("output.jpg", output);
}
}
Why Use Gaussian Blur?
Gaussian Blur helps in diminishing high-frequency noise components while retaining edges better than median filters in many cases. This allows for a cleaner image, which is especially crucial when the lighting is dim.
2. Histogram Equalization
Histogram equalization enhances the contrast of images. It redistributes the intensity values, leading to a more balanced image overall. Below is a sample code snippet demonstrating how to perform histogram equalization:
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.CvType;
import org.opencv.core.MatOfInt;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class HistogramEqualization {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// Read the input image
Mat image = Imgcodecs.imread("input.jpg", Imgcodecs.IMREAD_GRAYSCALE);
// Create an output image
Mat output = new Mat();
// Apply histogram equalization
Imgproc.equalizeHist(image, output);
// Save the output image
Imgcodecs.imwrite("output.jpg", output);
}
}
Why Equalize the Histogram?
Histogram equalization increases the dynamic range of the image, making details more visible. In low-light images, it aids in retrieving hidden data typically obscured by darkness.
3. Image Enhancement Techniques
Contrast-limited adaptive histogram equalization (CLAHE) is an advanced method for local contrast enhancement. Unlike standard histogram equalization, CLAHE operates on small tiles, making it more effective for revealing features in shadows.
Here’s how to implement CLAHE in Java:
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.imgproc.Imgproc.CLAHE;
public class CLAHEExample {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// Read the input image
Mat image = Imgcodecs.imread("input.jpg", Imgcodecs.IMREAD_GRAYSCALE);
// Initialize CLAHE
CLAHE clahe = Imgproc.createCLAHE();
clahe.setClipLimit(2.0); // Set clip limit to control contrast limit
Mat output = new Mat();
// Apply CLAHE
clahe.apply(image, output);
// Save the output image
Imgcodecs.imwrite("output.jpg", output);
}
}
Why Use CLAHE?
CLAHE is particularly necessary in images where the foreground may be significantly brighter than the background. By enhancing local contrast, you can pull out critical details lost in predominantly dark images.
4. Integrate Machine Learning for Better Analysis
Deep learning has revolutionized image processing. Libraries such as TensorFlow and DL4J allow you to build predictive models capable of distinguishing between salient and non-salient features within images.
By training a model on your specific dataset, you can create a tailored solution to recognize objects even in low lighting.
// pseudo-code for using a trained deep learning model
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
public class ObjectDetection {
public static void main(String[] args) {
// Load trained model
MultiLayerNetwork model = MultiLayerNetwork.load("model.zip");
// Assume inputMat is your processed image Mat
// Preprocess input image for your model
INDArray input = preprocess(inputMat);
// Make prediction
INDArray output = model.output(input);
// Post-process and visualize results
visualizeResults(output);
}
}
Why Use Machine Learning?
Machine learning provides a robust solution for recognizing patterns in images. It improves with data, meaning the more images you feed, the better your system becomes in low-light scenarios.
5. Camera Settings Optimization
Optimizing camera settings, such as ISO level and exposure time, can significantly enhance image quality in low light. Though not directly related to Java coding, ensure that your hardware settings are configured correctly, as post-processing can’t substitute for poor initial inputs.
My Closing Thoughts on the Matter
Navigating low-light conditions in Java-based vision systems demands a multifaceted approach. From noise reduction and histogram equalization to advanced methods like CLAHE and machine learning models, there are numerous paths to improving image quality.
As you implement these techniques, remember the parallels drawn to the insights from "Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars"; clarity and visibility depend on understanding and tackling the underlying challenges.
By employing the strategies discussed, you can transform your Java vision systems into capable performers, even when the lights go down. Happy coding!
Checkout our other articles