Mastering Java for Problem Solving: Factors and Multiples Explained
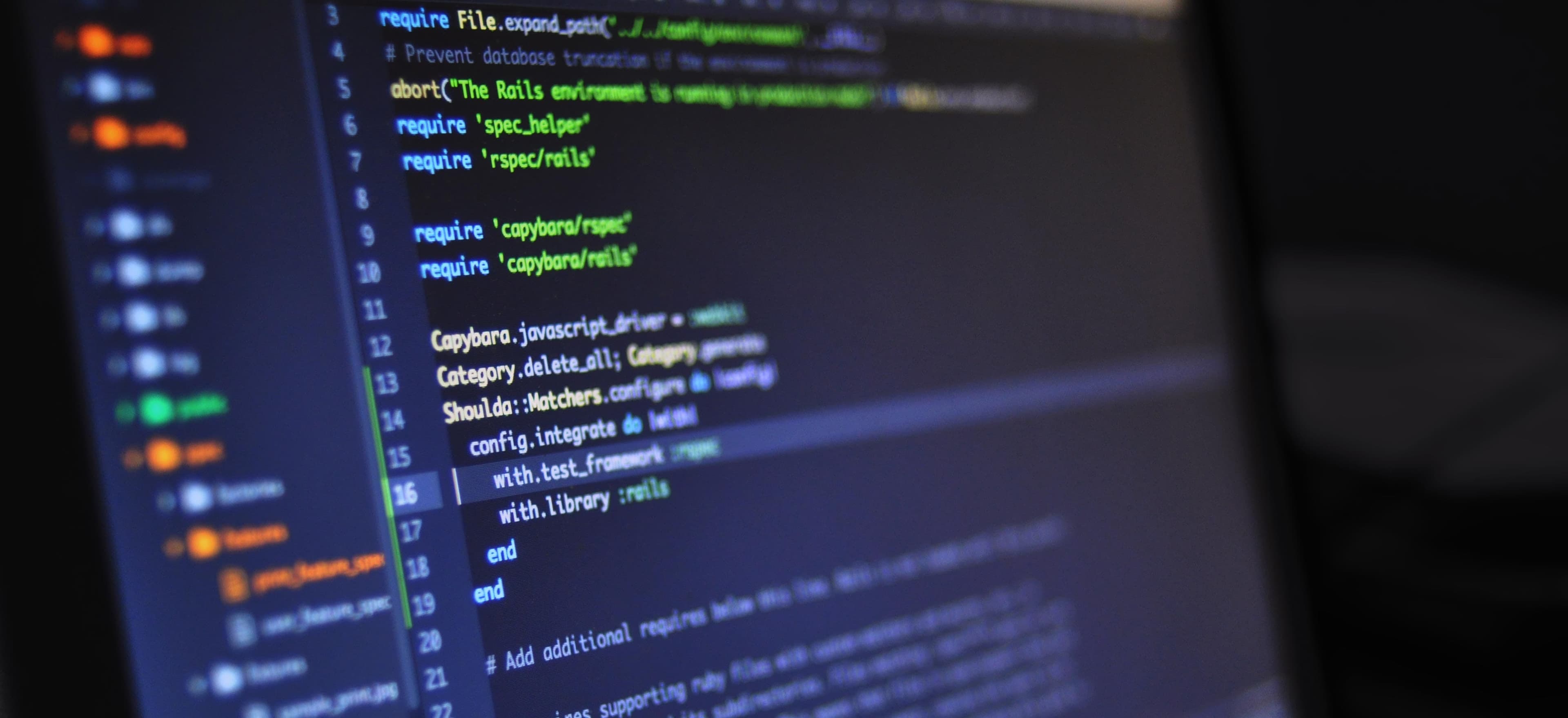
- Published on
Mastering Java for Problem Solving: Factors and Multiples Explained
Java is a powerful programming language known for its versatility and efficiency. It can be used for various applications, from simple scripts to complex enterprise systems. Understanding core concepts in Java, such as factors and multiples, can enhance your problem-solving abilities. This blog post will explore these concepts using Java code examples and help you tackle problems effectively, referencing existing approaches outlined in the article "Verständnisprobleme bei Vielfachen und Teilern leicht gelöst" (lernflux.com/post/verstaendnisprobleme-bei-vielfachen-und-teilern-geloest).
What Are Factors and Multiples?
Before diving into Java coding, it is essential to grasp what factors and multiples are:
- Factors of a number are integers that can be multiplied together to obtain that number. For example, for the number 12, the factors are 1, 2, 3, 4, 6, and 12.
