Configuring Timeout for Spring Integration Web Service Client
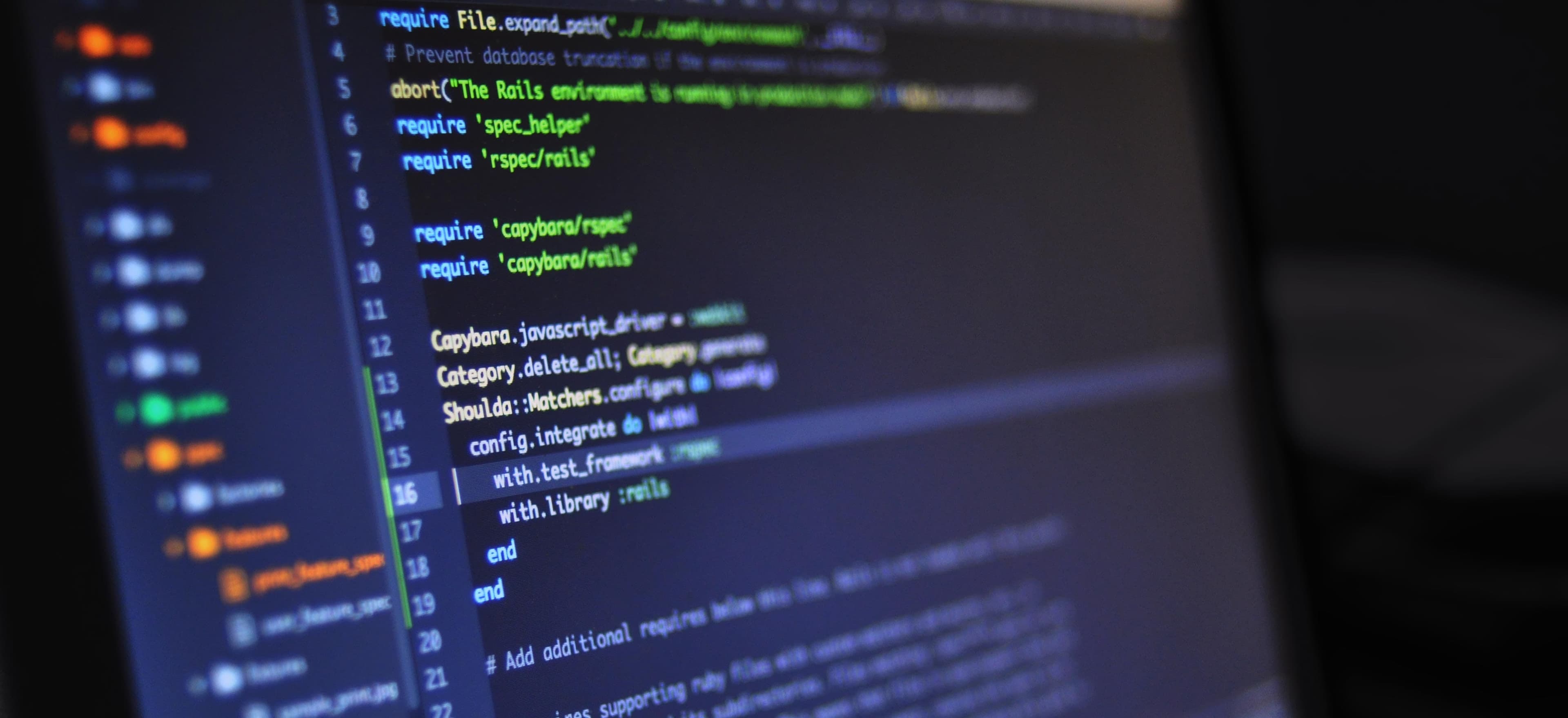
- Published on
Configuring Timeout for Spring Integration Web Service Client
When working with web service clients in a Java application, it is essential to handle timeouts effectively. Timeout configuration ensures that the application does not hang indefinitely when waiting for a response from the web service. In this blog post, we will explore how to configure timeouts for a Spring Integration web service client.
Understanding Timeout Configuration
Timeout configuration is crucial for web service clients to prevent long-running requests from impacting the overall performance of the application. When a client makes a request to a web service, it expects a response within a reasonable time frame. If the response takes too long to arrive, the client should time out and handle the situation accordingly.
