Mastering Java: Handling Custom Instructions in Solana
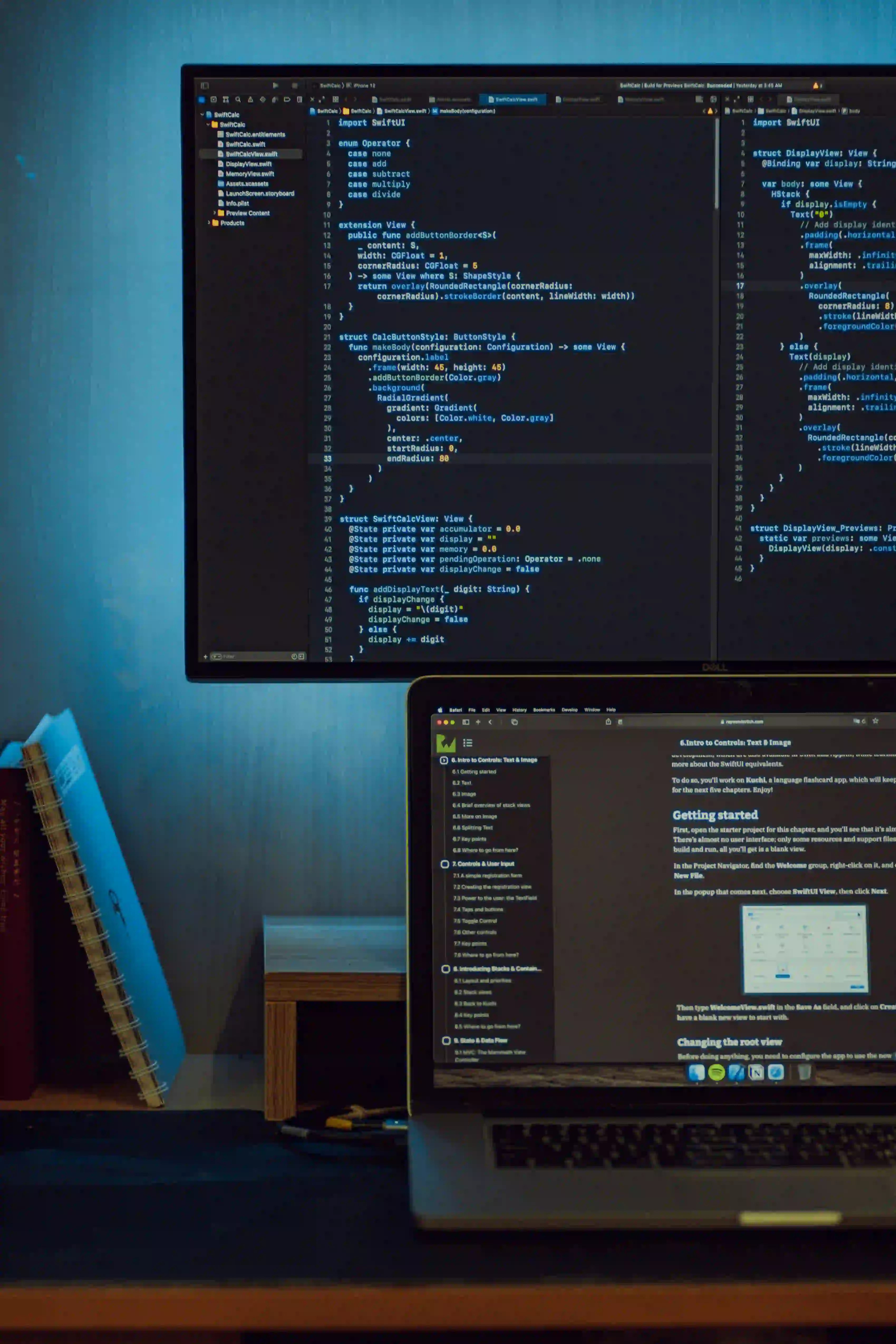
Mastering Java: Handling Custom Instructions in Solana
In the evolving world of blockchain technology, mastering programming languages to interact with various chains is essential. For instance, Java developers keen on Solana need to bridge their Java skill set with the abilities to send custom instructions using Solana instruction data. This blog post will provide detailed insights into handling custom instructions in Solana using Java, highlighting the relevant practices, necessary tools, and code snippets for a comprehensive understanding.
What Are Custom Instructions in Solana?
Custom instructions in Solana allow developers to define their own transaction logic beyond the standard operations. These transactions could include specific actions like transferring tokens, invoking smart contracts, or any custom logic your application needs. Understanding this functionality can greatly enhance the capabilities of your decentralized applications (dApps).
For a deeper dive into how custom instructions function within Solana, refer to the existing article titled Sending Custom Instructions Using Solana Instruction Data.
Prerequisites
To effectively work with Solana using Java, ensure you have the following prerequisites:
- Java Development Kit (JDK): It's essential to have JDK 8 or higher installed on your machine.
- Gradle or Maven: Dependency management tools are vital for integrating libraries.
