Optimizing Animation Performance in LibGDX
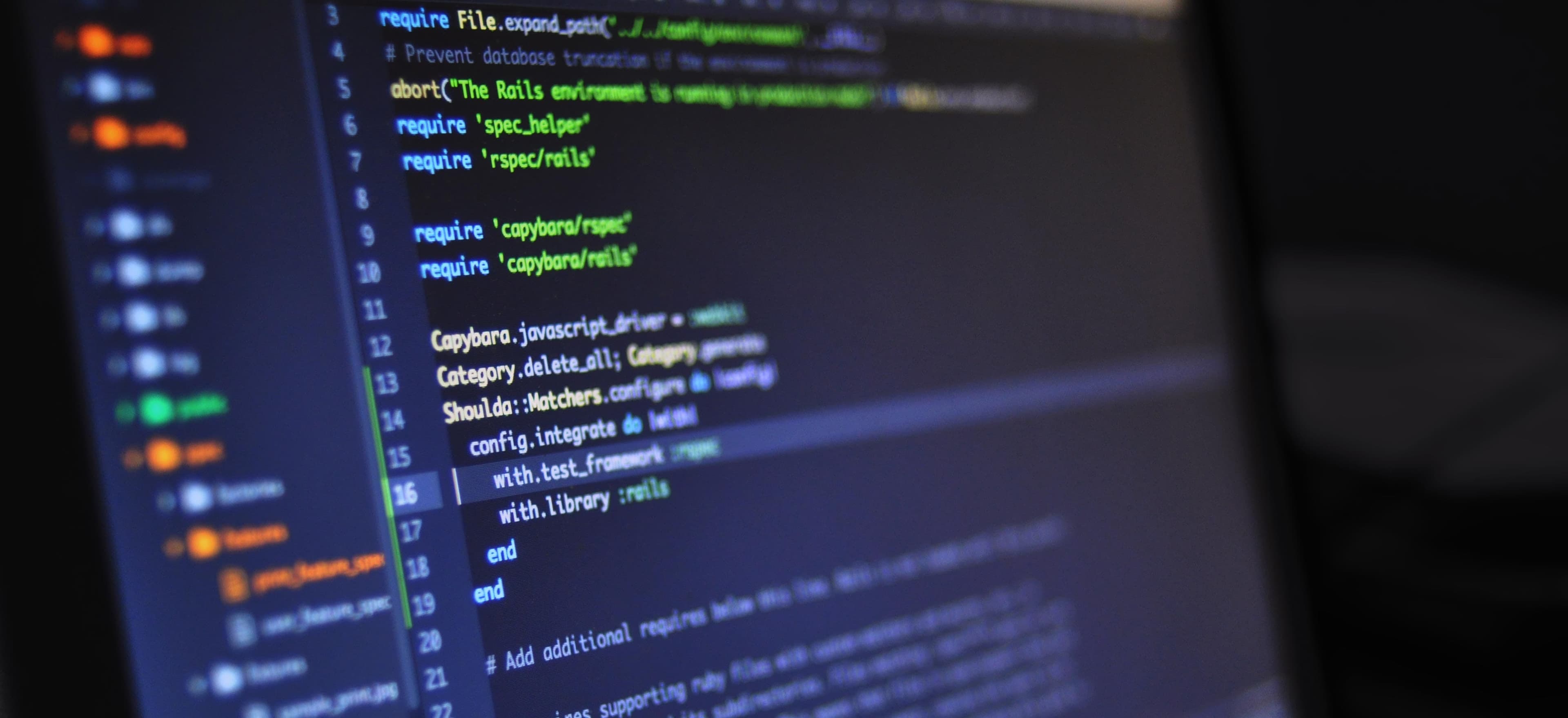
- Published on
Optimizing Animation Performance in LibGDX: A Complete Guide
LibGDX is a popular open-source game development framework for Java that provides a comprehensive set of tools and libraries for creating 2D and 3D games. One of the essential aspects of game development is animation, and ensuring optimal performance is crucial for delivering a smooth and immersive experience to the players. In this post, we will delve into the techniques and best practices for optimizing animation performance in LibGDX.
Understanding Animation in LibGDX
Before diving into optimization, let's have a quick overview of how animation works in LibGDX. The framework provides the Animation
class, which allows developers to create and manage sprite sheet-based animations. These animations involve cycling through a series of images at a specified frame rate, commonly used for character movements, visual effects, and more.
