Enhancing Java Applications with Solana's Custom Instructions
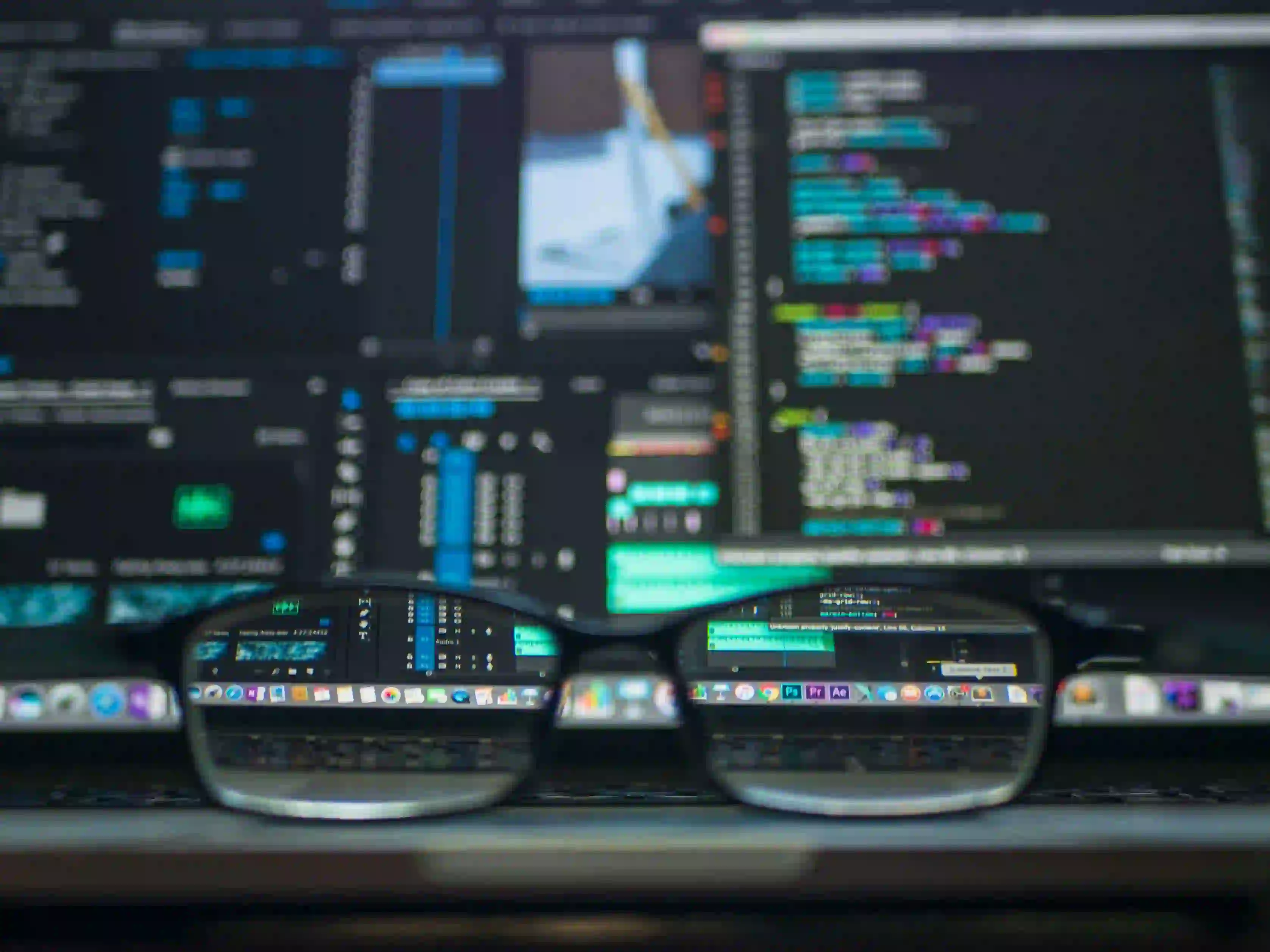
Enhancing Java Applications with Solana's Custom Instructions
In the world of blockchain technology, Solana has emerged as a robust contender, offering high throughput and low fees. As developers, it is crucial to explore how we can seamlessly integrate blockchain abilities into our applications. For Java developers, connecting Solana's functionalities, such as custom instructions, can elevate applications significantly. This article will delve deep into enhancing Java applications using Solana's custom instructions, offering both theoretical insights and practical code examples.
