Clearing the Fog: Java Solutions for Image Processing Challenges
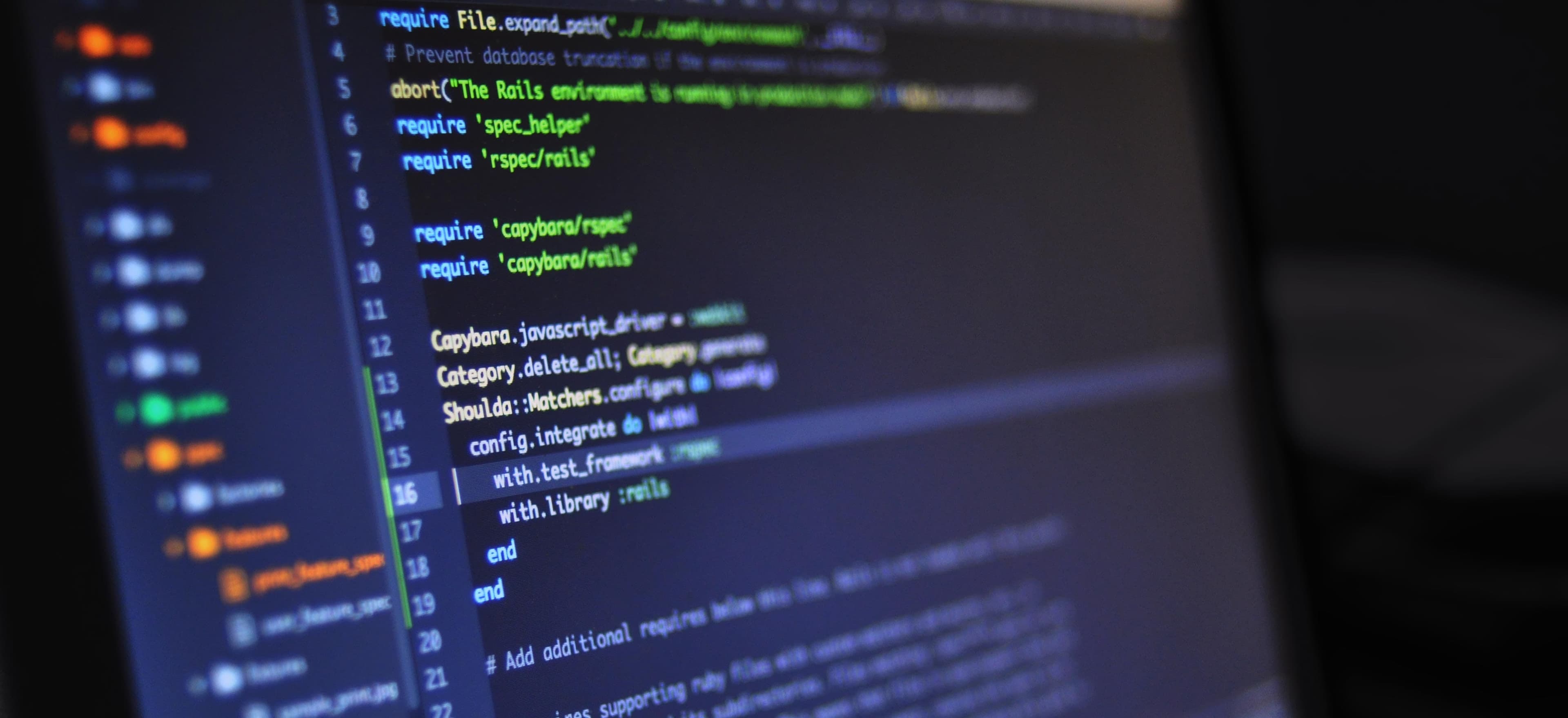
- Published on
Clearing the Fog: Java Solutions for Image Processing Challenges
In the world of software development, image processing can be one of the most fascinating and complex areas. With applications ranging from medical imaging to developing advanced surveillance systems, the need for clear and effective images is paramount. In this blog post, we will explore how Java can help mitigate various image processing challenges, such as the foggy lens issue referenced in the article "Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars" (youvswild.com/blog/solving-foggy-lens-night-vision-binoculars).
Whether you're a seasoned developer or just dipping your toes into the world of image processing, this tutorial will equip you with practical solutions and code snippets to clear the fog in your projects.
Understanding Foggy Images
Fog in images can obscure vital details, making it challenging for users to gain accurate insights. The causes can range from poor lighting conditions to suboptimal camera performance. However, with the right techniques, we can enhance the visibility and contrast in images.
Image Enhancement Techniques
Before we dive into code, let’s discuss some popular image enhancement techniques:
- Histogram Equalization: This technique improves the contrast of an image by stretching out the intensity range.
