Maximizing Feedback Loop Efficiency
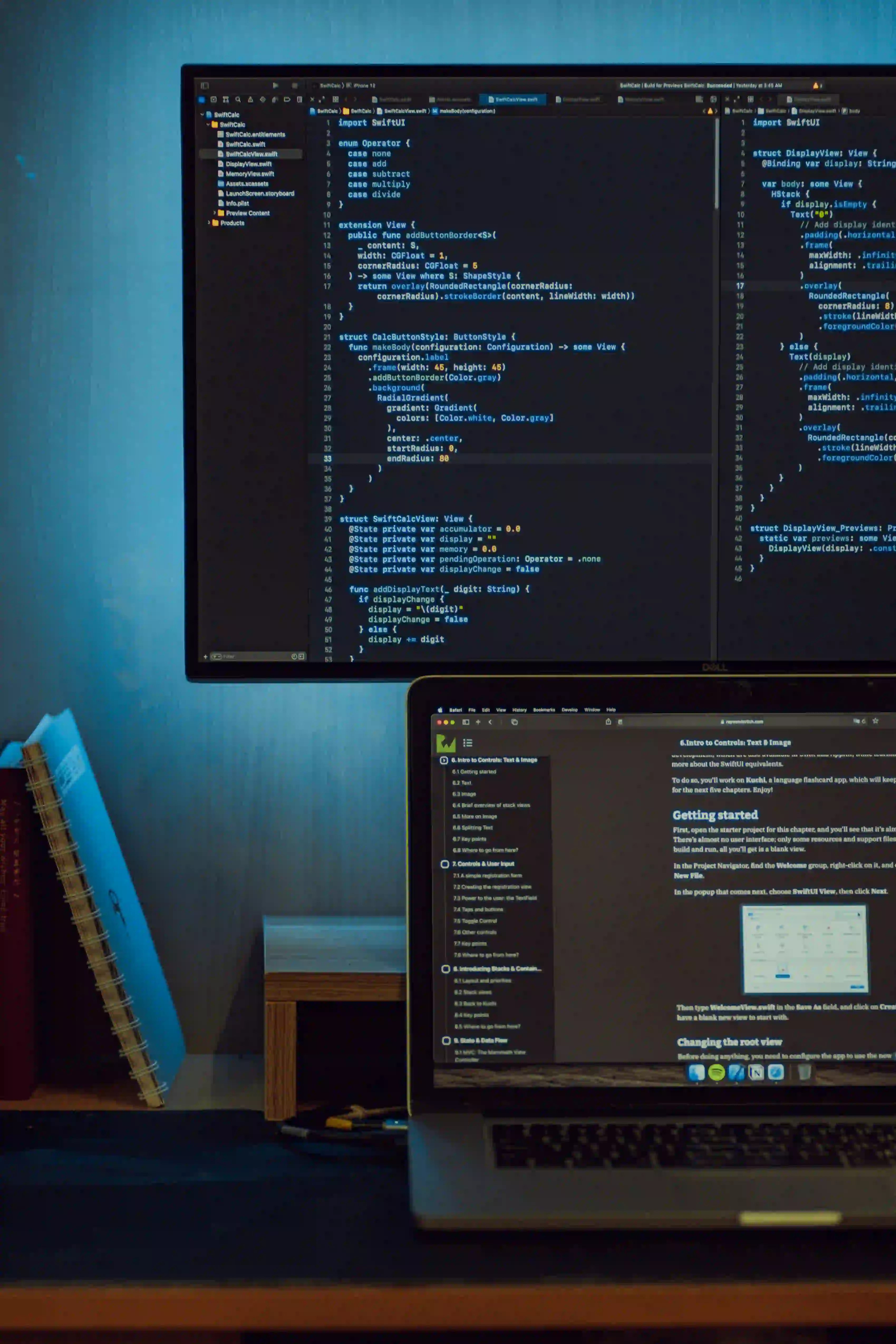
Maximizing Feedback Loop Efficiency in Java Development
In the realm of Java development, optimizing the feedback loop is crucial for enhancing productivity, code quality, and developer satisfaction. The feedback loop encompasses the process of receiving feedback on code changes, integrating this feedback, and iterating on the codebase. This iterative cycle forms the cornerstone of agile methodologies and continuous integration/delivery practices. In this blog post, we will delve into effective strategies for maximizing feedback loop efficiency in Java development.
