Streamline Java Development: Dockerize Your Java Apps Today!
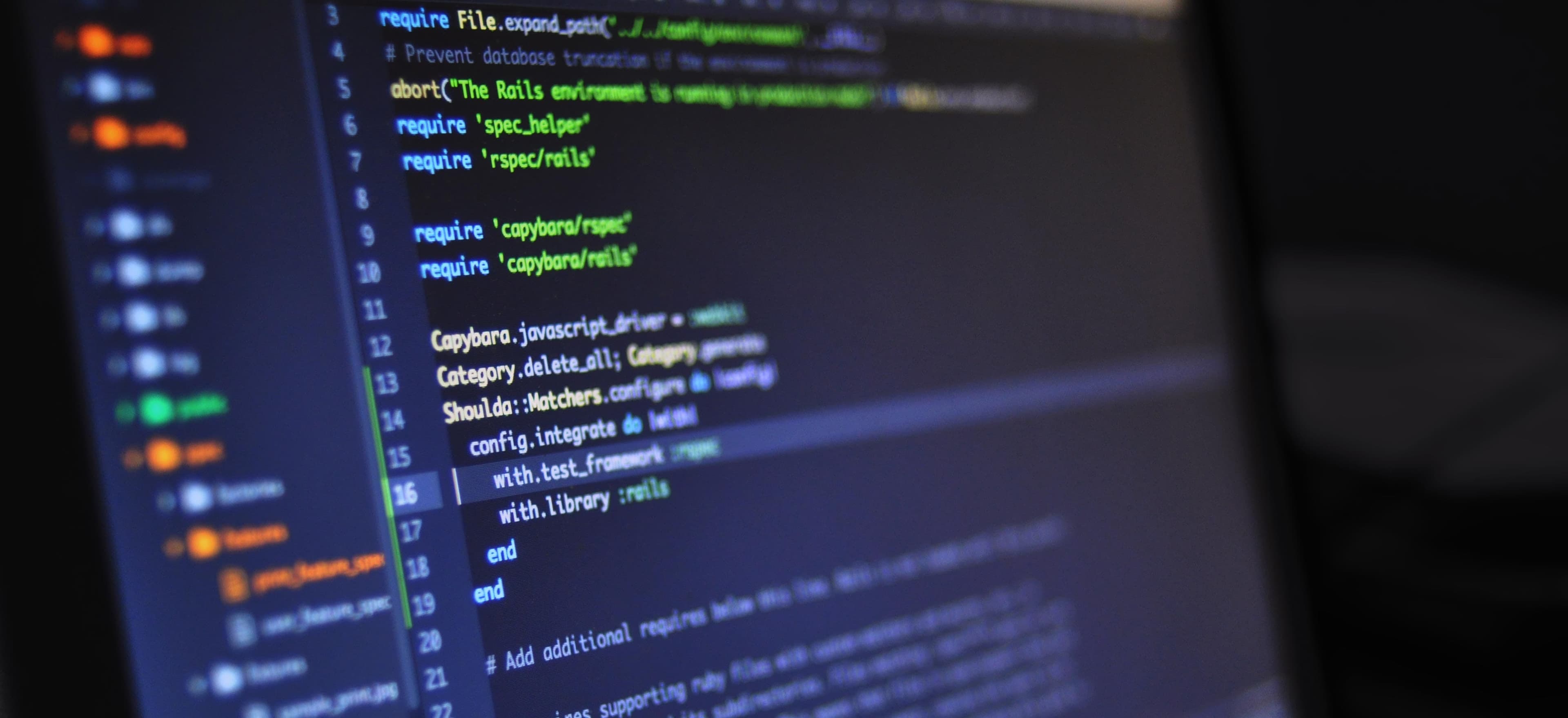
- Published on
Streamline Java Development: Dockerize Your Java Apps Today!
In the world of software development, efficiency is key. For Java developers, Docker can drastically streamline the development process, enabling smooth deployment and management of applications across various platforms. In this blog post, we will explore how to leverage Docker for your Java applications, simplifying both local development and deployment.
Why Use Docker for Java Development?
Docker is an open-source platform that helps automate the deployment of applications in lightweight, portable containers. Here are some key benefits of using Docker for Java applications:
-
Consistency Across Environments: Docker ensures your application runs the same way regardless of where it's deployed. Local development, staging, and production environments can all mirror each other precisely.