- Contrast Stretching: By adjusting the contrast levels, we can bring out hidden details in foggy images.
- Dehazing Algorithms: A more sophisticated technique that removes haze or fog from images based on atmospheric scattering models.
Java Libraries for Image Processing
To implement these techniques, you'll often find it beneficial to use libraries that can streamline your workflow. The Java Advanced Imaging (JAI) API and OpenCV are two powerful libraries you can leverage for image processing in Java.
Getting Started with Java Image Processing
Let's walk through how to implement a basic image enhancement technique—Histogram Equalization—using Java. For this, we will use the JAI library.
Setting Up Your Environment
First, ensure that you have the following tools installed:
- Java Development Kit (JDK)
- JAI library (can be downloaded from here)
Sample Code for Histogram Equalization
import javax.media.jai.*;
import java.awt.image.BufferedImage;
public class HistogramEqualization {
public static BufferedImage equalizeHistogram(BufferedImage img) {
// Create a PlanarImage from the BufferedImage
PlanarImage source = PlanarImage.wrapRenderedImage(img);
// Perform histogram equalization
PlanarImage equalizedImage = (PlanarImage) new HistogramEqualizationOpImage(source).create();
return equalizedImage.getAsBufferedImage();
}
public static void main(String[] args) {
// Load your image here
BufferedImage img = ... // Load image logic here
// Equalize histogram
BufferedImage equalizedImg = equalizeHistogram(img);
// Save or display the image
... // Save/display implementation
System.out.println("Histogram equalization completed.");
}
}
Commentary on the Code
- Imports: We import necessary classes from the JAI library.
- Method Declaration: The
equalizeHistogram
method takes aBufferedImage
as input and applies the histogram equalization. - PlanarImage: We wrap the
BufferedImage
to create aPlanarImage
, suitable for processing. - Creation of Equalized Image: We use JAI’s
HistogramEqualizationOpImage
to perform the actual processing. - Main Method: This method ties everything together by loading an image and calling our equalization method.
Why Histogram Equalization?
Histogram equalization is a powerful technique to improve image visibility. It works by redistributing the intensity values to cover the entire range. This is particularly useful for foggy images as it can bring out obscured areas that otherwise would remain hidden.
More Advanced Techniques
While histogram equalization is effective, it may not be enough for heavy fog situations. This is where dehazing algorithms come into play.
Implementing a Simple Dehazing Algorithm
For this section, let’s demonstrate a straightforward approach to remove haze using an atmospheric scattering model.
import java.awt.image.BufferedImage;
public class BasicDehaze {
public static BufferedImage dehaze(BufferedImage img, float A) {
int width = img.getWidth();
int height = img.getHeight();
BufferedImage output = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int rgb = img.getRGB(x, y);
int r = (rgb >> 16) & 0xff;
int g = (rgb >> 8) & 0xff;
int b = rgb & 0xff;
// Applying the dehazing algorithm
r = Math.min(255, (int)(r - (A / (A + 1)) * 255));
g = Math.min(255, (int)(g - (A / (A + 1)) * 255));
b = Math.min(255, (int)(b - (A / (A + 1)) * 255));
output.setRGB(x, y, (r << 16) | (g << 8) | b);
}
}
return output;
}
public static void main(String[] args) {
// Load your image here
BufferedImage img = ... // Load image logic here
float atmosphericLight = 0.1f; // Example atmospheric light
BufferedImage dehazedImg = dehaze(img, atmosphericLight);
// Save or display the image
... // Save/display implementation
System.out.println("Dehazing completed.");
}
}
Commentary on the Code
- Atmospheric Light (A): The
A
value is crucial as it represents the global atmospheric light. Proper tuning can lead to different results. - Pixel Manipulation: For each pixel, we extract RGB values, apply the dehaze formula, and set the new RGB values in the output image.
- Efficiency Considerations: This basic algorithm is not optimized for performance, but it serves well to illustrate the concept.
Why Use Dehazing?
Dehazing can significantly improve image quality in foggy conditions by restoring visibility. It’s particularly useful in applications such as low-light photography and vision systems.
Bringing It All Together
In this blog post, we've explored some effective techniques for clearing fog from images using Java. From simple histogram equalization to more advanced dehazing algorithms, Java’s robust libraries enable you to tackle multiple image processing challenges.
If you're aspiring to enhance your image processing skills, consider experimenting with these techniques. And for those intrigued by challenging situations such as foggy lenses in night vision binoculars, I recommend checking out the article "Seeing in the Dark: Solving Foggy Lens in Night Vision Binoculars" (youvswild.com/blog/solving-foggy-lens-night-vision-binoculars).
Stay curious, keep coding, and happy processing!
Checkout our other articles