Embracing Automated Testing
Automated testing plays a pivotal role in expediting the feedback loop. By employing unit tests, integration tests, and end-to-end tests, developers can promptly ascertain the impact of their code modifications. JUnit, a popular unit testing framework for Java, facilitates the creation and execution of unit tests, empowering developers to validate their code incrementally.
Consider a snippet of a JUnit test case for a simple Calculator
class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this snippet, the testAddition
method verifies the correctness of the add
method in the Calculator
class. When changes are made to the add
method, running this test promptly provides feedback regarding its behavior.
Continuous Integration and Continuous Delivery (CI/CD)
Integrating changes frequently and ensuring their seamless deployment are paramount to the feedback loop. CI/CD pipelines, orchestrated by tools like Jenkins, Travis CI, or GitLab CI/CD, automate the build, test, and deployment processes, enabling rapid feedback on code changes.
A simplified Jenkins pipeline's declarative syntax for a Java project might resemble the following:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'java -jar target/myapp.jar'
}
}
}
}
This pipeline automates the build using Maven, executes tests, and deploys the application. Feedback on the build and test outcomes is rapidly relayed to the developers, fostering a responsive feedback loop.
Version Control and Code Review Practices
Utilizing a robust version control system, such as Git, coupled with effective code review practices, is fundamental for feedback loop optimization. Platforms like GitHub offer pull requests, where peer reviewers can scrutinize code changes, provide feedback, and suggest improvements. Moreover, incorporating code review tools like CodeStream or Crucible streamlines the review process, thereby expediting feedback.
An illustrative snippet of a Git workflow entails creating a feature branch, making changes, committing them, and initiating a pull request:
git checkout -b feature/new-feature
# Make changes
git add .
git commit -m "Implement new feature"
git push origin feature/new-feature
The subsequent pull request triggers discussions, feedback, and iterative refinements, catalyzing the feedback loop.
Leveraging Static Code Analysis Tools
Integrating static code analysis tools, such as FindBugs, Checkstyle, or PMD, into the development workflow augments the feedback loop by flagging potential issues and enforcing coding standards. These tools offer insights into code quality and identify areas for improvement, furnishing developers with targeted feedback.
For instance, within a Maven project, integrating the FindBugs plugin entails configuring it in the pom.xml
as follows:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>findbugs-maven-plugin</artifactId>
<version>3.0.5</version>
<configuration>
<effort>Max</effort>
<threshold>Low</threshold>
</configuration>
</plugin>
</plugins>
</build>
Upon invoking the Maven build, FindBugs scrutinizes the codebase and generates feedback regarding potential bugs, inefficient code constructs, and more.
A Culture of Fast Feedback
Cultivating a culture that values fast feedback is indispensable for sustaining an efficient feedback loop. Encouraging prompt reviews, swift integration of feedback, and agile communication fosters a responsive development environment. Additionally, leveraging instant messaging platforms for quick clarifications and discussions can circumvent bottlenecks in the feedback dissemination process.
To Wrap Things Up
In the sphere of Java development, amplifying feedback loop efficiency is pivotal for fortifying development practices. Embracing automated testing, CI/CD pipelines, version control, code reviews, static code analysis, and a culture of prompt feedback are instrumental in honing the feedback loop. By prioritizing feedback loop efficiency, Java developers can iterate swiftly, deliver high-quality code, and foster a productive and gratifying development experience.
Incorporating these strategies into Java development workflows enables teams to respond adeptly to evolving requirements, rectify issues expediently, and engender a collaborative and agile development milieu. To explore more about the significance of feedback loops and agile methodologies, you can read Exploring the Agile Manifesto and The Importance of Feedback Loops in Software Development.
Remember, in Java development, the swifter the feedback, the smoother the journey towards exemplary software craftsmanship!