- Solana SDK for Java: Unfortunately, there isn’t an official SDK as established as in JavaScript, but libraries like Solana Java can help.
- Basic understanding of blockchain concepts: Familiarity with wallets, transactions, and contracts is crucial.
Setting Up Your Java Project
Let’s begin by setting up a basic Java project that can help us interact with the Solana blockchain.
Step 1: Create Project Structure
Create a new directory for your project and navigate into it:
mkdir SolanaJavaProject
cd SolanaJavaProject
Step 2: Initialize Gradle Project
If you are using Gradle, create a new Gradle build file:
gradle init --type basic
Your project will now have a build.gradle file. Open it and add the following dependencies:
dependencies {
implementation 'org.web3j:core:4.8.7'
}
Step 3: Sync the Project
After setting up your dependencies, sync your Gradle project. This will allow you to use the necessary libraries required to communicate with the Solana network.
Understanding Transaction Structuring in Solana
Now that your project setup is complete, let's delve into handling custom instructions by discussing the core functionality required to create and send a transaction on the Solana blockchain.
Creating a Custom Transaction
The primary components of creating a transaction on Solana include:
- Sender's Wallet: The account that initiates the transaction.
- Recipient Address: The address that will receive the tokens or data.
- Transaction Instruction: The custom logic you want to apply during the transaction.
Here’s a basic Java function that constructs a simple transaction:
import org.web3j.protocol.Web3j;
import org.web3j.protocol.core.methods.response.EthSendTransaction;
import org.web3j.tx.gas.DefaultGasProvider;
public class SolanaTransaction {
private Web3j web3j;
public SolanaTransaction(Web3j web3j) {
this.web3j = web3j;
}
public String sendTransaction(String privateKey, String recipientAddress, String instructionData) {
// Load account from private key
Credentials credentials = WalletUtils.loadCredentials(privateKey);
// Build and send transaction
Transaction transaction = Transaction.createFunctionCallTransaction(
recipientAddress,
BigInteger.valueOf(21000),
DefaultGasProvider.GAS_PRICE,
instructionData);
EthSendTransaction response = web3j.ethSendTransaction(transaction).send();
return response.getTransactionHash();
}
}
Code Explanation
- Web3j: This library allows your application to interact with the Solana blockchain. It is a bridge between Java applications and the blockchain.
- Credentials: Load the sender's credentials from their private key to ensure that they can secure transactions.
- Transaction: This represents the transaction data that you'll send. It creates a function call to the recipient address with the instruction data.
In this section, we covered how to send a basic transaction. The design allows you to modify the transaction details as per your application's requirements.
Custom Instructions in Detail
Now, let’s explore what custom instruction data might look like and how you can extend your transaction.
Structure of Instruction Data
Custom instructions are usually represented as byte arrays. In Java, you can convert string data to bytes like this:
public byte[] createInstructionData(String customMessage) {
return customMessage.getBytes(StandardCharsets.UTF_8);
}
This simple function converts a string into bytes, enabling you to include a custom instruction message in your transaction.
Integrating Custom Logic
Suppose you want to implement a custom logic feature where the transaction also logs a message or performs additional validation. Here’s how you can extend the previous transaction class:
public String sendCustomInstruction(String privateKey, String recipientAddress, String customMessage) {
// Convert custom message to instruction data
byte[] instructionData = createInstructionData(customMessage);
// Send the transaction
return sendTransaction(privateKey, recipientAddress, instructionData);
}
Code Explanation
- Custom Instruction: In this snippet, you can see how to pass a custom message as a parameter, which is converted to byte format before the transaction is sent.
- Reusability: This approach builds upon existing functionality without needing to replicate code, demonstrating solid object-oriented principles.
Testing Your Application
Once you have implemented the transaction and custom instructions, testing is critical. If you're engaging with a live Solana network, consider using a test network (devnet) first to avoid unnecessary costs and complications.
Execute the Transaction
In your main application, creating a new instance of SolanaTransaction
, and invoking the sendCustomInstruction
method could look like this:
public static void main(String[] args) {
Web3j web3j = Web3j.build(new HttpService("https://api.devnet.solana.com"));
SolanaTransaction solanaTransaction = new SolanaTransaction(web3j);
String transactionHash = solanaTransaction.sendCustomInstruction("your_private_key", "recipient_address", "Your custom instruction");
System.out.println("Transaction Successful. Hash: " + transactionHash);
}
Important Considerations
- Network Choices: Use the devnet for testing; ensure your transactions work smoothly before deploying to the mainnet.
- Security: Make sure you never expose your private key openly; always keep it secure.
Final Thoughts
With this guide, you have equipped yourself to handle custom instructions on the Solana blockchain through Java programming. By setting up a Java project, creating reusable functions, and integrating custom logic, you can enhance your dApps' functionality effectively.
The world of blockchain is vast, and Solana’s unique characteristics provide Java developers an exciting frontier. Explore further, experiment with your implementations, and utilize the existing resources to deepen your understanding.
For more insights on Solana's custom functionalities, check out the article on Sending Custom Instructions Using Solana Instruction Data.
Happy coding!