- Multiples of a number arise from multiplying that number by integers. For instance, for the number 3, the first five multiples are 3, 6, 9, 12, and 15.
Having a solid understanding of factors and multiples is crucial in solving numerous mathematical and programming challenges.
Java Implementation: Finding Factors
Finding the factors of a number in Java involves iterating through possible divisors and checking if they evenly divide the number. Let’s look at how to achieve this.
Code Snippet: Finding Factors
import java.util.ArrayList;
import java.util.List;
public class FactorFinder {
public static List<Integer> findFactors(int number) {
List<Integer> factors = new ArrayList<>();
for (int i = 1; i <= number; i++) {
if (number % i == 0) { // Check if i is a factor of number
factors.add(i);
}
}
return factors; // Return the list of factors
}
public static void main(String[] args) {
int number = 12; // Example number
List<Integer> factors = findFactors(number);
System.out.println("Factors of " + number + ": " + factors);
}
}
Explanation
- List Creation: We create a
List<Integer>
to store our factors. - Loop Through Numbers: The loop iterates from 1 to the target number. For every integer
i
, we check ifi
divides the target number without a remainder (number % i == 0
). - Adding Factors: If the condition is satisfied, we add
i
to our list of factors. - Output: Finally, we print the resulting factors.
This code succinctly demonstrates how to break down the problem into manageable parts, leveraging the properties of numbers.
Java Implementation: Finding Multiples
Finding multiples is just as straightforward as finding factors. Instead of checking divisibility, we'll multiply the base number by integers.
Code Snippet: Finding Multiples
import java.util.ArrayList;
import java.util.List;
public class MultipleFinder {
public static List<Integer> findMultiples(int base, int count) {
List<Integer> multiples = new ArrayList<>();
for (int i = 1; i <= count; i++) {
multiples.add(base * i); // Generate and store the multiple
}
return multiples; // Return the list of multiples
}
public static void main(String[] args) {
int base = 3; // Example base number
int count = 5; // Number of multiples to find
List<Integer> multiples = findMultiples(base, count);
System.out.println("First " + count + " multiples of " + base + ": " + multiples);
}
}
Explanation
- List Generation: Similar to the factor finder, we initialize a
List<Integer>
to hold the multiples. - Loop for Multiples: The loop runs from 1 to
count
, multiplying the base number byi
each iteration to generate the multiples. - Output: We then return and print the list of multiples.
This example illustrates another method to break down mathematical operations into a logical Java code format.
Exploring Real-World Applications
Understanding factors and multiples has practical applications in a variety of fields, from computer programming to data analysis. For example, knowing the factors and multiples of a number can aid in optimization algorithms, resource distribution tasks, and even game development.
Common Use Cases
- Optimizations: Some algorithms improve efficiency by utilizing factors.
- Resource Allocation: Multiples are often used to distribute resources evenly.
Related Concepts
In addition to factors and multiples, related concepts such as greatest common divisor (GCD) and least common multiple (LCM) can further enhance your mathematical toolkit. Understanding these concepts allows you to solve more complex problems with ease.
Example: Finding GCD
Here’s a quick illustration of how you might find the GCD of two numbers:
public class GCD {
public static int findGCD(int a, int b) {
if (b == 0) {
return a; // Base case: GCD is a when b is 0
}
return findGCD(b, a % b); // Recursive case
}
public static void main(String[] args) {
int num1 = 48;
int num2 = 18;
System.out.println("GCD of " + num1 + " and " + num2 + " is: " + findGCD(num1, num2));
}
}
Explanation
The above method uses recursion to find the GCD of two numbers. The function continues to call itself until b becomes zero, at which point a is returned as the GCD.
The Closing Argument
Mastering factors and multiples in Java can significantly bolster your problem-solving skills. With the foundational understanding and practical code examples provided in this post, you can confidently tackle various programming challenges. Whether you are developing algorithms, designing applications, or simply brushing up on your Java skills, these principles remain crucial.
For anyone facing difficulties in understanding the intricacies of factors and multiples, consider reading the article "Verständnisprobleme bei Vielfachen und Teilern leicht gelöst" (lernflux.com/post/verstaendnisprobleme-bei-vielfachen-und-teilern-geloest). It offers additional insights that can complement the examples discussed here.
Further Learning
To continue your journey into Java programming, consider exploring:
- Data Structures: Lists, Maps, and Sets for better data management.
- Algorithms: Sorting and Searching algorithms to refine your technical skills.
- Frameworks: Spring and Hibernate for enterprise-level development.
Embrace these concepts and practice regularly to become a proficient Java programmer. Happy coding!
Checkout our other articles