Java Developers: Fuel Your Code with Positive Inspiration
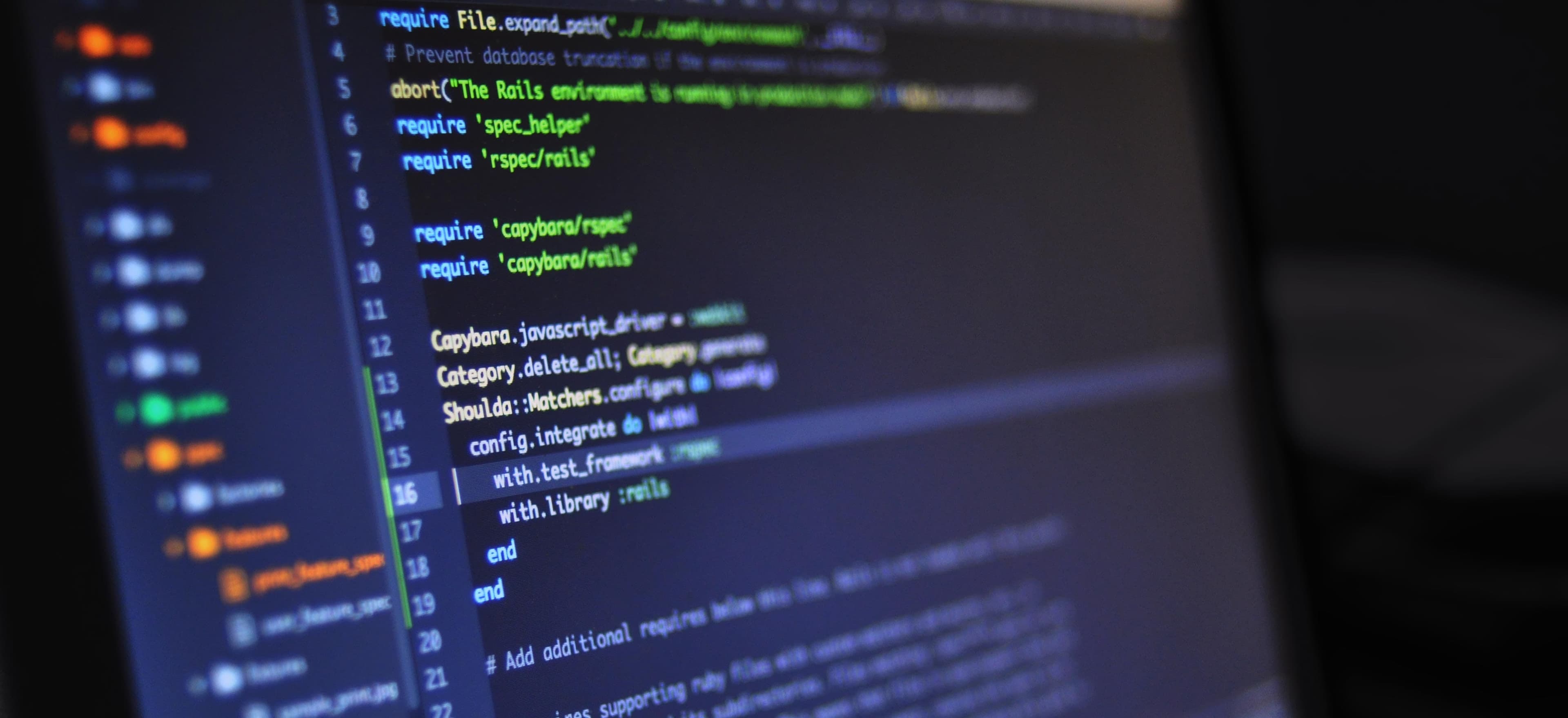
- Published on
Java Developers: Fuel Your Code with Positive Inspiration
As a Java developer, you know that programming requires not just technical skills but also a positive mindset. It’s easy to feel lost when faced with a complex problem or an overwhelming project. Yet, like any profession, a sprinkle of motivation can do wonders for your productivity and creativity. In this blog post, we will explore ways to inspire yourself while working with Java, draw connections to personal development, and even see how powerful quotes can motivate coding excellence.
The Role of Inspiration in Java Development
Inspiration fuels innovation. Likewise, finding ways to stay motivated can impact your coding experience. When distractions loom large or failures arise, a positive mindset can help you re-center and refocus on the task ahead. This is essential in a language like Java, which is frequently used for enterprise-level applications due to its stability and robustness.
