Java Solutions for Enhancing Image Clarity in Night Vision
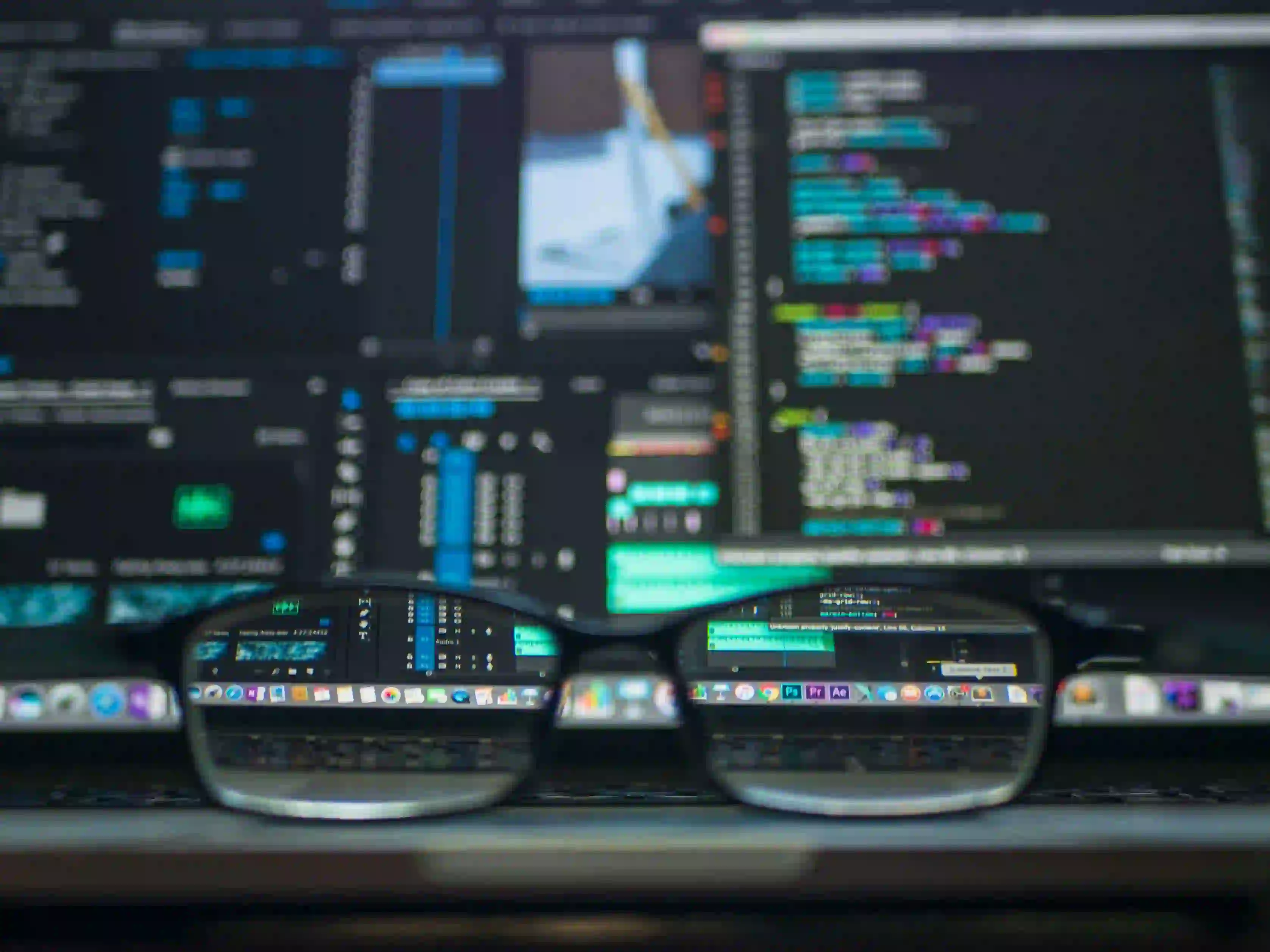
Java Solutions for Enhancing Image Clarity in Night Vision
Night vision technology is not merely a niche venture; it has broad applications ranging from wildlife observation to military use. However, one common issue users face is image clarity, especially when dealing with foggy lenses. A recent article, "Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars," discusses remedies to mitigate this issue (youvswild.com/blog/solving-foggy-lens-night-vision-binoculars). In this blog post, we explore how Java can be used to enhance image clarity in night vision applications, leveraging simple computational techniques.
Understanding Image Processing
Before diving into Java solutions, it's important to understand image processing fundamentals. Image processing involves manipulating pixel data to enhance or extract information. Night vision images often suffer from noise, blurriness, or reduced contrast. Our goal in this post is to process images to enhance their visual quality.
Key Concepts in Image Processing:
- Contrast Enhancement: Improving the differences in color and brightness in an image.
- Noise Reduction: Removing unwanted variations in pixel values.
- Histogram Equalization: A method that adjusts the contrast of the image.
These techniques can be implemented using Java's powerful image processing libraries.
Setting Up Your Java Environment
Ensure you have a suitable Java development environment. You can use any IDE, but IntelliJ IDEA and Eclipse are widely used. Make sure you add the following libraries to your project:
<dependency>
<groupId>javax.media</groupId>
<artifactId>jai-core</artifactId>
<version>1.1.3</version>
</dependency>
Handling Image Input/Output
You will need a way to load and display images. Java's built-in libraries provide methods to handle images easily.