In the context of Spring Integration, timeout configuration applies to the communication with a web service using the WebServiceMessageSender
interface. This interface provides the foundation for sending web service messages and allows us to set the connection and read timeouts.
Setting Timeout Values
To configure timeouts for a Spring Integration web service client, we need to set the connection and read timeouts for the underlying HTTP connection. Let's take a look at how we can achieve this.
Setting Connection Timeout
The connection timeout specifies the maximum time to establish a connection with the web service. If the connection cannot be established within this time frame, a java.net.SocketTimeoutException
is thrown.
import org.springframework.ws.transport.WebServiceMessageSender;
import org.springframework.ws.transport.http.HttpComponentsMessageSender;
WebServiceMessageSender messageSender = new HttpComponentsMessageSender();
messageSender.setConnectionTimeout(5000); // 5 seconds
In this example, we create an instance of HttpComponentsMessageSender
, which is one of the implementations of the WebServiceMessageSender
interface provided by Spring Integration. We then set the connection timeout to 5 seconds using the setConnectionTimeout
method.
Setting Read Timeout
The read timeout specifies the maximum time to wait for the response from the web service after the connection is established. If the response takes longer than this time frame, a java.net.SocketTimeoutException
is thrown.
messageSender.setReadTimeout(10000); // 10 seconds
Here, we set the read timeout to 10 seconds using the setReadTimeout
method. This ensures that the client waits for the response within a reasonable time frame.
Handling Timeouts
When a timeout occurs, it is essential to handle the situation gracefully. For example, we might want to log the timeout event, retry the request, or return a default response. In Spring Integration, we can use error handling and retry mechanisms to handle timeouts.
Error Handling
Spring Integration provides error handling capabilities through the ExpressionEvaluatingRequestHandlerAdvice
class. We can use this advice to handle timeout exceptions and perform custom actions, such as logging or triggering a fallback mechanism.
import org.springframework.integration.handler.advice.ExpressionEvaluatingRequestHandlerAdvice;
ExpressionEvaluatingRequestHandlerAdvice errorHandler = new ExpressionEvaluatingRequestHandlerAdvice();
errorHandler.setOnFailureExpressionString("payload + ' failed to process'");
errorHandler.setTrapException(true);
WebServiceOutboundGateway outboundGateway = new WebServiceOutboundGateway(messageSender);
outboundGateway.setAdviceChain(errorHandler);
In this example, we create an instance of ExpressionEvaluatingRequestHandlerAdvice
and configure it to handle failure expressions and trap exceptions. We then apply this advice to a WebServiceOutboundGateway
to handle timeout exceptions during the web service invocation.
Retry Mechanism
In addition to error handling, we can implement a retry mechanism to resend the request in case of a timeout. Spring Integration offers the RequestHandlerRetryAdvice
for this purpose.
import org.springframework.integration.handler.advice.RequestHandlerRetryAdvice;
RequestHandlerRetryAdvice retryAdvice = new RequestHandlerRetryAdvice();
retryAdvice.setRetryTemplate(retryTemplate);
WebServiceOutboundGateway outboundGateway = new WebServiceOutboundGateway(messageSender);
outboundGateway.setAdviceChain(retryAdvice);
Here, we create an instance of RequestHandlerRetryAdvice
and set a RetryTemplate
to define the retry behavior. We then apply this advice to a WebServiceOutboundGateway
to implement the retry mechanism for handling timeout scenarios.
Lessons Learned
Configuring timeouts for a Spring Integration web service client is essential for ensuring the responsiveness and resilience of the application. By setting connection and read timeouts, and implementing error handling and retry mechanisms, we can effectively manage timeout situations and provide a reliable web service client.
In this blog post, we discussed the significance of timeout configuration, demonstrated how to set timeout values for a web service client, and explored error handling and retry mechanisms to manage timeout scenarios effectively. By following these practices, Java developers can build robust web service clients that handle timeouts gracefully.
For further reading on Spring Integration and timeout configuration, refer to the official Spring Integration documentation. Additionally, exploring the official Java documentation can provide deeper insights into handling timeouts and network communication in Java applications.
Checkout our other articles