The Power of Quotes
Sometimes, all it takes is a few well-chosen words to shift your perspective. The article "Overcoming Cynicism: Motivating Quotes for Modern Life" offers a plethora of inspiring quotes that can lift anyone’s spirits, including developers. Here, we’ll extract motivation from a couple of these quotes and translate that positivity into our coding practices.
Coding with Clarity: Keep It Simple
One of the fundamental principles of Java development is simplicity. The Java mantra "Keep It Simple, Stupid" (KISS) reminds developers to write code that is easy to read and maintain.
Example: Simple Code Structure
public class Greeting {
public String createGreeting(String name) {
return "Hello, " + name + "!";
}
public static void main(String[] args) {
Greeting greet = new Greeting();
System.out.println(greet.createGreeting("Java Developer"));
}
}
Why this works: This code snippet illustrates a basic class with a single method for greeting. Instead of complicating things, we maintained clarity with method functionality, which is easier to enhance later. Simplicity is not a sign of weakness; it is a strength. By keeping your code simple, you allow yourself to step back and see the larger picture rather than getting bogged down by intricate details.
Embrace Challenges
Another motivating quote that aligns well with coding is, "Fall seven times, stand up eight." Encountering bugs and issues is inevitable when developing software. Instead of seeing these as failures, view them as opportunities to learn and improve.
Example: Debugging Practice
public class Division {
public static void divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero.");
}
System.out.println("Result: " + (numerator / denominator));
}
public static void main(String[] args) {
try {
divide(10, 0); // This will throw an exception
} catch (IllegalArgumentException e) {
System.err.println(e.getMessage());
}
}
}
Why this works: Handling exceptions properly not only enhances the robustness of your application but also teaches you resilience. Rather than crumbling under the pressure of a runtime error, addressing it methodically can bolster your skills. When you face challenges, you'll find that each bug fixed leads to a more refined product.
Cultivate a Growth Mindset
Adopting a growth mindset is crucial. This means you believe your skills and intelligence can be developed over time. As a Java developer, you're constantly learning, be it new libraries, frameworks, or best practices.
Example: Leveraging Java Libraries
import java.util.Arrays;
import java.util.List;
public class CollectionExample {
public static void main(String[] args) {
List<String> items = Arrays.asList("Java", "Python", "JavaScript");
items.forEach(item -> System.out.println(item.toUpperCase()));
}
}
Why this works: This snippet showcases the use of the Java Collections Framework and lambda expressions, which simplify code and make it more readable. By actively seeking to use modern practices, you are nurturing your growth mindset. An encouraging approach would be to embrace libraries that lift the burden of manual tasks.
Network and Share
Collaboration opens the doors of opportunity. Engage with your peer developers. Share your successes and struggles; you may find inspiration from their journeys.
Example: Code Review
In a collaborative project setting, always encourage code reviews. Example scenarios during these reviews could include:
- Pointing out code optimizations.
- Suggesting alternative libraries.
- Sharing personal lessons learned from a coding challenge.
Why this works: A network of support fosters a culture of learning. It builds confidence knowing you can rely on others when facing difficulties. This can help drive motivation and lead to innovative solutions.
Developing Your Skills
Investing time in continuous education will pay dividends in your development career. Resources like online courses, webinars, and coding challenges can keep you sharp.
Example: Online Coding Challenge
Consider participating in platforms like HackerRank for Java challenges. Here's a simple example of how to handle a coding challenge for summing an array of numbers.
public class SumArray {
public static int sum(int[] numbers) {
int total = 0;
for(int number : numbers) {
total += number;
}
return total;
}
public static void main(String[] args) {
int[] values = {1, 2, 3, 4, 5};
System.out.println("Total sum is: " + sum(values));
}
}
Why this works: This straightforward structure not only assists in mastering loops but also reinforces your understanding of arrays. Challenges like this foster mastery while maintaining motivation as you progress.
In Conclusion, Here is What Matters
As a Java developer, you have the tools to ignite your potential. Draw inspiration from quotes, welcome challenges, focus on simplicity, deepen your skills, and engage with a community. Positivity is contagious, and just as you uplift yourself, you can inspire others.
Remember, the journey of programming is as important as the destination. So, next time you feel overwhelmed, revisit the motivating wisdom from sources like the article on overcoming cynicism and lift your spirit - you’re not just writing code, you’re crafting the future. Happy coding!