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class ImageLoader {
public static BufferedImage loadImage(String path) throws IOException {
return ImageIO.read(new File(path));
}
}
In the code above, we define a method loadImage
that reads an image file and returns a BufferedImage object. This object is crucial for all subsequent image manipulations.
Contrast Enhancement
Enhancing the contrast can significantly improve the clarity of an image. The simplest and most effective method is linear contrast stretching.
Implementing Contrast Stretching
public class ContrastEnhancer {
public static BufferedImage enhanceContrast(BufferedImage img) {
int width = img.getWidth();
int height = img.getHeight();
BufferedImage enhancedImg = new BufferedImage(width, height, img.getType());
// Find min and max pixel values
int minVal = 255, maxVal = 0;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int pixel = img.getRGB(x, y) & 0xff;
minVal = Math.min(minVal, pixel);
maxVal = Math.max(maxVal, pixel);
}
}
// Stretch contrast
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int pixel = img.getRGB(x, y) & 0xff;
int newPixel = (int) (((pixel - minVal) / (maxVal - minVal)) * 255);
enhancedImg.setRGB(x, y, (newPixel << 16) | (newPixel << 8) | newPixel);
}
}
return enhancedImg;
}
}
Explanation
- We first loop through the image to find the minimum and maximum pixel values.
- We then create a new image where the pixel values are stretched to span the full range of 0-255.
- This method is efficient and can dramatically improve contrast, especially in dimly lit images.
Noise Reduction
Reducing noise is vital, mainly because night vision footage can contain significant grain or artifacts. One common approach is to apply a Gaussian blur filter.
Implementing Gaussian Blur
import java.awt.image.Kernel;
import java.awt.image.ConvolveOp;
public class NoiseReducer {
public static BufferedImage reduceNoise(BufferedImage img) {
float[] matrix = {
1/16f, 2/16f, 1/16f,
2/16f, 4/16f, 2/16f,
1/16f, 2/16f, 1/16f
};
Kernel kernel = new Kernel(3, 3, matrix);
ConvolveOp convolve = new ConvolveOp(kernel);
return convolve.filter(img, null);
}
}
Explanation
- We define a Gaussian kernel and apply it using a convolution operation.
- This effectively smooths out the pixels in the image, helping reduce noise without overly blurring the details.
Histogram Equalization
Histogram Equalization is another powerful method to improve the visibility of features in an image.
Implementing Histogram Equalization
public class HistogramEqualizer {
public static BufferedImage equalize(BufferedImage img) {
// Convert to grayscale
BufferedImage grayscaleImg = new BufferedImage(img.getWidth(), img.getHeight(), BufferedImage.TYPE_BYTE_GRAY);
for (int y = 0; y < img.getHeight(); y++) {
for (int x = 0; x < img.getWidth(); x++) {
int rgb = img.getRGB(x, y);
int gray = (int) ((0.299 * ((rgb >> 16) & 0xff)) + (0.587 * ((rgb >> 8) & 0xff)) + (0.114 * (rgb & 0xff)));
grayscaleImg.setRGB(x, y, gray << 16 | gray << 8 | gray);
}
}
// Calculate histogram
int[] histogram = new int[256];
for (int y = 0; y < grayscaleImg.getHeight(); y++) {
for (int x = 0; x < grayscaleImg.getWidth(); x++) {
int pixel = grayscaleImg.getRGB(x, y) & 0xff;
histogram[pixel]++;
}
}
// Normalize histogram
float[] cdf = new float[256];
cdf[0] = histogram[0];
for (int i = 1; i < histogram.length; i++) {
cdf[i] = cdf[i - 1] + histogram[i];
}
for (int i = 0; i < cdf.length; i++) {
cdf[i] = (cdf[i] - cdf[0]) * 255 / (grayscaleImg.getWidth() * grayscaleImg.getHeight() - cdf[0]);
}
// Apply equalization
BufferedImage equalizedImg = new BufferedImage(img.getWidth(), img.getHeight(), img.getType());
for (int y = 0; y < grayscaleImg.getHeight(); y++) {
for (int x = 0; x < grayscaleImg.getWidth(); x++) {
int pixel = grayscaleImg.getRGB(x, y) & 0xff;
int newPixel = (int) cdf[pixel];
equalizedImg.setRGB(x, y, (newPixel << 16) | (newPixel << 8) | newPixel);
}
}
return equalizedImg;
}
}
Explanation
- We first convert the image to grayscale to simplify processing.
- A histogram is built to track pixel intensity distribution, followed by normalizing and applying the transformation to enhance the histogram.
- The final image displays improved details and contrast, facilitating better visibility in lower-light conditions.
Closing Remarks
Java offers powerful tools to enhance clarity in night vision images through contrast enhancement, noise reduction, and histogram equalization. By creating and applying these algorithms, we empower users with clearer and more usable imagery.
As the technology evolves, considerations like real-time processing and hardware acceleration will also need to be addressed. However, these foundational techniques serve as an excellent starting point for those interested in image processing using Java.
For a broader discussion on issues related to night vision, including solutions for foggy lenses, check out the article on youvswild.com: Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars.
Happy coding!