Understanding Custom Instructions
Custom instructions in Solana allow developers to define specific actions that the blockchain should perform when a transaction is executed. Essentially, they offer a way to extend Solana's default capabilities, enabling developers to process transactions tailored to their particular use cases. Whether it is executing smart contracts or facilitating unique token transactions, custom instructions bring flexibility to Solana's otherwise fixed architecture.
For a comprehensive understanding of custom instructions and how to send them on Solana, reference our previous article: Sending Custom Instructions Using Solana Instruction Data.
Setting Up Your Java Environment
Before diving into coding, ensure you have your Java development environment set up.
- Install Java JDK: Download and install the latest JDK from the official Oracle website.
- Maven or Gradle: Use either Maven or Gradle for dependency management. In this guide, we'll use Maven.
Adding Required Dependencies
In your pom.xml
, include the necessary dependencies for your project:
<dependencies>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
<!-- Add additional dependencies as necessary -->
</dependencies>
These dependencies allow your Java application to make HTTP requests and handle JSON data.
Creating a Basic Client to Interact with Solana
To communicate with the Solana blockchain, we will create a simple client that interacts with Solana's JSON RPC API.
Java Code Example for the Solana Client
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class SolanaClient {
private static final String SOLANA_API_URL = "https://api.mainnet-beta.solana.com";
public String sendTransaction(String jsonPayload) {
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpPost httpPost = new HttpPost(SOLANA_API_URL);
StringEntity entity = new StringEntity(jsonPayload);
httpPost.setEntity(entity);
httpPost.setHeader("Content-Type", "application/json");
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
BufferedReader reader = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuilder responseString = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
responseString.append(line);
}
return responseString.toString();
}
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
Code Commentary
- This class creates a simple HTTP client using Apache HttpComponents.
- A
sendTransaction
method is provided to send a JSON payload to Solana’s JSON RPC API. - The response is read from the InputStream and returned as a string.
Why Use This Approach?
An HTTP client like this enables Java applications to interact dynamically with the Solana blockchain. It abstracts the communication, allowing developers to focus on crafting custom instructions rather than worrying about the underlying API details.
Creating Custom Instructions
To create a custom instruction, we need to define the instruction type, specify the required accounts, and include any additional data.
Custom Instruction Example
Here’s how you can define a custom instruction in your Java code:
import org.json.JSONObject;
public class CustomInstruction {
public JSONObject createInstruction(String programId, String accountPubKey, String data) {
JSONObject instruction = new JSONObject();
instruction.put("programId", programId);
instruction.put("accounts", new String[]{accountPubKey});
instruction.put("data", data);
return instruction;
}
}
Code Commentary
- The
createInstruction
method generates a JSON object representing a custom instruction. programId
is the identifier of the deployed program on Solana that handles the instruction.accountPubKey
represents the account affected by the instruction.data
could contain any payload you wish to send.
Sending the Custom Instruction
Now that we have a basic foundation, let's combine our client and custom instruction to send a transaction to Solana.
public class SolanaTransaction {
public static void main(String[] args) {
SolanaClient solanaClient = new SolanaClient();
CustomInstruction customInstruction = new CustomInstruction();
String programId = "YourProgramIdHere";
String accountPubKey = "YourAccountPublicKeyHere";
String data = "YourInstructionDataHere"; // Sample data
JSONObject instruction = customInstruction.createInstruction(programId, accountPubKey, data);
String jsonPayload = new JSONObject()
.put("jsonrpc", "2.0")
.put("id", 1)
.put("method", "sendTransaction")
.put("params", new Object[]{instruction.toString()})
.toString();
String response = solanaClient.sendTransaction(jsonPayload);
System.out.println("Response from Solana: " + response);
}
}
Code Commentary
- The
main
method sets up the Solana client and creates a custom instruction. - The JSON-RPC payload is constructed, including the instruction.
- Finally, it sends the transaction using the Solana client and outputs the response.
Wrapping Up
Integrating Solana's custom instructions into your Java applications opens a world of possibilities. Learning to communicate with Solana's blockchain allows you to leverage decentralization and enhanced transaction capabilities. The above steps guide you in setting up your environment, creating client communication, and crafting specific instructions to interact deeply with the Solana ecosystem.
To fully explore the intricacies of custom instructions, further investigation into the documentation would be beneficial. As previously mentioned, consider reading the article on Sending Custom Instructions Using Solana Instruction Data for more nuanced insights.
With a solid understanding of these components, you can begin enhancing your applications, harnessing the speed and scalability of the Solana blockchain in your Java projects. Happy coding!