-
Isolation: By using containers, you can separate your Java applications and their dependencies. This minimizes potential conflicts caused by different library versions.
-
Easy Scaling & Management: Docker containers can be easily replicated and deployed, allowing for efficient scaling of applications up or down based on needs.
-
Integrated Development: The Docker ecosystem integrates well with Continuous Integration/Continuous Deployment (CI/CD) pipelines, enhancing your development workflow.
Getting Started with Docker for Java
Before diving into the implementation, ensure you have Docker installed on your machine. You can download Docker from the official website.
Step 1: Create Your Java Application
Let’s start with a basic Java application. We will create a simple "Hello World" application. Create a file named HelloWorld.java
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Docker!");
}
}
Save this code in a directory (for example, my-java-app
).
Step 2: Dockerize Your Application
- Create a Dockerfile: A Dockerfile is a text document that contains all the commands to assemble an image. Here's how to create one for our Java application:
# Use an official Java runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . .
# Compile the Java program
RUN javac HelloWorld.java
# Run the Java program
CMD ["java", "HelloWorld"]
Explanation of the Dockerfile:
FROM openjdk:11-jre-slim
: We are using an official Java 11 runtime image. Using a slim version means we save space by not having unnecessary packages.WORKDIR /app
: This command sets the working directory to/app
inside the container, where our application code will reside.COPY . .
: This copies all files from the current directory into the/app
directory in the container.RUN javac HelloWorld.java
: This compiles our Java application.CMD ["java", "HelloWorld"]
: This specifies the command to run our application when the container starts.
Step 3: Build the Docker Image
Navigate to the directory containing your Dockerfile
and run the following command:
docker build -t my-java-app .
-t my-java-app
: This tags the image with a recognizable name.
Step 4: Run Your Docker Container
To run your Java application in a Docker container, execute the following command:
docker run --rm my-java-app
--rm
: This option automatically cleans up the container after it exits, keeping your environment tidy.
Output
If everything is set up correctly, you should see the output:
Hello, Docker!
Congratulations! You have successfully dockerized a simple Java application.
Advanced Configuration: Using Docker Compose
As your application grows, you might need to manage multiple containers. This is where Docker Compose shines. Let’s extend our example to include a web server.
Step 1: Create a Docker Compose File
Create a docker-compose.yml
file in the same directory:
version: '3'
services:
java-app:
build: .
ports:
- "8080:8080"
Explanation of the docker-compose.yml
:
version: '3'
: Defines the version of the Docker Compose file format.services
: This section defines the different services (containers) that your application will use. In this case, we have a single service namedjava-app
.build: .
: This tells Docker Compose to build the image using theDockerfile
in the current directory.ports
: This maps port 8080 of the container to port 8080 of your host, enabling you to access the application externally.
Step 2: Run with Docker Compose
To start the service, run:
docker-compose up
Docker Compose will build the image and start the Java application. If you navigate to http://localhost:8080
, you should see the response from your Java application.
Best Practices for Dockerizing Java Applications
To maximize the benefits of Docker in Java development, consider these best practices:
-
Multi-Stage Builds: Use multi-stage builds to keep your images smaller. Compile your code in one stage and only copy the necessary artifacts to the final image.
-
Use
.dockerignore
: Similar to.gitignore
, this file can specify which files and directories are not needed in the build context, helping speed up the build process. -
Environment Variables: Leverage Docker's ability to pass environment variables to your applications to keep your configuration clean and secure.
-
Regularly Update Images: Ensure you often rebuild your images with the latest versions of the base images to incorporate security improvements and patches.
-
Leverage Caching: Docker caches image layers. Organize your
Dockerfile
to take advantage of this feature and speed up your build process.
Key Takeaways
By leveraging Docker to containerize your Java applications, you can improve consistency, enhance isolation, and streamline deployment processes. Whether you are developing a simple "Hello World" app or scaling a multi-service Java enterprise application, Docker equips you with the tools needed to manage your applications effectively.
To learn more about enhancing local development with Docker, consider checking out the article "Ease Your Local Dev: Run Odoo in Docker Effortlessly" at infinitejs.com/posts/run-odoo-in-docker-effortlessly.
Now that you have the knowledge and tools at your disposal, it's time to dive into the world of Docker and explore how it can transform your Java development workflow. Happy coding!
Checkout our other articles