When working with animations, it's essential to keep an eye on performance to avoid potential issues such as stuttering, lag, or high resource consumption.
Use Texture Packing for Sprite Sheets
Sprite sheets are a commonly used technique to efficiently manage individual frames of an animation. However, using multiple individual image files for animation frames can lead to increased loading times and memory consumption. To address this, it's advisable to pack all the individual frames into a single sprite sheet using a texture packing tool like TexturePacker.
By doing so, you can reduce the number of texture switches and improve the texture cache efficiency, leading to better rendering performance and reduced memory overhead.
Here's a snippet illustrating the implementation of a packed sprite sheet with LibGDX:
TextureAtlas textureAtlas = new TextureAtlas(Gdx.files.internal("animations/packed_animation.atlas"));
Animation<TextureRegion> animation = new Animation<>(1 / 30f, textureAtlas.findRegions("animation_frames"), Animation.PlayMode.LOOP);
In this example, the TextureAtlas
is used to load the packed sprite sheet, and the Animation
is created using the regions from the atlas. By utilizing texture packing, you can streamline the rendering process and enhance animation performance.
Optimize Drawing Calls
Each frame of an animation involves drawing the corresponding image onto the screen. Excessive drawing calls can lead to performance bottlenecks, especially when dealing with a large number of animated entities simultaneously.
To mitigate this, it's crucial to optimize drawing calls by utilizing batch rendering. LibGDX provides the SpriteBatch
class, which efficiently handles the rendering of multiple sprites at once, reducing the overhead of individual draw calls.
Here's a simplified example demonstrating the use of SpriteBatch
for drawing animations:
SpriteBatch batch = new SpriteBatch();
batch.begin();
batch.draw(animation.getKeyFrame(elapsedTime), x, y);
batch.end();
By encapsulating multiple drawing operations within a single batch, you can significantly improve rendering performance, making it an essential practice for optimizing animation in LibGDX.
Implement Delta Time for Frame Rate Independence
Frame rate independence is a crucial aspect of animation performance that ensures consistent behavior across different devices and frame rates. When animations are tied directly to the frame rate, they can appear faster or slower based on the device's capabilities, leading to inconsistent experiences for the players.
To address this, implementing delta time (time elapsed since the last frame) for frame rate independence is essential. LibGDX provides a built-in mechanism to handle delta time, allowing animations to progress uniformly regardless of the frame rate.
Here's a code snippet showcasing the usage of delta time for frame rate independence:
float deltaTime = Gdx.graphics.getDeltaTime();
elapsedTime += deltaTime;
batch.begin();
batch.draw(animation.getKeyFrame(elapsedTime), x, y);
batch.end();
By incorporating delta time, you can ensure that animations behave consistently across various platforms and devices, enhancing the overall gaming experience.
Utilize Object Pooling for Memory Management
Managing memory allocation and deallocation is crucial for maintaining optimal performance, especially in the context of animations where entities might be frequently created and destroyed. Without proper memory management, excessive garbage collection can degrade performance and lead to frame rate drops.
To address this, leveraging object pooling for animation entities can significantly reduce memory churn and allocation overhead. Object pooling involves reusing pre-allocated objects instead of creating new instances, thereby minimizing the impact of garbage collection.
In LibGDX, the Pool
class can be utilized to implement object pooling for animation entities, ensuring efficient memory management and improved performance.
Pool<Sprite> spritePool = new Pool<Sprite>() {
@Override
protected Sprite newObject() {
return new Sprite();
}
};
Sprite sprite = spritePool.obtain();
// Use the sprite
spritePool.free(sprite);
By employing object pooling, you can mitigate memory-related performance issues and contribute to a smoother animation experience within your game.
The Last Word
Optimizing animation performance is a critical aspect of game development, and with LibGDX, developers have access to a rich set of tools and techniques to enhance rendering efficiency and deliver seamless animations. By employing texture packing, optimizing drawing calls, implementing delta time, and utilizing object pooling, developers can ensure that their animations run smoothly across a wide range of devices, providing players with an engaging and immersive gaming experience.
Incorporating these best practices into your LibGDX projects will not only elevate the visual appeal of your games but also contribute to overall performance optimization, paving the way for success in the competitive gaming industry.
Remember, understanding and implementing these optimization strategies is fundamental to achieving optimal animation performance in your LibGDX games, ultimately leading to a more enjoyable and compelling gaming experience for your audience.
Start leveraging these techniques today and take your game development skills to the next level with optimized animation performance in LibGDX!